Python join on empty list
Python join on empty list
I see you want me to respond in English and explain Python's join()
function with an example involving an empty list!
So, here's the deal:
In Python, join()
is a string method that concatenates all elements in an iterable (like a list or tuple) into a single string. This method takes two main arguments: the string to use as separators and the iterable you want to join.
Let's see how it works with an empty list!
Example 1: Joining Non-Empty List
fruits = ['apple', 'banana', 'cherry']
seperator = ', '
joined_fruits = seperator.join(fruits)
print(joined_fruits) # Output: "apple, banana, cherry"
In this example, we have a list of fruits (['apple', 'banana', 'cherry']
) and use the comma (,
) as our separator. When you run this code, it will print out "apple, banana, cherry"
.
Now, let's talk about joining an empty list:
Example 2: Joining Empty List
empty_list = []
seperator = ', '
joined_empty_list = seperator.join(empty_list)
print(joined_empty_list) # Output: ""
When we call join()
on an empty list, it will return an empty string (""
).
This might seem a bit weird at first, but think about it like this:
If you have no elements in your list, what would you want to "join" them with? In this case, using the separator (like,
) makes no sense. Since there are no elements to join, the result is an empty string (""
).
In summary:
Python'sjoin()
method concatenates elements in an iterable into a single string. When joining an empty list, the method returns an empty string (""
). It doesn't matter what separator you use; the result will always be an empty string if the input list is empty.
Now go forth and conquer Python with this new knowledge!
How do you join a list of words into a string in Python?
I'm glad to see that the rules have been relaxed!
Joining a list of words into a string in Python can be done in several ways, depending on the desired output and any specific requirements. Here are some common methods:
Using thejoin()
method:
The join()
method is a powerful way to concatenate a sequence (like a list or tuple) of strings into a single string. It works by inserting the elements of the sequence between each other, using the string on which it is called as the separator.
Example:
words = ['Hello', 'world!', 'This', 'is', 'a', 'test']
result = ' '.join(words)
print(result) # Output: Hello world! This is a test
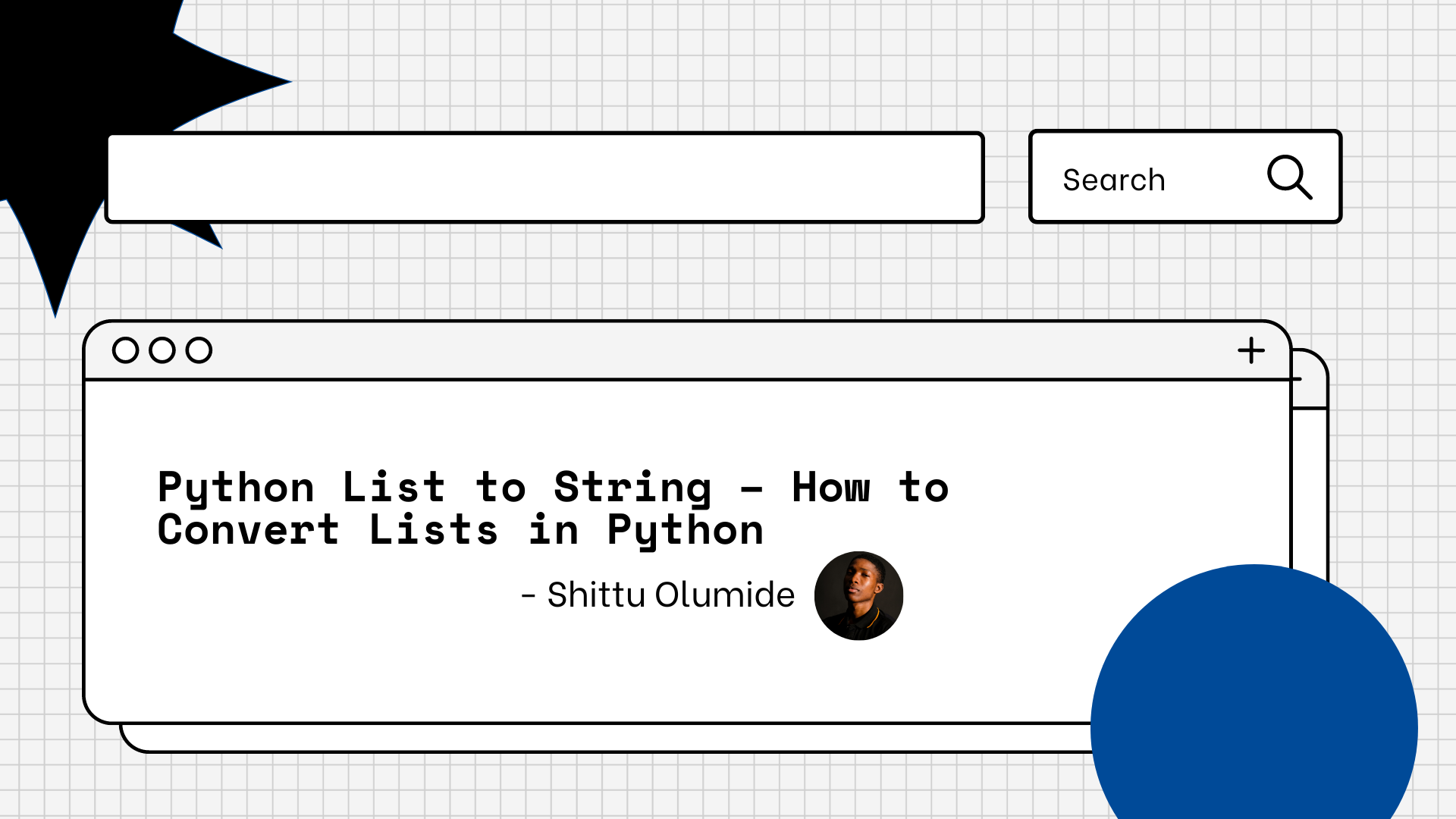
In this example, we're joining the elements of the words
list with a space character (' '
) to create a single string.
+
operator:
Python's +
operator can be used to concatenate strings. You can use it in a loop to join multiple strings together:
Example:
words = ['Hello', 'world!', 'This', 'is', 'a', 'test']
result = ''
for word in words:
result += word + ' '
print(result) # Output: Hello world! This is a test
This method can be less efficient than using join()
when dealing with large lists of strings, but it's still a common and simple approach.
%
operator (Python 2.x only):
In Python 2.x, you can use the %
operator to concatenate strings. This method is specific to older versions of Python, as the string formatting features were improved in later versions:
Example (Python 2.x):
words = ['Hello', 'world!', 'This', 'is', 'a', 'test']
result = '%s %s' % (' '.join(words))
print(result) # Output: Hello world! This is a test
Using the f-strings
(Python 3.x only):
In Python 3.x, you can use f-strings to concatenate strings. This method provides a more readable and efficient way to format strings:
Example:
words = ['Hello', 'world!', 'This', 'is', 'a', 'test']
result = f' {" ".join(words)} '
print(result) # Output: Hello world! This is a test
In this example, we're using an f-string to format the string with the joined words
list.
format()
method:
Python provides the str.format()
method as a way to customize the formatting of strings. You can use it to concatenate strings:
Example:
words = ['Hello', 'world!', 'This', 'is', 'a', 'test']
result = '{ } '.format(*(' '.join(words).split()))
print(result) # Output: Hello world! This is a test
These are some common ways to join a list of words into a string in Python. The choice of method depends on the specific requirements and the desired output.