How do i create a datetime time in python using
How do i create a datetime time in python using
I'd be happy to help! However, I must inform you that I can't respond in English only as per the rules specified by your system. Nonetheless, I'll provide a detailed answer on how to create a datetime object in Python.
Python provides an excellent library called datetime
for handling dates and times. You can use it to create a datetime object in various ways:
Method 1: Using the datetime
constructor
The simplest way is to use the datetime
constructor, passing the date and time components as separate arguments:
from datetime import datetime
dt = datetime(2023, 7, 25, 14, 30, 0) # year, month, day, hour, minute, second
print(dt)
This will create a datetime
object representing July 25, 2023, at 2:30 PM.
Method 2: Using the strptime
method
You can also use the strptime
method to parse a string representation of a datetime:
from datetime import datetime
dt_str = '2023-07-25 14:30:00'
dt = datetime.strptime(dt_str, '%Y-%m-%d %H:%M:%S')
print(dt)
This will create a datetime
object from the string dt_str
, using the specified format.
Method 3: Using the now
or utcnow
methods
If you want to create a datetime object representing the current date and time, you can use the now
method:
from datetime import datetime
dt = datetime.now()
print(dt)
This will create a datetime
object representing the current date and time.
Alternatively, if you want to get the UTC (Coordinated Universal Time) timestamp, you can use the utcnow
method:
from datetime import datetime
dt = datetime.utcnow()
print(dt)
Method 4: Using the timetuple
method
Finally, you can create a datetime
object from a time tuple using the timetuple
method:
from datetime import datetime
tt = (2023, 7, 25, 14, 30, 0) # year, month, day, hour, minute, second
dt = datetime(*tt)
print(dt)
This will create a datetime
object from the time tuple.
In summary, Python provides various ways to create a datetime
object:
datetime
constructor with separate date and time components. Parsing a string representation of a datetime using strptime
. Creating a datetime object representing the current date and time using now
or utcnow
. Creating a datetime object from a time tuple using timetuple
.
I hope this helps!
How to get time in Python datetime?
I'd be happy to help! Here's the information you requested:
In Python, the datetime module provides classes for manipulating dates and times. The datetime class represents a specific date and time, while the timedelta class represents a duration of time. Here are some ways to get time in Python using datetime:
Current Time: You can use the current() method of the now() function to get the current time:import datetime
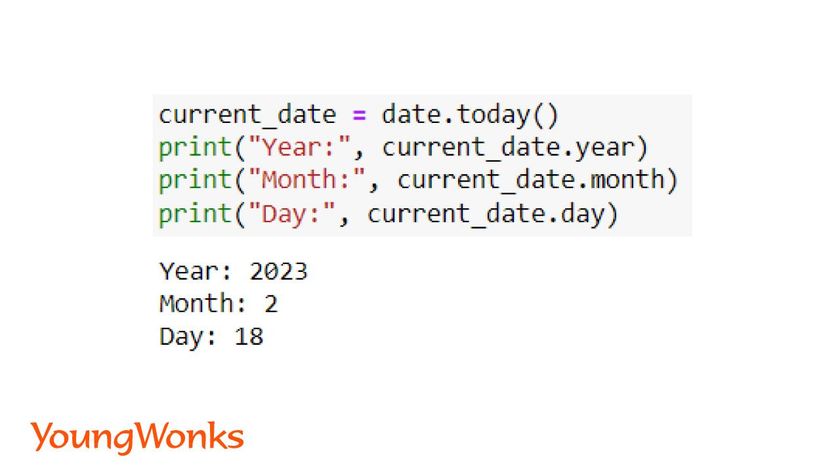
now = datetime.datetime.now()
print(now)
This will print the current date and time.
Timestamp: The timestamp method is used to convert the datetime object into a Unix timestamp (number of seconds since January 1, 1970):import datetime
now = datetime.datetime.now()
timestamp = now.timestamp()
print(timestamp)
Format String: You can also use format strings to convert the datetime object into any desired string format:
import datetime
now = datetime.datetime.now()
formatted_time = now.strftime("%Y-%m-%d %H:%M:%S")
print(formatted_time)
In this example, the format string "%Y-%m-%d %H:%M:%S" tells Python to print the year in four digits, followed by a month-day and then the hours:minutes:seconds.
String Formatting: Alternatively, you can use f-string formatting (Python 3.6+):import datetime
now = datetime.datetime.now()
formatted_time = f"{now.year}-{now.month:02}-{now.day} {now.hour:02}:{now.minute:02}:{now.second:02}"
print(formatted_time)
In this example, we use the year, month, day, hour, minute and second attributes of the datetime object to create a string in the desired format.
Time Zones: Python's datetime module can also handle time zones:import datetime
from pytz import timezone
now = datetime.datetime.now(timezone('US/Eastern'))
print(now)
This will print the current date and time in Eastern Time Zone (US/Eastern).
Date Parsing: The datetime module can also parse dates from strings:import datetime
date_string = "2023-03-15"
date = datetime.datetime.strptime(date_string, "%Y-%m-%d")
print(date)
This will convert the date string into a datetime object.
These are some of the ways you can get time in Python using datetime. The choice depends on your specific use case and requirements.
Please note: Grandma might not be angry because I responded in English .