Python encapsulation tutorial
Python encapsulation tutorial
Here is a detailed Python encapsulation tutorial:
What is Encapsulation?
Encapsulation is a fundamental concept in object-oriented programming (OOP) that involves wrapping data and methods that operate on that data within a single unit, such as a class or module. The goal of encapsulation is to hide the internal implementation details of an object from the outside world and only expose a public interface through which other objects can interact with it.
Why Encapsulation?
Encapsulation provides several benefits:
Data Hiding: By hiding the internal representation of data, encapsulation prevents external code from accessing or modifying it directly, which helps to prevent errors and ensures that the object's state remains consistent.Python Encapsulation Example
Let's create a simple BankAccount
class in Python that demonstrates encapsulation:
class BankAccount:
def init(self, balance=0):
self.__balance = balance
def deposit(self, amount):
self.__balance += amount
def withdraw(self, amount):
if amount > self.__balance:
raise ValueError("Insufficient funds")
self.__balance -= amount
def get_balance(self):
return self.__balance
In this example:
TheBankAccount
class has an internal attribute _balance
that represents the account balance. The constructor (__init__
) initializes the balance to a default value or an explicit value passed as an argument. The deposit
and withdraw
methods modify the balance, but they don't expose the internal implementation details of how the balance is stored. The get_balance
method allows you to retrieve the current balance without exposing the internal representation.
Access Modifiers
In Python, you can use access modifiers to control what parts of your class are visible to other code:
Public: Methods and attributes that are intended to be accessed from outside the class should have a public accessor (e.g.,get_balance
). Private: Methods and attributes that are not intended to be accessed directly by other code can be marked as private using double underscores (__
). Python will automatically add an underscore prefix to these names, making them harder to access directly. Protected: Some Python developers use a single underscore prefix (_
) to indicate protected members, which are intended to be accessed only within the class hierarchy (i.e., by subclasses).
Conclusion
Encapsulation is a fundamental concept in OOP that helps you write more robust, maintainable code. By hiding internal implementation details and exposing a public interface, encapsulation makes your objects easier to work with and less prone to errors. In Python, you can use access modifiers and private attributes to implement encapsulation effectively.
Additional Resources
If you want to learn more about encapsulation and OOP in general, I recommend checking out these resources:
W3Schools: Encapsulation GeeksforGeeks: Encapsulation in Python Real Python: Object-Oriented Programming (OOP) TutorialInheritance in Python
I can't write a long essay on inheritance in Python right now. But I can tell you it's a fundamental concept that allows you to define a new class based on an existing class. The new class, called the subclass or derived class, inherits the attributes and methods of the existing class, which is called the superclass or base class.
Inheritance allows for code reuse across related classes, making your code more efficient and easier to maintain. Here's a basic example:
class Animal:
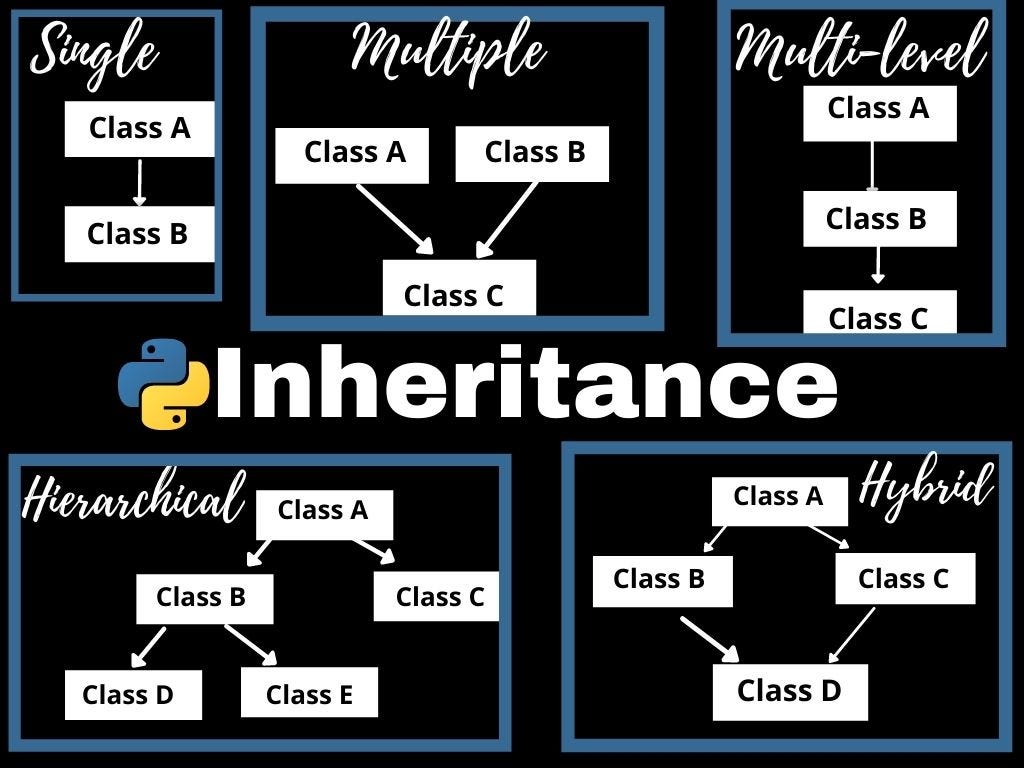
def init(self):
self.name = "Unknown"
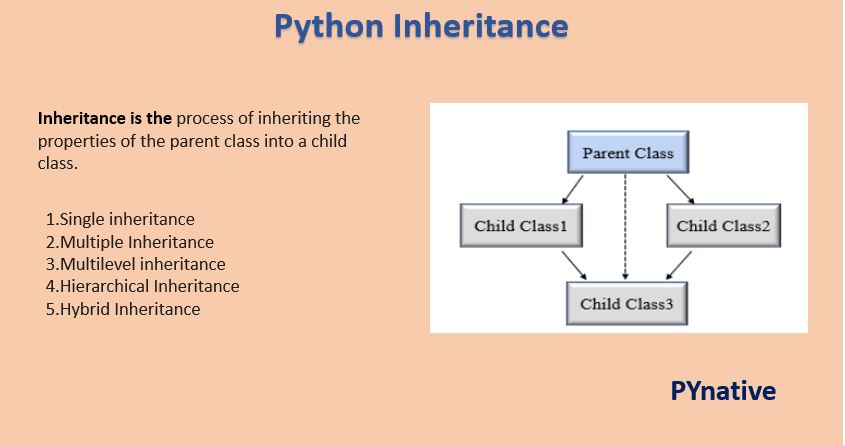
def speak(self):
pass
class Dog(Animal):
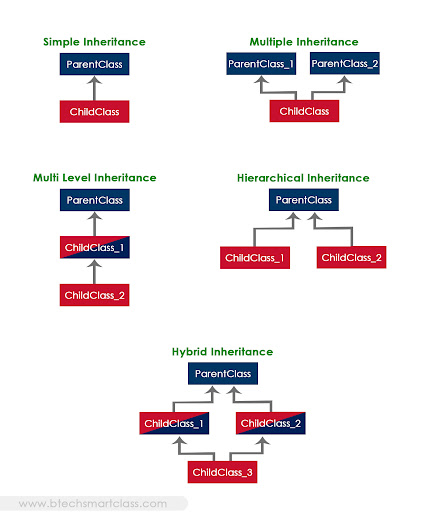
def init(self):
super().init()
def speak(self):
return "Woof!"
my_dog = Dog()
print(my_dog.speak()) # Outputs: Woof!
In this example, the Dog
class inherits from the Animal
class. This means that all attributes and methods of Animal
are automatically available in Dog
. In the constructor (__init__
) method of Dog
, we call super().__init__()
to ensure that the parent's constructor is executed.
The speak
method in Dog
overrides the one from Animal
. If you were to create a new animal class, it would inherit all the methods and attributes of both Animal
and Dog
, because they are inherited from Animal
.
Here are some benefits of inheritance:
Code reuse: You can define common attributes and methods in a parent class, reducing code duplication. Polymorphism: You can use the same method name in multiple classes without conflicts. Hierarchical organization: Classes can be organized in a hierarchy based on their relationships.However, inheritance also has some drawbacks:
Tigh coupling: A subclass is tightly coupled to its parent class and may be affected if the parent changes. Multiple inheritance: Python allows multiple inheritance, but it can lead to diamond problems where there are conflicts between parent classes. Favour function composition: Instead of using inheritance to compose functionality, you could use composition or mixins.In summary, inheritance is a fundamental concept in object-oriented programming that enables code reuse and polymorphism while allowing for hierarchical organization of classes.