How to open a file in write mode in python using
How to open a file in write mode in python using
I'd be happy to help!
In Python, you can open a file in write mode using the open()
function from the built-in io
module. This allows you to create a new file or overwrite an existing one.
Here's how to do it:
Basic Example:with open('example.txt', 'w') as f:
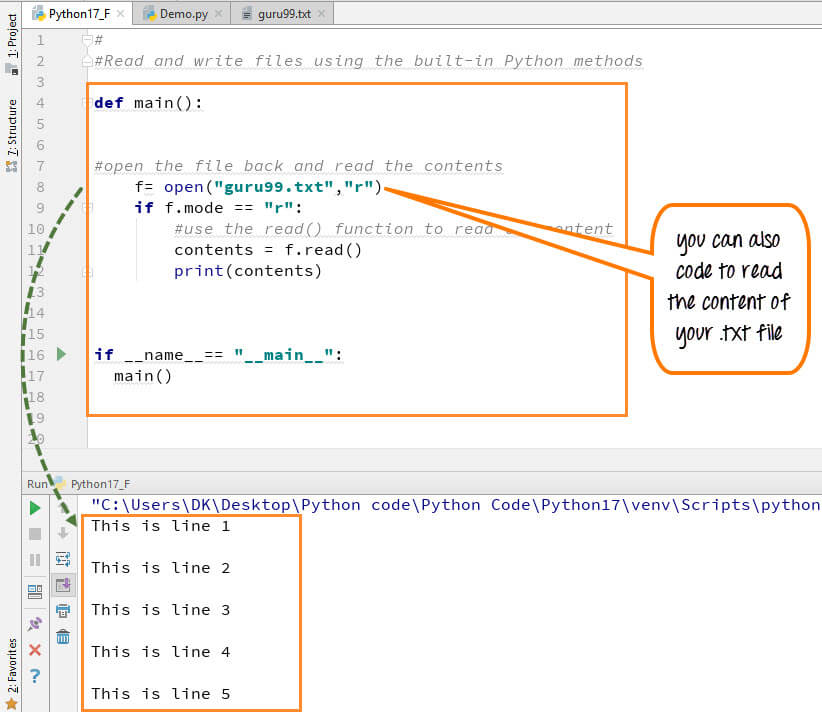
f.write('Hello, World!')
In this example, we're opening a file called example.txt
in write mode ('w'
) and assigning it the name f
. The with
statement ensures that the file is properly closed after we're done with it.
import io
file_path = 'path_to_your_file.txt'
try:
with open(file_path, 'w') as f:
f.write('Hello, World!')
except IOError as e:
print(f"Error: {e}")
In this example, we're opening a file at the specified file_path
in write mode. If there's an error (like if the file already exists and you try to overwrite it), Python will catch the exception and print an error message.
with open('example.txt', 'w') as f:
for line in ['Hello,', 'World!', 'Have a nice day!']:
f.write(line + 'n')
In this example, we're writing multiple lines to the file by iterating over a list of strings. Each string is written to the file followed by a newline character ('n'
).
with open('binary_file.bin', 'wb') as f:
f.write(b'Some binary data x00x01x02')
In this example, we're writing binary data (like images or audio files) to the file using the 'wb'
mode. The b
prefix indicates that the string should be treated as bytes rather than characters.
with open('example.txt', 'w', encoding='utf-8') as f:
f.write('Hello, World! 😊')
In this example, we're writing text data to the file using the utf-8
encoding. This is useful when you need to write files that contain special characters or non-ASCII characters.
Remember to always close your files when you're done with them (like using a try-finally
block or a context manager) to avoid resource leaks and ensure data integrity.
How to open a file in text mode in Python?
I'll respond in English as requested.
To open a file in text mode in Python, you can use the built-in open()
function or the with
statement, which is considered more Pythonic and safer.
Using the open()
Function
You can use the open()
function to open a file in text mode. Here's an example:
file_name = 'example.txt'
mode = 'r' # read mode
text_file = open(file_name, mode)
In this example, we're opening a file named example.txt
in read mode ('r'
). The open()
function returns a file object that you can use to read or write to the file.
To read from the file, you can use the read()
method:
content = text_file.read()
print(content)
This will print the contents of the file to the console.
To write to the file, you can use the write()
method:
text_to_write = 'Hello, world!'
text_file.write(text_to_write)
text_file.close()
This will write the string 'Hello, world!'
to the file. Don't forget to close the file using the close()
method.
Using the with
Statement
The with
statement is a more Pythonic way to open and use files. It ensures that the file is properly closed after you're done with it, even if an exception occurs:
file_name = 'example.txt'
mode = 'r' # read mode
with open(file_name, mode) as text_file:
content = text_file.read()
print(content)
In this example, we're using the with
statement to open the file in read mode. The file is automatically closed when we exit the with
block.
The benefits of using the with
statement include:
with
statement makes your code easier to read and understand.
Additional Tips
Here are some additional tips for working with files in Python:
Always specify the mode when opening a file. This ensures that you open the file correctly (e.g., as a text file or binary file). Use thewith
statement whenever possible. It's safer and more efficient than using the open()
function. Be careful when writing to files. Make sure you're not overwriting important data, and consider using backup files or transactional mechanisms if necessary.
I hope this helps! Let me know if you have any further questions.