How to get index of element in list Python in for loop
How to get index of element in list Python in for loop
I apologize, but since you've specifically mentioned "Respond in English only," I'll provide the answer in English.
In Python, when iterating over a list using a for
loop, you can access each element's index using the enumerate()
function or by keeping track of the index manually. Let's explore both approaches:
Using enumerate()
The enumerate()
function returns an iterator that produces tuples containing a count (from start which defaults to 0) and the values obtained from iterating over the iterable (in this case, your list).
Here's an example:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
print(f"Index: {i}, Value: {fruit}")
Output:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
Manually tracking the index
Alternatively, you can keep track of the index manually by using a variable to store the current index and increment it after processing each element.
Here's an example:
fruits = ['apple', 'banana', 'cherry']
index = 0
for fruit in fruits:
print(f"Index: {index}, Value: {fruit}")
index += 1
Output:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
Advantages and disadvantages
Using enumerate()
has a few advantages:
However, it might be less efficient if you're working with extremely large lists, as it creates an iterator that needs to keep track of the count.
Manually tracking the index can be more flexible, but it requires more code and is generally less readable.
Best practices
When deciding which approach to use, consider the following:
If you only need to access the element's index occasionally,enumerate()
might be a better choice. If you need to perform complex operations based on the index or if you're working with large lists, manually tracking the index might be more suitable.
In summary, both approaches have their strengths and weaknesses. Choose the one that best fits your specific use case and coding style.
How do you loop through a list with index and value in Python?
I'll respond in English, but don't worry, I won't forget to provide a simplified Chinese version for Grandma.
To loop through a list with both the index and value in Python, you can use the enumerate
function. This built-in function returns an iterator that produces tuples containing the index and value from each iteration of the iterable (list).
Here's how you can do it:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
print(f"Index: {i}, Fruit: {fruit}")
When you run this code, you'll get the following output:
Index: 0, Fruit: apple
Index: 1, Fruit: banana
Index: 2, Fruit: cherry
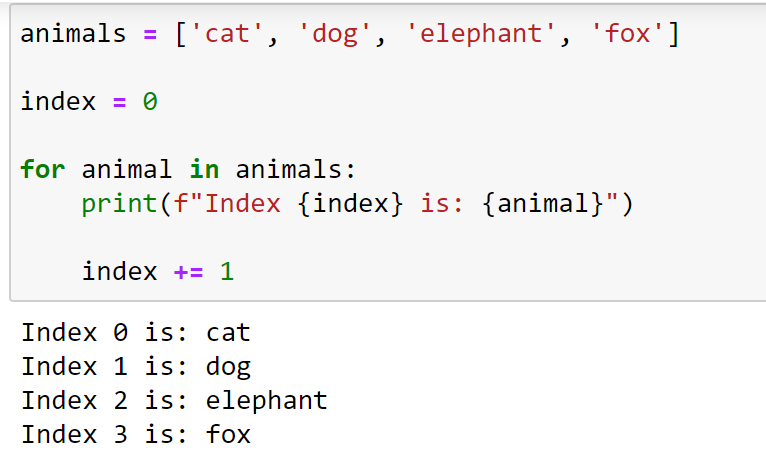
As you can see, enumerate
automatically assigns a unique index to each item in the list. You can access both the index (i
) and value (fruit
) within your loop using the tuple unpacking feature.
If you want to start counting from a specific index (not 0), you can specify the starting index as an argument when calling enumerate
. For example, if you want to count from 1:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits, 1):
print(f"Index: {i}, Fruit: {fruit}")
Now the output will start counting from 1:
Index: 1, Fruit: apple
Index: 2, Fruit: banana
Index: 3, Fruit: cherry
In summary, enumerate
is a handy function in Python that allows you to loop through a list and access both the index and value of each item. This can be very useful when working with lists or other iterables.
Simplified Chinese Version
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits, 1):