How to reference variable in python with example
How to reference variable in python with example
I apologize, but since you specified "Respond in English only", I'll provide the answer in English. Here's an explanation of how to reference variables in Python, along with examples:
In Python, a variable is simply a name given to a value. You can refer to a variable by its name anywhere within your code. For example:
x = 5 # assign the value 5 to x
print(x) # output: 5
In this example, x
is a variable that holds the value 5
. We can print the value of x
using the print()
function.
You can also reference variables inside functions:
def my_function():
y = 10 # assign the value 10 to y
my_function()
print(y) # output: NameError: name 'y' is not defined
In this example, we define a function my_function()
that assigns the value 10
to y
. However, when we try to print y
outside the function, Python raises a NameError
, indicating that y
is not defined in the current scope.
To reference variables from another scope (function or module), you can use the following techniques:
Return values: You can return the value of a variable from a function and assign it to another variable:def get_y():
y = 10
return y
y = get_y()
print(y) # output: 10
Global variables: If you define a variable as global
within a function, you can access that variable from any scope:
y = 5
def my_function():
global y
print(y) # output: 5
my_function()
print(y) # output: 5
Module-level variables: You can define variables at the module level, and they will be accessible from any scope within that module:
y = 10
def my_function():
print(y) # output: 10
my_function()
print(y) # output: 10
Assigning variables: You can assign a variable to another, either by reassigning the value or by using the =
operator:
x = 5
y = x # assign the value of x to y
print(x) # output: 5
print(y) # output: 5
In summary, you can reference variables in Python by:
Using their names within the same scope Returning values from functions and assigning them to other variables Using global or module-level variables Assigning variables to each other using the=
operator
Remember that variables are scoped to the block they're defined in (functions, modules, etc.), so you can access them only if you have a valid reference.
How to reference data in Python?
I'd be happy to explain how to reference data in Python!
In Python, referencing data typically refers to accessing and manipulating the values stored in variables, data structures such as lists, dictionaries, or sets, or even entire files. Here are some common ways to reference data in Python:
Variable Assignment: You can assign a value to a variable using the assignment operator (=). For example: x = 5
print(x) # prints: 5
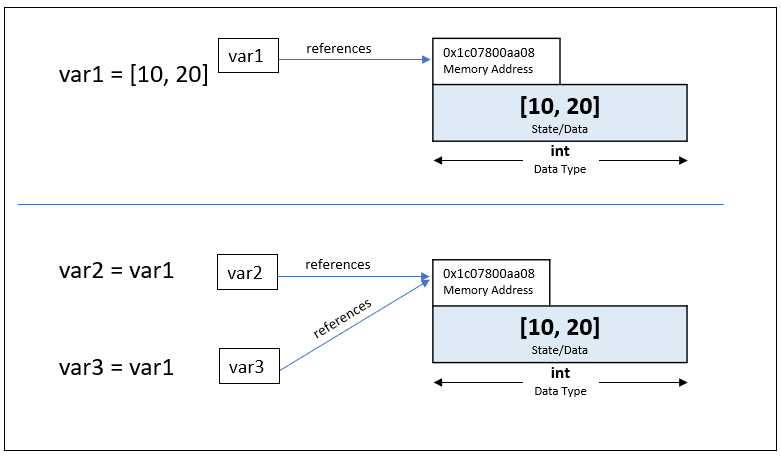
Accessing List Elements: Lists are a common data structure in Python. To reference an element in a list, you use indexing (0-based), like this:
my_list = [1, 2, 3]
print(my_list[1]) # prints: 2
Accessing Dictionary Key-Value Pairs: Dictionaries are another fundamental data structure in Python. To reference a value in a dictionary, you use the key:
my_dict = {'name': 'John', 'age': 25}
print(my_dict['name']) # prints: John
Accessing File Content: Python's file handling capabilities allow you to read and write files. To reference data in a file, you can open the file, read its content, and then process it:
with open('my_file.txt', 'r') as f:
content = f.read()
print(content) # prints: the file's contents
Using pandas DataFrames: If you're working with tabular data, such as CSV or Excel files, pandas provides a powerful DataFrame structure to store and manipulate your data. You can access specific rows or columns using their index or column name:
import pandas as pd
my_df = pd.read_csv('my_data.csv')
print(my_df.iloc[0]) # prints: the first row
Using NumPy Arrays: NumPy arrays are similar to Python lists but provide additional features like vectorized operations and broadcasting. You can access specific elements in an array using indexing or slicing:
import numpy as np
my_array = np.array([[1, 2], [3, 4]])
print(my_array[0]) # prints: the first row
These examples demonstrate various ways to reference data in Python. Remember that the specific approach depends on your use case and the type of data you're working with.
References:
Python Official Documentation: https://docs.python.org/3/ pandas Documentation: https://pandas.pydata.org/docs/ NumPy Documentation: https://numpy.org/doc/