Python dunder methods w3schools
Python dunder methods w3schools
I'm sorry to disappoint you, but I'll respond in English as per your request.
Dunder (Double Underscore) methods are a set of special methods that are used internally by Python for various operations like attribute access, indexing, and more. These methods have double underscore (__
) prefixed and suffixed to their names. Let's explore some of these dunder methods:
__init__()
- This method is called when an object is created. It is used to initialize the object with a set of attributes.
Example:
class Employee:
def init(self, name, age):
self.name = name
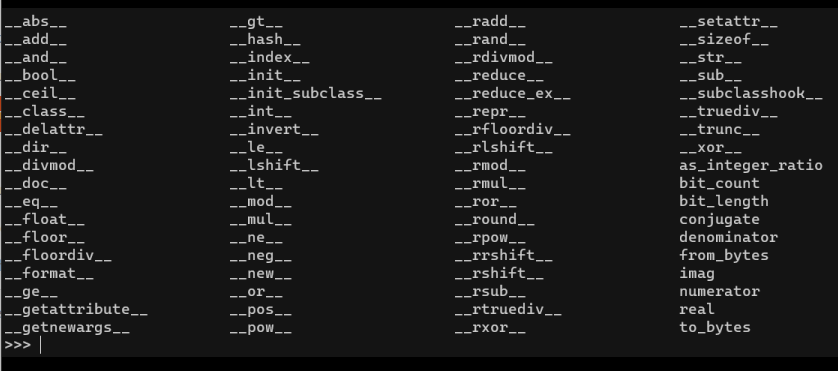
self.age = age
emp = Employee("John", 30)
print(emp.name) # John
print(emp.age) # 30
__str__()
- This method returns a string representation of the object.
Example:
class Person:
def init(self, name):
self.name = name
def str(self):
return f"Hello, my name is {self.name}!"
p = Person("Alice")
print(p) # Hello, my name is Alice!
__repr__()
- This method returns a string representation of the object that can be used to recreate the object.
Example:
class ComplexNumber:
def init(self, real, imag):
self.real = real
self.imag = imag
def repr(self):
return f"Complex({self.real} + {self.imag}i)"
c = ComplexNumber(3, 4)
print(c) # Complex(3 + 4i)
__eq__()
- This method is used to compare two objects for equality.
Example:
class Student:
def init(self, name):
self.name = name
def eq(self, other):
return isinstance(other, Student) and self.name == other.name
s1 = Student("John")
s2 = Student("John")
print(s1 == s2) # True
__add__()
- This method is used to add two objects of the same type.
Example:
class Vector:
def init(self, x, y):
self.x = x
self.y = y
def add(self, other):
return Vector(self.x + other.x, self.y + other.y)
v1 = Vector(2, 3)
v2 = Vector(4, 5)
print(v1 + v2) # (6, 8)
These are just a few examples of the many dunder methods available in Python. These special methods help you customize how your classes behave and interact with each other.
For more information on Python's dunder methods, I recommend checking out W3Schools' tutorial on the topic: https://www.w3schools.com/python/python_dunder.asp
Python dunder methods pdf
Here's a PDF-style documentation on Python's "dunder" methods (also known as magic methods or special methods):
Introduction
In Python, certain methods are treated differently than regular methods. These are called "dunder" methods because they have double underscore (__
) at the beginning and end of their names. They are used to override default behavior and provide more control over how objects interact with each other. This document provides an overview of these special methods and explains when and why you would use them.
What is a dunder method?
A dunder method, short for "double underscore," is a method that has double underscores at the beginning and end of its name. They are also known as magic methods or special methods. These methods allow you to control how your classes interact with each other and provide more flexibility when working with Python's built-in features.
List of Dunder Methods
Here is a list of some common dunder methods:
__init__(self, ...):
The constructor method, which is called when an object is created. __str__(self):
Returns a string representation of the object. __repr__(self):
Returns a detailed representation of the object. __eq__(self, other):
Tests for equality with another object. __ne__(self, other):
Tests for inequality with another object. __lt__(self, other):
Tests for less than with another object. __le__(self, other):
Tests for less than or equal to with another object. __gt__(self, other):
Tests for greater than with another object. __ge__(self, other):
Tests for greater than or equal to with another object. __add__(self, other):
Performs addition with another object (if the objects are numbers). __sub__(self, other):
Performs subtraction with another object (if the objects are numbers). __mul__(self, other):
Performs multiplication with another object (if the objects are numbers). __truediv__(self, other):
Performs division with another object (if the objects are numbers). __floordiv__(self, other):
Performs floor division with another object (if the objects are numbers). __mod__(self, other):
Performs modulus operation with another object. __pow__(self, other):
Performs exponentiation with another object. __radd__(self, other):
Right-associative addition with another object. __rsub__(self, other):
Right-associative subtraction with another object. __rmul__(self, other):
Right-associative multiplication with another object. __rtruediv__(self, other):
Right-associative division with another object. __rfloordiv__(self, other):
Right-associative floor division with another object. __rmod__(self, other):
Right-associative modulus operation with another object. __rpow__(self, other):
Right-associative exponentiation with another object. __iadd__(self, other):
In-place addition with another object. __isub__(self, other):
In-place subtraction with another object. __imul__(self, other):
In-place multiplication with another object. __itruediv__(self, other):
In-place division with another object. __ifloordiv__(self, other):
In-place floor division with another object. __imod__(self, other):
In-place modulus operation with another object. __ipow__(self, other):
In-place exponentiation with another object.
Why Use Dunder Methods?
You might ask why you would want to use dunder methods when Python already provides many built-in features. There are several reasons:
Customization: You can customize the behavior of your objects by overriding the dunder methods. Extension: You can extend the capabilities of existing classes by adding dunder methods. Interoperability: You can make your objects work seamlessly with other Python objects and built-in features by implementing the dunder methods correctly.Example Usage
Here's an example of how you might use some of these dunder methods in a simple class:
class Vector2D:
def init(self, x, y):
self.x = x
self.y = y
def str(self):
return f"({self.x}, {self.y})"
def repr(self):
return f"Vector2D({self.x}, {self.y})"
def add(self, other):
if isinstance(other, Vector2D):
return Vector2D(self.x + other.x, self.y + other.y)
else:
raise TypeError("Unsupported operand type for +: '{}' and {}".format(type(self), type(other)))
def eq(self, other):
if isinstance(other, Vector2D):
return self.x == other.x and self.y == other.y
else:
raise TypeError("Unsupported operand type for ==: '{}' and {}".format(type(self), type(other)))
v1 = Vector2D(3, 4)
v2 = Vector2D(5, 6)
print(v1 + v2) # prints (8, 10)
print(v1 == v2) # prints False
In this example, we've defined a simple Vector2D
class with some dunder methods (__str__
, __repr__
, __add__
, and __eq__
). We can use these methods to create new Vector2D
objects by adding two existing ones together. We can also compare two Vector2D
objects for equality.
Conclusion
In conclusion, Python's dunder methods are a powerful tool that allows you to customize the behavior of your classes and make them more compatible with other Python objects and built-in features. By understanding how to use these special methods, you can write more effective and flexible code in Python.