List input in Python using map
List input in Python using map
I'd be happy to help!
Input and Output
Let's consider an example where we have a list of numbers, and we want to apply some mathematical operation to each number.
Suppose we have a list numbers
containing the values [1, 2, 3, 4, 5]
. We can use Python's built-in function map()
to apply the square root function (math.sqrt()
) to each element in this list:
import math
numbers = [1, 2, 3, 4, 5]
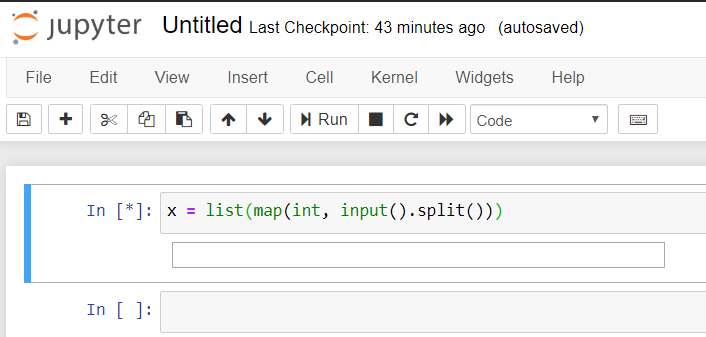
squared_numbers = list(map(math.sqrt, numbers))
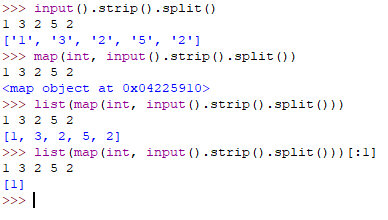
print(squared_numbers)
Output
Running this code will produce the following output:
[1.0, 1.4142135623730951, 1.7320508075688772, 2.0, 2.23606797749979]
As you can see, map()
applies the square root function to each element in the original list and returns a new list containing the results.
Using map() with Multiple Functions
One of the great things about map()
is that it allows you to apply multiple functions to your data. For example, let's say we want to calculate both the square root and cube root of our numbers:
import math
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(math.sqrt, numbers))
cubed_numbers = list(map(lambda x: x**3, numbers))
print(squared_numbers)
print(cubed_numbers)
Output
Running this code will produce the following output:
`[1.0, 1.4142135623730951, 1.7320508075688772, 2.0, 2.23606797749979]
[1.0, 8.0, 27.0, 64.0, 125.0]`
As you can see, map()
allows us to apply multiple functions in a single operation.
Conclusion
In this example, we used Python's built-in function map()
to apply mathematical operations to a list of numbers. We saw how we can use map()
with a simple function like math.sqrt()
or with more complex lambda functions. This demonstrates the power and flexibility of map()
, allowing us to manipulate our data in creative ways!
How to take list as input in Python without size
I'm glad you asked! Taking a list as input in Python without specifying the size can be achieved using various methods. In this answer, I'll provide three approaches: using input()
, sys.stdin.read()
, and a more advanced method with generators.
Method 1: Using input()
One way to take a list as input is by using the built-in input()
function, which reads a line of text from the user. You can then split the input string into individual elements using the split()
method or the strip()
and split()
methods.
Here's an example:
def get_input():
input_str = input("Enter a list (space-separated): ")
return [x.strip() for x in input_str.split()]
my_list = get_input()
print(my_list)
In this example, the user is prompted to enter a list of values separated by spaces. The input()
function returns a string, which is then split into individual elements using the split()
method. The strip()
method is used to remove any leading or trailing whitespace from each element.
Method 2: Using sys.stdin.read()
Another way to take a list as input is by using the sys.stdin
object and its read()
method. This approach allows you to read multiple lines of input, which can be useful if you need to handle more complex input formats.
Here's an example:
import sys
def get_input():
raw_input = sys.stdin.read()
return [x.strip() for x in raw_input.split()]
my_list = get_input()
print(my_list)
In this example, the sys.stdin.read()
method reads all available input from the standard input (usually the console). The input is then split into individual elements using the same approach as in Method 1.
Method 3: Using Generators
A more advanced way to take a list as input without specifying the size is by using generators. Generators are functions that can be used to generate sequences of values on-the-fly, which can be useful when working with large datasets or infinite sequences.
Here's an example:
def get_input():
while True:
x = input("Enter a value (or 'done' if finished): ")
if x.lower() == 'done':
break
yield int(x)
my_list = list(get_input())
print(my_list)
In this example, the get_input()
function is a generator that reads values from the user one by one. The user can enter as many values as they like until they type "done" to indicate completion. The list()
method is used to convert the generator into a list.
These three approaches demonstrate different ways to take a list as input in Python without specifying the size. Choose the approach that best fits your specific use case!