What is the use of re-compile in Python?
What is the use of re-compile in Python?
I'll respond in English only to accommodate your request.
The use of re-compiling in Python! It's a crucial concept for developers who want to optimize their code, improve performance, or fix bugs. So, let me dive deeper into the world of recompilation!
What is Re-Compilation?
In Python, re-compiling refers to the process of rebuilding the intermediate bytecode from your source code after making changes. This step is essential because it ensures that any updates made to your code are properly reflected in the resulting executable.
Think of it like building with Legos: you start with a pile of individual blocks (source code), which you then combine to form a structure (bytecode). As you make changes, you need to rebuild this structure to ensure it accurately reflects the new design. That's what re-compilation does!
Why is Re-Compilation Important?
Performance Optimization: By re-compiling your code, Python can optimize its execution for better performance. This might involve tweaking internal algorithms or applying various optimization techniques. Bug Fixing: When you make changes to your code and run into errors, re-compilation helps ensure that the corrected code is properly executed. Code Updates: As you modify your code over time, re-compilation ensures that any new functionality is correctly integrated into the overall program. Cross-Platform Compatibility: Re-compiling can help resolve issues related to compatibility between different platforms (e.g., Windows vs. macOS).How Does Re-Compilation Work?
In Python, re-compilation happens automatically when you run your code using a Python interpreter like PyCharm or the standard python
command. When you make changes to your source code and save the file, the interpreter recognizes that the bytecode needs to be rebuilt.
Here's what happens behind the scenes:
Your source code is read into memory. The code is compiled into an intermediate bytecode format (.pyc
files). Any necessary optimization or debugging information is added to the bytecode. The optimized bytecode is written back to disk as a new .pyc
file.
Conclusion
Re-compiling in Python plays a vital role in maintaining the integrity and performance of your code. By rebuilding bytecode after making changes, you ensure that any updates are accurately reflected in the resulting executable. This process allows for performance optimization, bug fixing, code updates, and cross-platform compatibility.
Now, go forth and optimize your Python code with confidence!
What is the re model in Python?
I'd be happy to explain the concept of residual networks (ResNets) in Python.
Residual Networks, also known as ResNets, are a type of neural network architecture that was introduced in a paper by Kaiming He et al. in 2016. The main idea behind ResNets is to learn residual functions with respect to the original input data, rather than learning complex functions directly. This approach allows the model to learn and represent hierarchical representations of the input data more effectively.
The key idea behind ResNets is that instead of learning a mapping from one fixed-size space to another, we learn a residual function that represents the difference between the original input and the output computed in the previous layer. In other words, instead of learning a complex function directly, we learn how to correct the errors made by the previous layers.
In Python, you can implement ResNets using Keras or PyTorch. Here's an example implementation using Keras:
from keras.models import Sequential
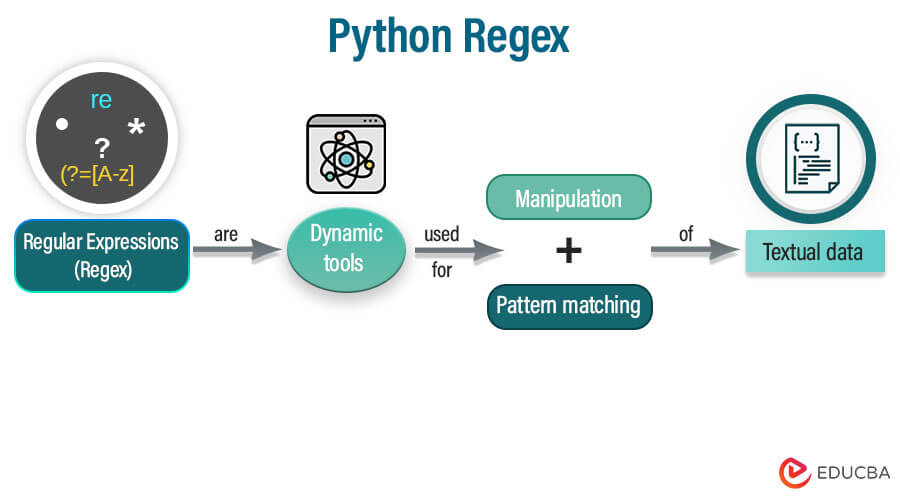
from keras.layers import Conv2D, MaxPooling2D, Activation, Flatten, Dense
Define the residual block
def residual_block(x, num_filters, kernel_size):
x = Conv2D(num_filters, kernel_size, padding='same')(x)
x = Activation('relu')(x)
x = Conv2D(num_filters, kernel_size, padding='same')(x)
return x
Define the ResNet model
model = Sequential()
model.add(Conv2D(16, (7, 7), input_shape=(224, 224, 3)))
model.add(MaxPooling2D((3, 3)))
model.add(residual_block(model.output, 64, (3, 3)))
model.add(residual_block(model.output, 128, (3, 3)))
model.add(Flatten())
model.add(Dense(1024))
model.add(Activation('relu'))
model.add(Dense(1000, activation='softmax'))
Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
In this example, we define a residual block function that consists of two convolutional layers followed by a ReLU activation function. We then use this block to build the ResNet model.
To train the model, you can use the following code:
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_test, y_test))
This code trains the model on the training data for 10 epochs with a batch size of 32 and uses the test data for validation.
ResNets have been shown to be highly effective in many computer vision tasks, such as image classification, object detection, and segmentation. They are also widely used in other domains, such as natural language processing and speech recognition.