How to compare two keys in one dictionary in Python?
How to compare two keys in one dictionary in Python?
Comparing two keys in a single dictionary can be done by directly accessing the values associated with those keys, provided that you know the key names. Here are some ways to do so:
Accessing Values using Keys:You can directly access the values associated with specific keys in your dictionary using the following syntax: dict_name[key1]
and dict_name[key2]
.
Example:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
print(person['name']) # Output: John
print(person['age']) # Output: 30
In the above example, we are accessing the values associated with keys 'name'
and 'age'
. If the keys do not exist in your dictionary, Python will throw a KeyError
.
Python dictionaries provide a built-in method called get()
. It is used to safely retrieve the value for a given key.
Example:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
print(person.get('name')) # Output: John
print(person.get('occupation', 'Unknown')) # Output: Unknown (because 'occupation' is not present in the dictionary)
Here, get()
method returns the value for the given key. If the key does not exist in the dictionary, it returns a default value specified as the second argument to the method.
Another way to compare keys is using an if-else statement.
Example:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
if 'name' in person and 'age' in person:
print("Both 'name' and 'age' are present")
else:
print("Either 'name' or 'age' is missing")
print("Comparing keys: ")
if 'name' < 'age':
print("'name' is less than 'age'")
elif 'name' == 'age':
print("'name' is equal to 'age'")
else:
print("'name' is greater than 'age'")
Here, we are checking if the keys exist in the dictionary using the in
operator. Then, based on their values or the conditions you set, you can perform further actions.
In Python, dictionaries allow direct comparison of keys. This means you can compare two keys to see which one is smaller (lexicographically), equal, or larger.
Example:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
print('name' < 'age') # Output: True
print('name' == 'age') # Output: False
print('name' > 'age') # Output: False
Here, we are comparing the keys using comparison operators <
, ==
, and >
. This is only possible if you know that your key names will always be strings.
Remember to handle potential errors when accessing dictionary keys. You can use try-except blocks to catch KeyError
exceptions or use safe methods like get()
to retrieve values for missing keys.
python dict get multiple levels
I'd be happy to help!
In Python, you can access nested dictionaries (i.e., dictionaries within dictionaries) using a combination of dictionary lookups and slicing. Here's an example:
# Define a sample nested dictionary
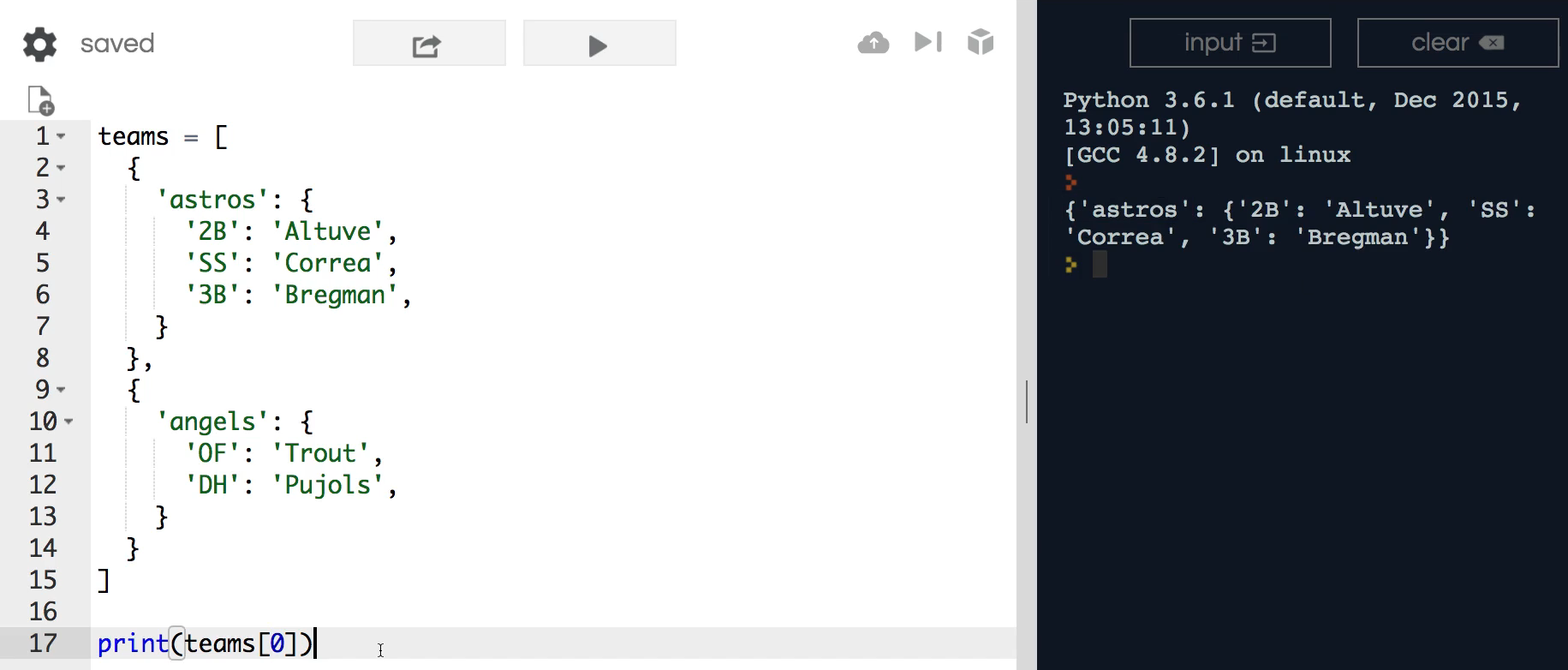
nested_dict = {
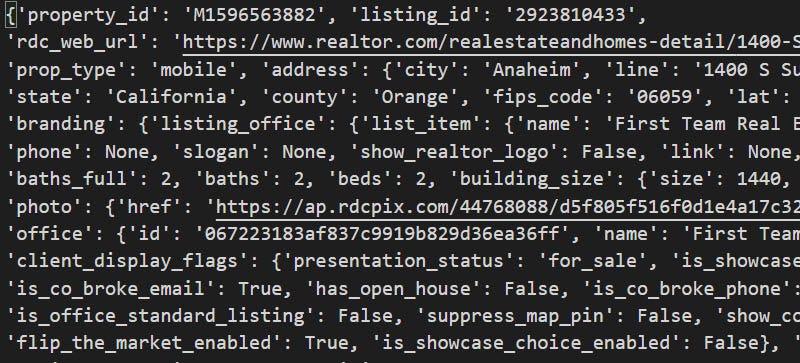
"key1": {"subkey1": "value1", "subkey2": "value2"},
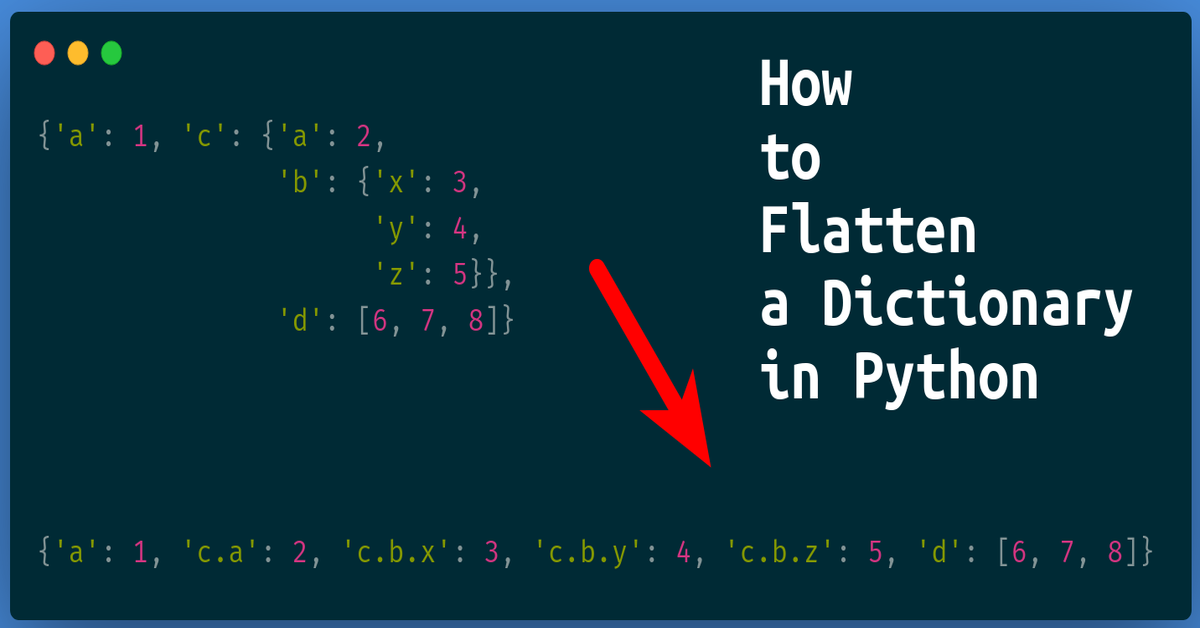
"key2": {"subkey3": "value3"}
}
Access a value at the top level
print(nested_dict["key1"]) # Output: {'subkey1': 'value1', 'subkey2': 'value2'}
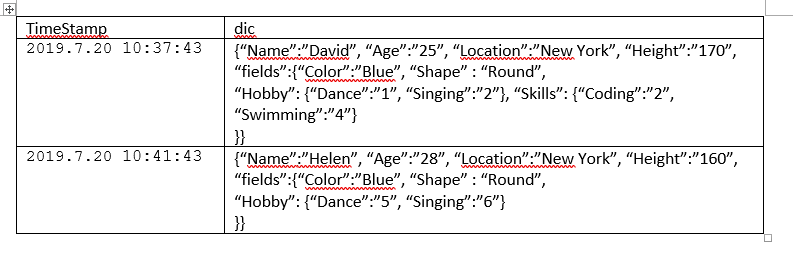
Access a sub-dictionary and then a value within it
print(nested_dict["key1"]["subkey1"]) # Output: 'value1'
Use the get() method to safely access nested values
nested_value = nested_dict.get("key3", {}).get("subkey4", None)
print(nested_value) # Output: None (since "key3" doesn't exist in the dictionary)
Create a new key-value pair within an existing sub-dictionary
nested_dict["key1"]["new_subkey"] = "new_value"
print(nested_dict) # Output:
{
"key1": {"subkey1": "value1", "subkey2": "value2", "new_subkey": "new_value"},
"key2": {"subkey3": "value3"}
}
As you can see, accessing nested dictionaries in Python is relatively straightforward. You can use dictionary lookups to navigate down the hierarchy and access specific values.
Now, let's talk about the get()
method. This is a useful tool for safely accessing nested values. When you call dict["key"]
, if the key doesn't exist, you'll get a KeyError
. But with get()
, you can provide a default value (in this case, an empty dictionary {}
) to return instead of throwing an error.
Here's another example that shows how you can use get()
to create new keys and values:
# Create a new key-value pair within an existing sub-dictionary
new_key = "key4"
new_value = {"new_subkey": "new_value"}
nested_dict.get(new_key, {}).update(new_value)
print(nested_dict)
{
"key1": {"subkey1": "value1", "subkey2": "value2", "new_subkey": "new_value"},
"key2": {"subkey3": "value3"},
"key4": {"new_subkey": "new_value"}
}
In this example, we create a new key-value pair by calling get()
with the new key and an empty dictionary as the default value. Then, we update the sub-dictionary with the new value using the update()
method.
I hope this helps! Let me know if you have any questions or need further clarification.