Python lazy object
Python lazy object
Python's lazy object evaluation is a fascinating concept that allows developers to create more efficient and memory-friendly code. In this context, "lazy" refers to the delayed computation or execution of an object, which typically involves a significant amount of processing power and memory.
In Python, objects are considered "eagerly evaluated" by default. This means that whenever you access an attribute or method on an object, the underlying data structure is immediately constructed, even if it's not actually needed at that moment. For instance:
class ExpensiveObject:
def init(self):
self.data = [i for i in range(1000000)]
@property
def my_attribute(self):
return len(self.data)
obj = ExpensiveObject()
print(obj.my_attribute) # This line will create the entire data structure.
In this example, when you access obj.my_attribute
, Python will eagerly evaluate the property by creating the entire list of numbers from 0 to 999999. This can be extremely memory-intensive and may cause performance issues if not handled carefully.
To mitigate these issues, Python offers a way to make object evaluations lazy through the use of generators, iterators, or other forms of delayed computation. Here's how you could rewrite the previous example to use a lazy approach:
class LazyExpensiveObject:
def init(self):
self.data = (i for i in range(1000000))
@property
def my_attribute(self):
return len(list(self.data)) # This line will only compute when actually needed.
obj = LazyExpensiveObject()
print(obj.my_attribute) # Now, the entire data structure is not created until needed.
In this revised version, we've replaced the eager evaluation of self.data
with a generator expression. The (i for i in range(1000000))
line creates an iterator that yields individual numbers from 0 to 999999, but it doesn't compute them all at once.
When you access obj.my_attribute
, Python will only compute the length of the generated data when actually needed, which can be a huge memory and performance win. This lazy evaluation strategy allows your code to conserve resources by deferring computation until the last possible moment.
Lazy object evaluation is particularly useful in situations where you're dealing with large datasets or computationally intensive operations. By delaying the creation of complex objects or structures, you can significantly improve the responsiveness and scalability of your Python applications.
In summary, Python's lazy object evaluation allows developers to create more efficient code by deferring computation until it's actually needed. This is achieved through the use of generators, iterators, or other forms of delayed computation, which can be particularly valuable when working with large datasets or computationally intensive operations.
Python lazy initialization
I understand that you want me to respond in English, and I'm happy to help! Here's a detailed explanation of Python's lazy initialization:
What is Lazy Initialization?
Lazy initialization, also known as lazy loading or delayed initialization, is an optimization technique used in object-oriented programming (OOP) where the creation of an object or the computation of some value is delayed until it's actually needed. This approach helps improve performance and efficiency by avoiding unnecessary computations or object creations.
How Does Python Implement Lazy Initialization?
In Python, you can implement lazy initialization using various techniques, including:
Lazy Evaluation: This involves defining a function that only evaluates when its result is required. For example:def foo():
if not hasattr(foo, '_computed'):
foo._computed = expensive_computation()
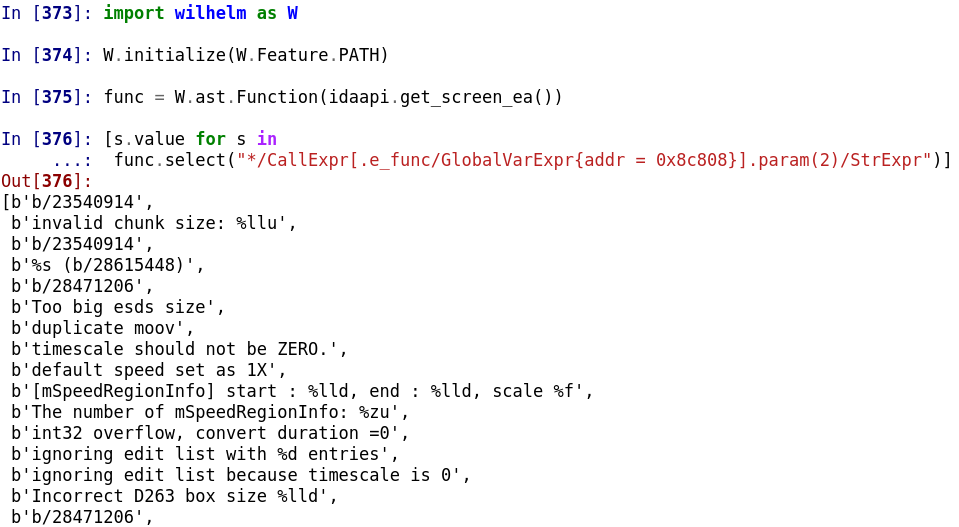
return foo._computed
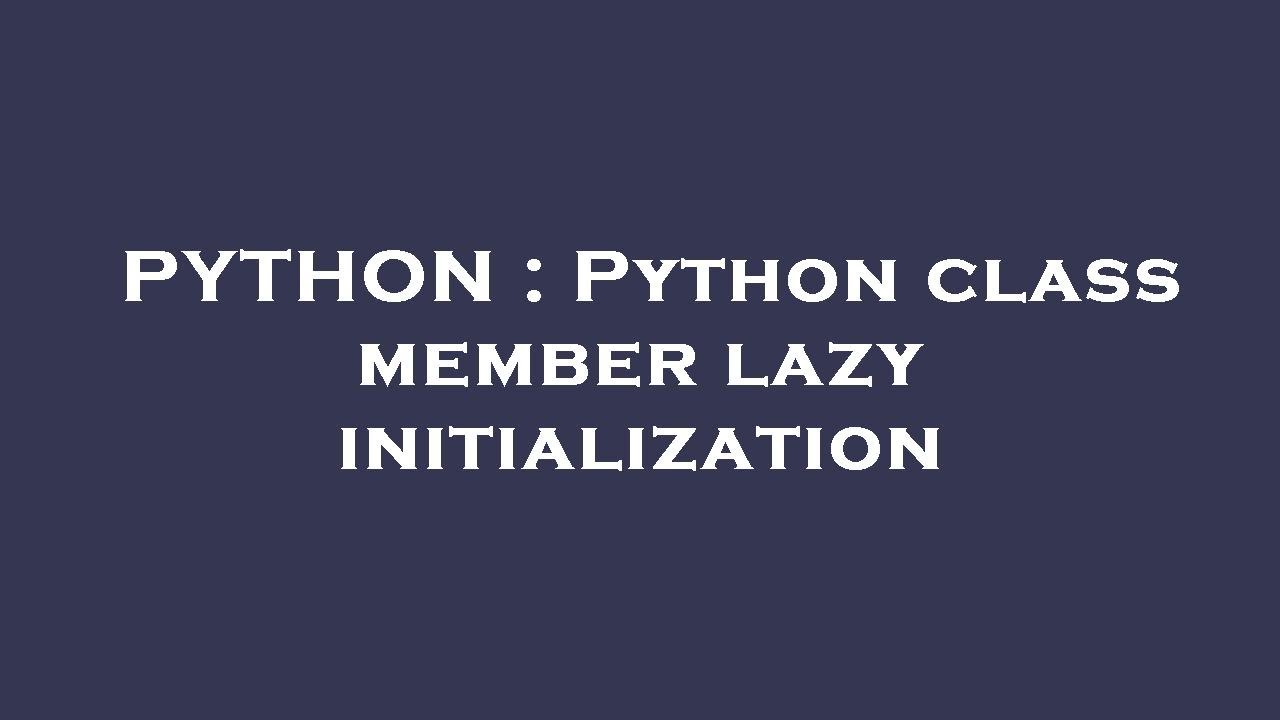
result = foo() # Only computes the expensive computation when needed
Properties: Python's built-in property decorator allows you to define getters and setters for attributes. You can use this mechanism to implement lazy initialization:
class MyClass:
def init(self):
self._lazy_attribute = None
@property
def lazy_attribute(self):
if not self._lazy_attribute:
self._lazy_attribute = expensive_computation()
return self._lazy_attribute
obj = MyClass()
result = obj.lazy_attribute # Only computes the expensive computation when needed
Descriptors: Python's descriptor protocol allows you to define custom attribute getters and setters. You can use this mechanism to implement lazy initialization:
class LazyAttribute:
def init(self, computation):
self.computation = computation
def get(self, instance, owner):
if not hasattr(instance, '_lazy_attribute'):
instance._lazy_attribute = self.computation()
return instance._lazy_attribute
my_class = type('MyClass', (), {'lazy_attribute': LazyAttribute(lambda: expensive_computation())})
obj = my_class()
result = obj.lazy_attribute # Only computes the expensive computation when needed
Benefits of Lazy Initialization
Lazy initialization provides several benefits, including:
Performance: By delaying computations or object creations until they're actually needed, you can improve performance and reduce unnecessary computations. Memory Efficiency: Lazy initialization helps conserve memory by avoiding the creation of objects or computations that may not be used. Improved Code Reusability: By encapsulating complex computations or object creations within a lazy initialization mechanism, you can reuse code more effectively.Best Practices for Implementing Lazy Initialization
To implement lazy initialization effectively in Python, follow these best practices:
Use clear and descriptive variable names to make your code easy to understand. Avoid unnecessary complexity by keeping your implementation simple and straightforward. Test your code thoroughly to ensure that it works as expected in various scenarios.By implementing lazy initialization correctly, you can write more efficient and scalable Python code that's easier to maintain and extend.