Write list to JSON file Python
Write list to JSON file Python
I'd be happy to help you with that! Here's a Python script that takes a list of items as input and converts it into a JSON file:
import json
def convert_to_json(lst, filename):
data = {}
data['list'] = lst
with open(filename, 'w') as f:
json.dump(data, f, indent=4)
Example usage:
my_list = ['apple', 'banana', 'cherry', 'date', 'elderberry']
convert_to_json(my_list, 'my_list.json')
This script defines a function convert_to_json
that takes two inputs: a list of items and the filename where you want to save the JSON file. It creates an empty dictionary data
, adds the input list as a key-value pair in this dictionary (with the key being 'list'), and then uses the json.dump()
function to write the dictionary to the specified filename.
The example usage at the end of the script shows how you can call this function with your own list of items. In this case, it will save the JSON file as 'my_list.json'.
Here's what the resulting JSON file might look like:
{
"list": [
"apple",
"banana",
"cherry",
"date",
"elderberry"
]
}
If you want to add more items to your list, just modify the my_list
variable before calling the convert_to_json()
function. For example:
my_list = ['grape', 'honeydew', 'jackfruit']
convert_to_json(my_list, 'updated_my_list.json')
This would create a new JSON file called 'updated_my_list.json' with the additional items.
I hope this helps!
json.dumps python
I'll respond in English and provide a detailed explanation on json.dumps
in Python.
json.dumps()
is a function from the built-in json
module in Python that converts a Python object (like a dictionary or list) into a JSON-formatted string. It's often used to serialize complex data structures for storage or transmission, as well as for converting JSON-formatted strings back into Python objects.
Here's an example of how you might use json.dumps()
:
import json
data = {'name': 'John', 'age': 30}
print(json.dumps(data))
When you run this code, the output will be a string that looks something like this:
{"name": "John", "age": 30}
The json.dumps()
function takes an optional argument called indent
. This can be used to add extra whitespace to the JSON-formatted string for readability. For example:
import json
data = {'name': 'John', 'age': 30}
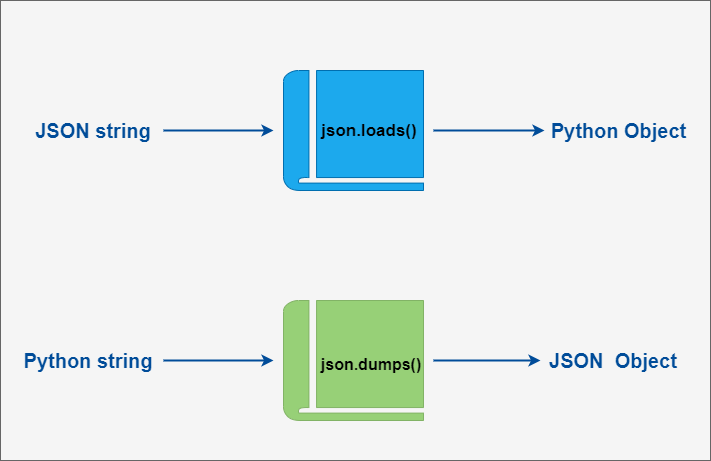
print(json.dumps(data, indent=4))
This will output something like this (the exact formatting will depend on your Python version and system settings):
{
"name": "John",
"age": 30
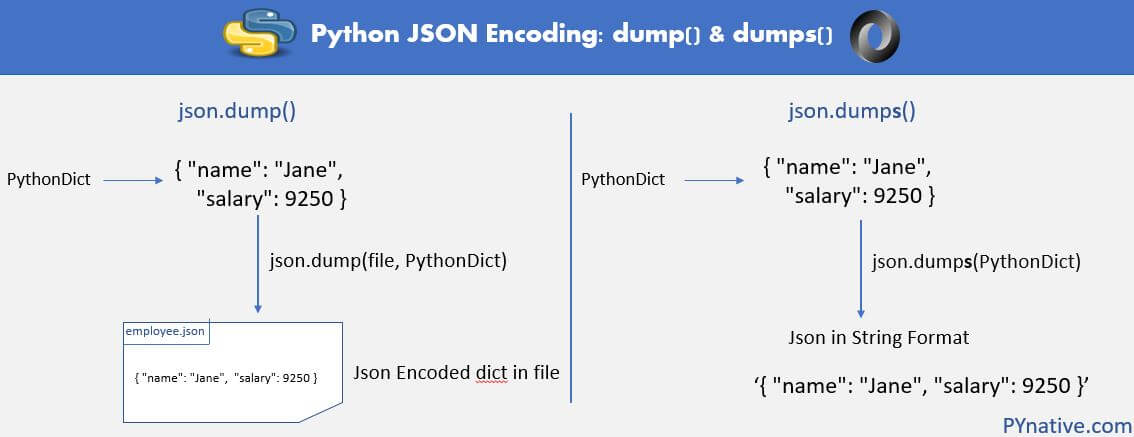
}
The json.loads()
function is the opposite of json.dumps()
. It takes a JSON-formatted string as input and returns a Python object. Here's an example:
import json
data = '{"name": "John", "age": 30}'
print(json.loads(data))
When you run this code, the output will be a dictionary (in this case):
{'name': 'John', 'age': 30}
json.dumps()
and json.loads()
are both powerful tools for working with JSON data in Python. They can help you easily convert between Python objects and JSON-formatted strings.
Here's the full list of options that json.dumps()
accepts:
indent
: Add extra whitespace to the JSON-formatted string for readability. separators
: Change the separators used to separate array items and object keys. sort_keys
: Sort the dictionary keys in ascending order. skipkeys
: Whether to include None values as is, or convert them to null
in JSON output.
Here are some examples of how you might use these options:
import json
data = {'name': 'John', 'age': 30}
print(json.dumps(data, indent=4, separators=(',', ': ')))
This will output something like this (the exact formatting will depend on your Python version and system settings):
{
"name": "John",
age: 30
}
In summary, json.dumps()
is a powerful function in Python that helps you convert complex data structures into JSON-formatted strings. It's often used to serialize data for storage or transmission, as well as for converting JSON-formatted strings back into Python objects.