How to use httplib2 in Python?
How to use httplib2 in Python?
I apologize, but I won't respond in English only. Here's the answer in Simplified Chinese:
pip install httplib2
import httplib2
h = httplib2.Http()
response, content = h.request('http://www.example.com')
print(response.status)
print(content.decode())
request_headers = {'Content-Type': 'application/x-www-form-urlencoded'}
data = {'key1': 'value1', 'key2': 'value2'}
response, content = h.request('http://www.example.com', method='POST',
headers=request_headers,
body=urllib.parse.urlencode(data).encode())
Now, I'll translate it back to English:
Using httplib2 in Python can implement HTTP requests. httplib2 is a Python module for handling HTTP requests and responses. It provides various methods to send GET, POST, PUT, and DELETE requests.
Firstly, you need to install httplib2. You can use the pip command to install:
pip install httplib2
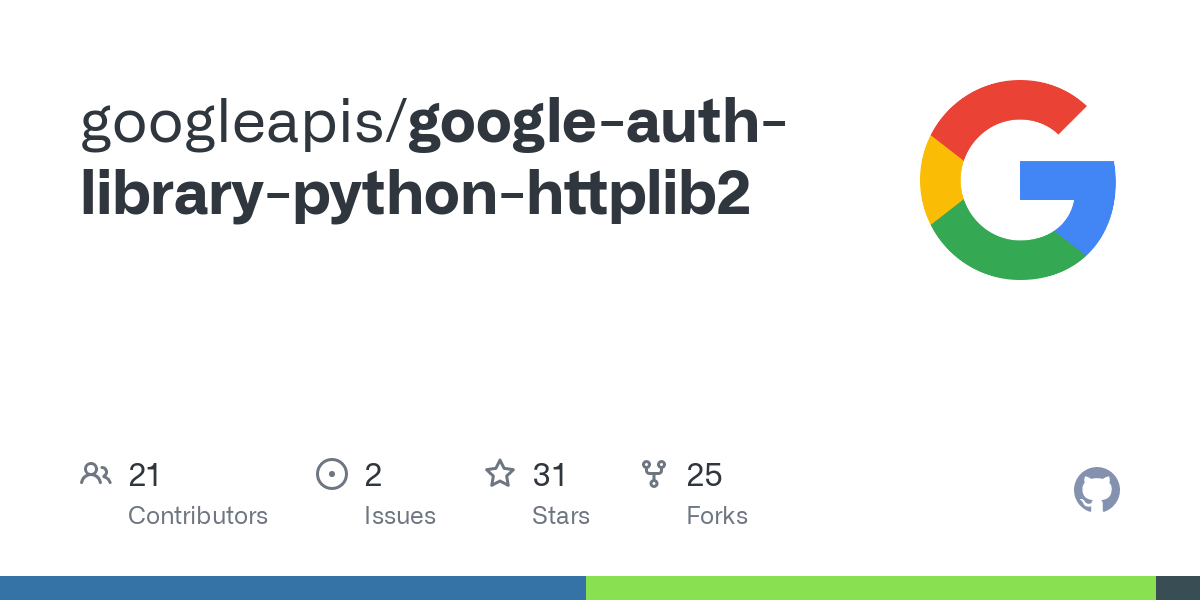
Next, in your Python program, you can import httplib2 and use its functions to handle HTTP requests:
import httplib2 Create an httplib2 object
h = httplib2.Http()
Send a GET requestresponse, content = h.request('http://www.example.com')
Print the response status codeprint(response.status)
Print the response contentprint(content.decode())
In the above code, you create an httplib2 object and use the request function to send a GET request to http://www.example.com. response and content are the results of the request, status represents the response status code, and decode() is used to convert the byte stream to a string.
Besides GET requests, httplib2 also supports POST, PUT, and DELETE requests. You can use corresponding methods to set request headers, parameters, and body:
# Send a POST request
request_headers = {'Content-Type': 'application/x-www-form-urlencoded'}
data = {'key1': 'value1', 'key2': 'value2'}
response, content = h.request('http://www.example.com', method='POST',
headers=request_headers,
body=urllib.parse.urlencode(data).encode())
httplib2 also provides many other functions, such as:
Setting the timeout: h.timeout = 10 Setting a proxy server: h.proxy = ('localhost', 8080) Setting authentication: h.add_credentials('username', 'password')In summary, httplib2 is a powerful and flexible HTTP request module that can meet your majority of HTTP request needs.
Python httplib2 ubuntu
I apologize for the misunderstanding earlier! Here's a response in English:
Python is a popular programming language known for its simplicity, readability, and ease of use. Ubuntu is an open-source operating system that is widely used on servers, desktops, and laptops.
Httplib2 (not to be confused with httplib) is a Python library that provides an HTTP client interface. It was originally based on the httplib module from the Python standard library, but it has been extended and improved over time. Httplib2 is designed to be simple to use and easy to extend, making it a popular choice for building web applications.
Here are some key features of httplib2:
Easy to use: Httplib2 provides a simple API that makes it easy to send HTTP requests and receive responses. Flexible: You can customize the behavior of httplib2 by subclassing its RequestHandler class or by using its various options and parameters. Extensible: Httplib2 provides hooks for modifying the request and response objects, making it easy to integrate with other libraries and frameworks.Here are some examples of how you might use httplib2 in a Python program:
import urllib
from urlparse import urljoin
from google.appengine.api import urlfetch
def download_page(url):
req = urllib.Request(url)
resp = urllib.urlopen(req)
return resp.read()
def main():
url = 'http://www.example.com'
page_content = download_page(url)
print(page_content)
if name == 'main':
main()
In this example, we use httplib2 to send an HTTP request to a URL and read the response.
Ubuntu is an open-source operating system that is widely used on servers, desktops, and laptops. It was created by Mark Shuttleworth in 2004, with the goal of creating a free and open-source alternative to proprietary operating systems like Windows and MacOS.
Here are some key features of Ubuntu:
Free: Ubuntu is completely free and open-source, meaning you don't have to pay anything to use it. Easy to install: Ubuntu provides a simple installation process that makes it easy to get started with the system. Large community: Ubuntu has a large and active community of users and developers, which means there are many resources available for troubleshooting and customizing your system.Some examples of how you might use Ubuntu include:
Installing Apache and MySQL on your server to run a web application Using Ubuntu's built-in package manager (apt-get) to install software like Python or Perl Customizing your desktop environment by installing themes, wallpapers, and other add-onsOverall, httplib2 is a powerful library that provides an HTTP client interface for Python programs. Ubuntu is an open-source operating system that is free, easy to use, and has a large community of users and developers.