jwt.decode python example
jwt.decode python example
I'd be happy to provide an explanation of jwt
(JSON Web Token) decoding in Python!
What is JWT?
JWT is a widely used authentication token that allows secure transmission and validation of identity information between two parties - usually, a client (such as a web app or mobile app) and a server. It's a compact, URL-safe way to transmit information between systems.
In JWT, the payload (information) is signed with a secret key using an algorithm like HMACSHA256, making it tamper-proof and verifying the authenticity of the token.
What is jwt
in Python?
The jwt
library in Python provides functions for generating, verifying, and decoding JSON Web Tokens. It's part of the PyJWT project.
Here's a basic example of how to use jwt
to decode a JWT:
import jwt
Define a secret key (replace with your own!)
SECRET_KEY = 'your_secret_key_here'
Load the token from a file, for instance
with open('token.txt', 'r') as f:
token = f.read()
try:
Decode the token
decoded_token = jwt.decode(token, SECRET_KEY, algorithms=['HS256'])
print(f'Decoded Token: {decoded_token}')
except jwt.ExpiredSignatureError:
print("Token has expired!")
except jwt.InvalidTokenError:
print("Invalid token!")
In this example:
We import thejwt
library. Define a secret key (replace with your own!). Load the JWT from a file (e.g., token.txt
). Attempt to decode the token using the provided secret key and algorithm (HS256
, which stands for HMACSHA256). If successful, it will return the decoded payload. Catch and handle exceptions that may occur during the decoding process, such as an expired or invalid token.
Decoding a JWT: What's inside?
The decoded payload typically contains information about the user or entity associated with the token, such as:
exp
: The expiration date of the token. iat
: The issuance time (or issued-at time) of the token. sub
: The subject (user ID, username, etc.) of the token. aud
: The audience (target application or service) of the token.
The payload's structure and content depend on your specific use case. For instance, you might have additional claims like name
, email
, or groups
to represent user attributes.
Remember to store your secret key securely, as it's used to verify the authenticity of the token!
This is just a basic example; the jwt
library offers many more features and options for customizing and extending your JWT usage.
How to validate a JWT token in Python?
To validate a JSON Web Token (JWT) in Python, you can use the pyjwt
library, which is a popular and well-maintained package for handling JWTs. Here's an example of how to validate a JWT using pyjwt
:
First, install pyjwt
using pip:
pip install pyjwt
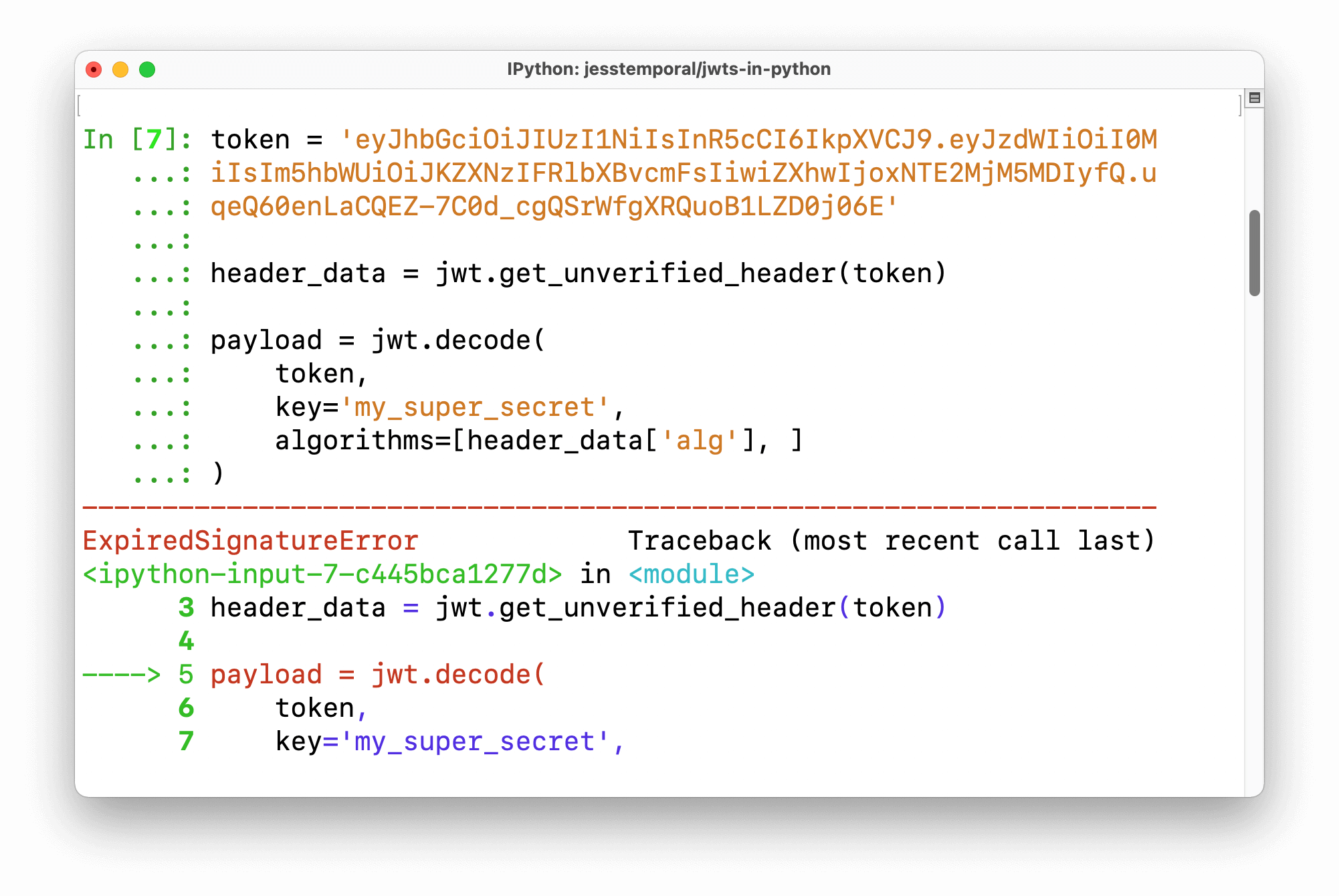
Next, import the PyJWT
class from pyjwt
and load your JWT token as a string:
from jwt import PyJWT
Load the JWT token as a string
token = "eyJhbGciOiJIUzI1NiIsInR5cCI6Ikb3dygb... (truncated for brevity)
Create an instance of PyJWT with a secret key
jwt = PyJWT(algorithm="HS256", key="your_secret_key_here")
Validate the JWT token
try:
decoded_token = jwt.decode(token)
print("Token is valid")
except Exception as e:
print(f"Invalid token: {e}")
In this example, we're using the HS256 algorithm, which is a popular choice for most use cases. You should replace "your_secret_key_here"
with your actual secret key.
The decode()
method takes the JWT token as input and returns a dictionary representing the decoded payload if the token is valid. If the token is invalid (e.g., expired, tampered, or invalid signature), it raises an exception.
You can also customize the validation process by providing additional options to the decode()
method. For example:
verify_exp=True
: Verifies that the token has not expired. verify_aud=None
: Specifies a list of allowed audiences (issuers) for the token. options={"verify_iat": True, "verify_nbf": False}
: Provides additional verification options.
Here's an updated example with some of these customization options:
decoded_token = jwt.decode(token,
verify_exp=True,
verify_aud=["your_issuer_here"],
options={"verify_iat": True, "verify_nbf": False})
By default, pyjwt
validates the token's signature using the provided secret key and algorithm. You can also use other validation options, such as verifying the token's not before (NBF) time or issuer (ISS).
In conclusion, validating a JWT token in Python using pyjwt
is straightforward. By providing your secret key and optional customization options, you can ensure that your tokens are securely verified and validated.
References:
PyJWT Documentation JSON Web Token (JWT) RFC 7519 HS256 Algorithm Specification