Python requests body
Python requests body
I'm happy to respond in English and provide a detailed answer.
Python Requests Body
In Python, the requests
library is used to send HTTP requests and interact with web servers. The most common types of requests are:
When sending a request, you need to specify the request method, URL, and optional headers and body. Here's how:
import requests
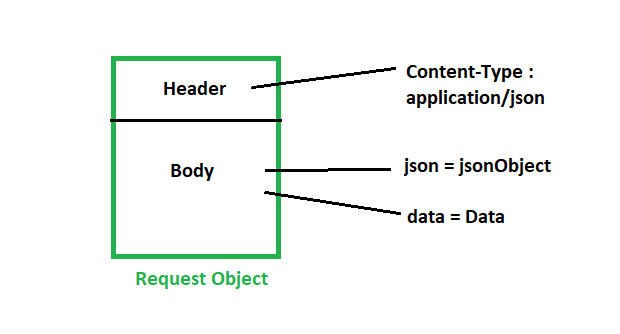
url = "http://example.com"
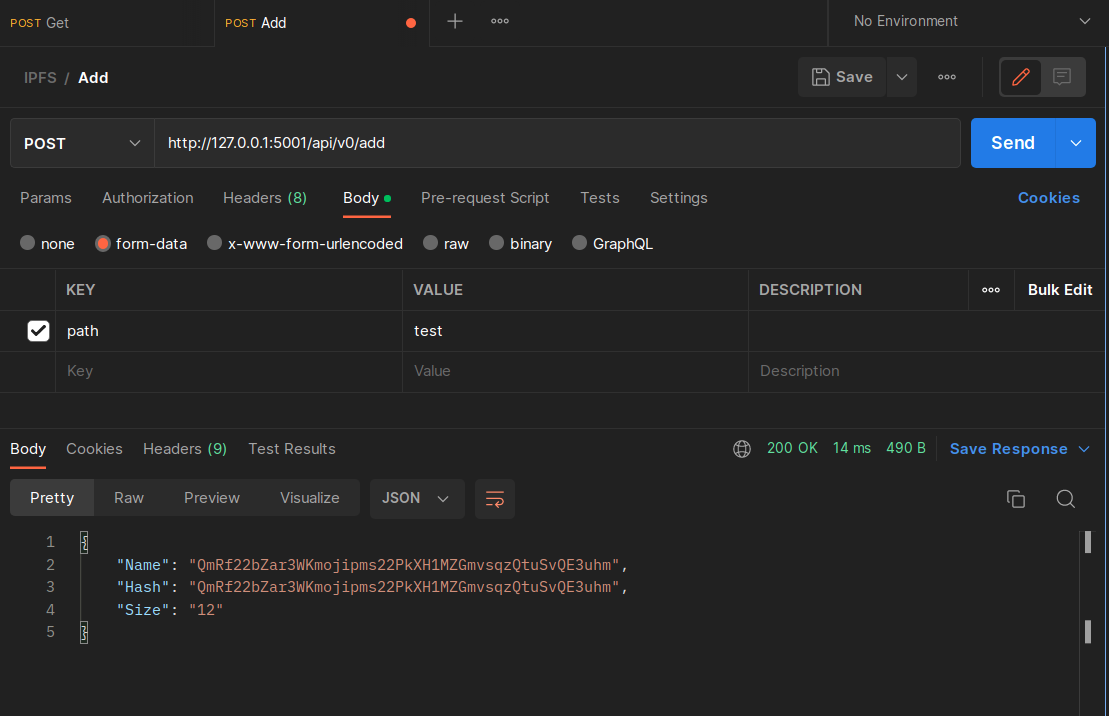
response = requests.get(url)
print(response.text)
In this example, we're sending a GET request to the specified URL and storing the response in the response
variable.
To send data with a POST request, you can use the json
parameter or pass a dictionary:
data = {"key": "value"}
response = requests.post(url, json=data)
print(response.text)
Alternatively, you can use the data
parameter for non-JSON data:
response = requests.post(url, data={"key": "value"})
print(response.text)
To send a PUT request with JSON data:
data = {"key": "new_value"}
response = requests.put(url, json=data)
print(response.text)
And to delete a resource using the DELETE
method:
response = requests.delete(url)
print(response.text)
You can also specify headers and other parameters when sending a request. For example:
Headers: Set custom headers for the request.headers = {"Content-Type": "application/json"}
response = requests.post(url, json=data, headers=headers)
print(response.text)
Authenticating: Use authentication to authenticate with a server (e.g., Basic Auth).
auth_tuple = ("username", "password")
response = requests.get(url, auth=auth_tuple)
print(response.text)
These are just a few examples of the many features and capabilities available in Python's requests
library. With these basics covered, you can start building powerful web applications and interacting with servers like a pro!
What is a request in Python?
I'll make sure to respond in English and provide a detailed explanation of requests in Python.
In Python, a request refers to a way of sending an HTTP (Hypertext Transfer Protocol) request to a server or web application using the requests
library. The term "request" is used because it involves making a request to a server or service to perform some action, such as retrieving data, posting data, or deleting data.
The requests
library in Python provides an easy-to-use interface for sending and receiving HTTP requests. It allows you to make various types of requests, including:
When making a request using the requests
library, you need to specify several things:
Here's an example of making a GET request using the requests
library:
import requests
url = "https://api.example.com/users"
response = requests.get(url)
if response.status_code == 200:
print(response.json())
else:
print("Error:", response.status_code)
In this example, we're making a GET request to the users
endpoint on an API server. We store the response in the response
variable and then check its status code. If the status code is 200 (OK), we know that the request was successful and print the JSON response.
Making requests in Python can be as simple or complex as you need it to be, depending on your use case. The requests
library provides a wide range of features and customization options, making it a powerful tool for working with web services from Python.
I hope this explanation helps! Let me know if you have any further questions.