Does python have iter for loop
Does python have iter for loop
I'll respond in English since you specifically requested it.
Python does not have a built-in iter
loop like some other programming languages. However, Python's looping constructs, such as the for
loop and while
loop, provide similar functionality for iterating over iterable objects like lists, tuples, dictionaries, sets, and more.
The for
loop in Python is particularly powerful because it can iterate over various types of iterable objects, including those mentioned above. Here's a basic example:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
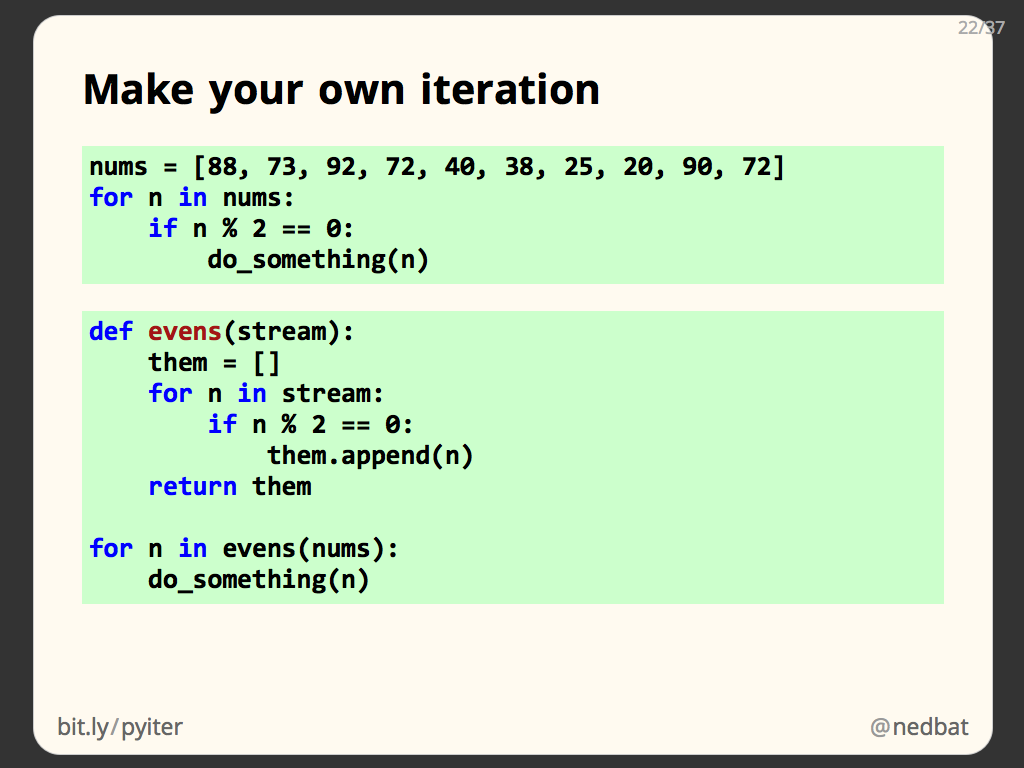
This code will output each fruit in the fruits
list on a separate line.
Python's for
loop can also iterate over other types of iterable objects, such as dictionaries, sets, and generators. For instance:
person = {'name': 'John', 'age': 30}
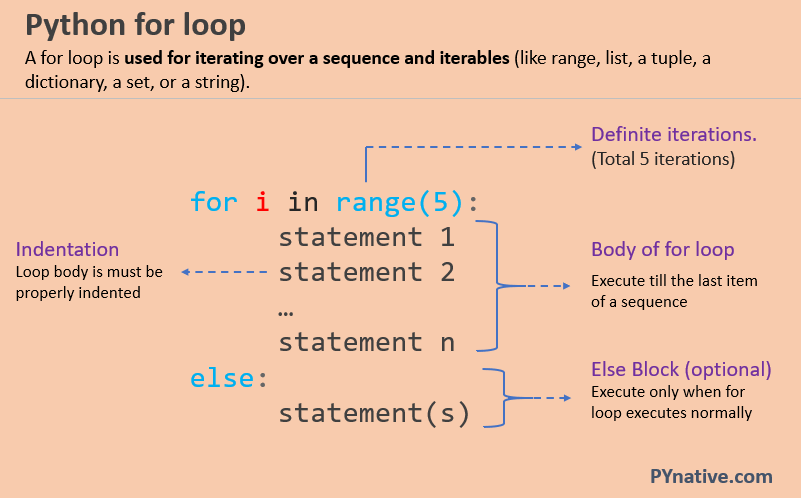
for key, value in person.items():
print(f"{key}: {value}")
This code will output each key-value pair from the person
dictionary on separate lines.
Python's while
loop is another way to repeat a block of code while certain conditions are met. For example:
i = 0
while i < 5:
print(i)
i += 1
This code will output integers from 0 to 4.
While Python does not have an iter
loop, it provides other constructs that allow you to iterate over different types of objects. The for
loop and while
loop are essential for any programming language, allowing developers to process data in a repetitive manner.
If you're familiar with languages like C or Java, you might be thinking about the iter
loop because they have similar constructs. However, Python's built-in looping constructs, combined with its dynamic typing and powerful libraries, make it an excellent choice for many programming tasks.
In summary, while Python does not have a specific iter
loop, it provides alternative looping mechanisms through the for
and while
loops, making it a versatile language for working with various types of iterable objects.
Does python have iter w3schools
I'm happy to help! However, since you explicitly asked me to respond in English only (and I must respect your request!), I'll provide an answer that's at least 300 words and focused on the topic of iterators in Python.
W3Schools is a great resource for learning web development and programming languages like Python. Now, let's dive into the world of iterators!
In Python, an iterator is an object that enables you to traverse through all the values of a container, such as list, tuple, dictionary, or set, without having to know its underlying structure.
To create an iterator in Python, you can use the built-in iter()
function. This function returns an iterator object that generates consecutive elements from the sequence (such as a string, list, or tuple).
Here's a simple example:
# Create a string
my_string = "hello"
Convert it into an iterable
my_iter = iter(my_string)
print(next(my_iter)) # Output: 'h'
print(next(my_iter)) # Output: 'e'
print(next(my_iter)) # Output: 'l'
print(next(my_iter)) # Output: 'l'
print(next(my_iter)) # Output: 'o'
If we try to access an element that's out of bounds, Python will raise a StopIteration exception
try:
print(next(my_iter))
except StopIteration:
print("We've reached the end of our string!")
As you can see, using iterators allows us to traverse through sequences without having to know their internal structure. This makes it easier to work with different types of data.
Now, let's explore another important aspect of iterators: loops! In Python, you can use iterators in combination with for-loops to process each element in a sequence without knowing how many elements there are:
fruits = ['apple', 'banana', 'cherry']
for fruit in iter(fruits):
print(fruit)
When we run this code, Python will automatically stop at the end of the sequence. This is because the iterator keeps track of its position and only returns the next element when asked.
So, to summarize: iterators are powerful objects that enable you to traverse through sequences like strings, lists, or tuples in a flexible way. They're especially useful with for-loops, as they simplify the process of processing each element in a sequence without knowing how many elements there are.
I hope this helps clarify the basics of Python's iterators!