python pass by reference
python pass by reference
I'll respond in English.
Python is a dynamically-typed language, which means that it does not perform explicit memory management. However, when you assign an object to a new variable or pass an object as an argument to a function, Python doesn't actually create a copy of the original object. Instead, it creates a new reference to the same object.
In Python, everything is an object, including integers, floats, strings, lists, dictionaries, and even functions. When you assign one object to another variable or pass one object as an argument to a function, you are actually creating a new reference to the original object, not a copy of it.
Here's an example:
a = [1, 2, 3]
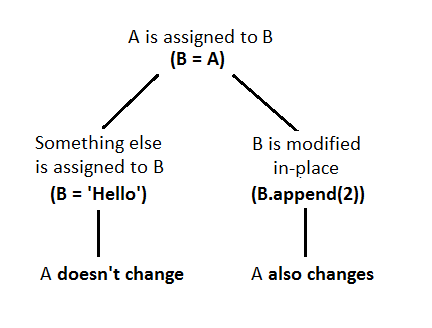
b = a
print(id(a), id(b)) # prints the same memory address for both variables
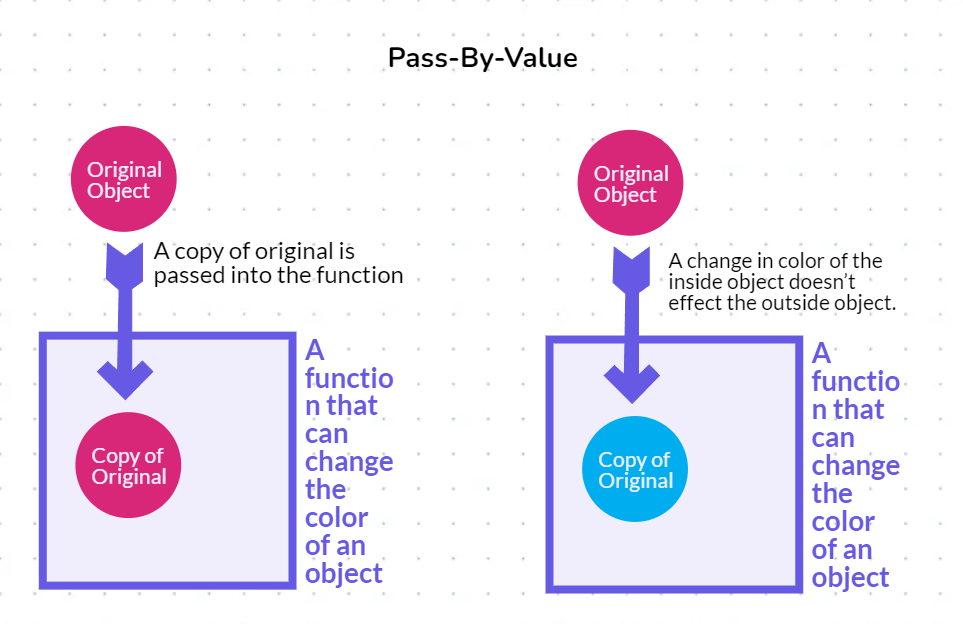
c = b
print(id(c)) # still prints the same memory address as a and b
In this example, a
, b
, and c
are all referencing the same list object. When you modify one of them, the change will be reflected in the others because they are actually pointing to the same memory location.
This is known as "call by sharing" or "pass-by-object-sharing". It's different from languages like C++, which use "call by value" (create a copy of the object) or "call by reference" (pass a pointer to the original object).
The implications of Python's pass-by-reference behavior are significant. For example, when you pass a list as an argument to a function and modify it inside the function, the changes will be reflected in the original list:
def modify_list(lst):
lst.append(4)
my_list = [1, 2, 3]
modify_list(my_list)
print(my_list) # prints [1, 2, 3, 4]
This is because lst
and my_list
are both referencing the same list object. When you modify the list inside the function, you're actually modifying the original list.
Understanding Python's pass-by-reference behavior can help you write more effective code and avoid unexpected side effects. It's an important aspect of Python programming that's worth keeping in mind!
how to reference variable in python
I'd be happy to help you with that!
In Python, referencing variables is a crucial concept to understand. When working with variables, you need to know how to access and manipulate their values. Here's a comprehensive guide on how to reference variables in Python:
Accessing Variables
To refer to a variable, you use the variable name followed by an assignment operator (=
) or an expression that evaluates to the desired value.
For example:
x = 5
print(x) # outputs: 5
In this example, x
is the variable being referred to. The print()
function accesses and prints its value.
Variable Names
Python variables can have any valid identifier as their name. A valid identifier consists of:
Letters (a-z, A-Z) Digits (0-9) Underscores (_)Here are some examples:
good_variable_name = "hello"
bad_variable_name = 5 # invalid, because it's a number
valid_identifier = "this_is_okay"
Assigning Values
To assign a value to a variable, use the assignment operator (=
). For example:
y = 3.14 # y is assigned the value 3.14
z = "hello" # z is assigned the string "hello"
In both cases, y
and z
are variables that now hold specific values.
Referencing Variables with Keywords
Python provides several keywords to reference variables:
print()
: Print the value of a variable.
Example:
x = 5
print(x) # outputs: 5
input()
: Read input from the user and store it in a variable.
Example:
name = input("What is your name? ") # stores user's name as a string
len()
: Get the length (number of items) in a variable, such as a list or string.
Example:
words = ["hello", "world"]
print(len(words)) # outputs: 2
type()
: Get the type of a variable (e.g., integer, string, etc.).
Example:
x = 5
print(type(x)) # outputs: <class 'int'>
Referencing Variables with Operators
Python's operators can be used to reference variables:
+
: Add the values of two variables.
Example:
x = 2
y = 3
result = x + y # result is assigned the value 5
-
: Subtract one variable from another.
Example:
x = 5
y = 2
result = x - y # result is assigned the value 3
And that's a wrap! In this guide, you've learned how to reference variables in Python using assignment operators, keywords like print()
and input()
, and operators for mathematical operations. Practice your newfound knowledge with exercises and projects, and soon you'll be well-versed in referencing variables in Python.