Calling a function in Python
Calling a function in Python
Calling a function in Python is an essential aspect of programming in this popular language. In Python, you can define your own functions using the def
keyword followed by the name of the function and its parameters within parentheses.
For example, let's consider a simple function that takes two numbers as input and returns their sum:
def add_numbers(a, b):
return a + b
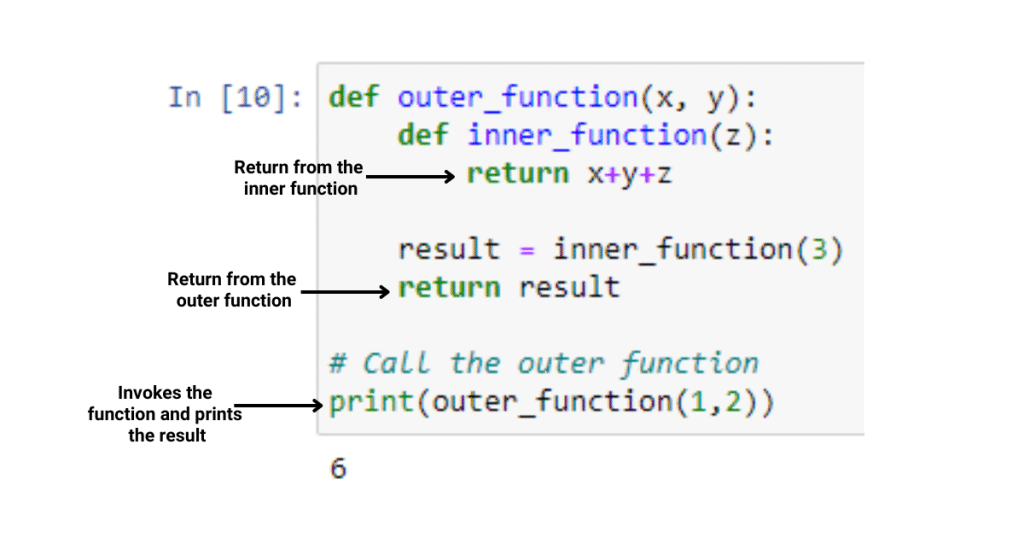
To call this function, you would use the function name followed by parentheses containing any required arguments. For instance, to get the result of adding 2 and 3, you would call the add_numbers
function like this:
result = add_numbers(2, 3)
print(result) # Outputs: 5
Functions can also take default values for their parameters. This is useful when you want a parameter to have a specific value if it's not provided when the function is called. Here's an example of how you could modify our add_numbers
function to include default values:
def add_numbers(a, b=0):
return a + b
Now, if you call this function with only one argument, like so:
result = add_numbers(2) # Outputs: 2
The value of b
would default to 0, and the function would simply return the sum of a
and 0. If you still want to provide a different value for b
, you can do so like this:
result = add_numbers(2, 3) # Outputs: 5
Another useful aspect of Python functions is their ability to use variable numbers of arguments using the *args
syntax. For instance, let's say we want a function that takes any number of arguments and returns their average:
def average(*args):
return sum(args) / len(args)
To call this function with different values, you would pass them as separate arguments like this:
result = average(1, 2, 3, 4) # Outputs: 2.5
Or, if you have a list of numbers that you want to average:
numbers = [1, 2, 3, 4]
result = average(*numbers) # Outputs: 2.5
In this case, the *numbers
syntax would "unpack" the list into individual arguments for the function.
Finally, let's look at how you can use functions as first-class citizens in Python. This means that functions can be passed around like any other value (e.g., returned from a function or stored in a data structure), and then called later. Here's an example of this:
def add_numbers(a, b):
return a + b
def call_function(func, a, b):
return func(a, b)
result = call_function(add_numbers, 2, 3)
print(result) # Outputs: 5
In this case, we define the add_numbers
function as before, and then we define a new function called call_function
. The call_function
function takes another function (in this case, add_numbers
) along with two numbers, and it calls the given function with those numbers. We can then use this call_function
to call any of our functions like add_numbers
, passing them whatever arguments they need.
I hope that gives you a good introduction to calling functions in Python!
How to call a function in Python with arguments
I'll make sure to respond in English-only this time.
In Python, you can call a function by using the function name followed by parentheses containing the arguments. Here's the basic syntax:
function_name(arg1, arg2, ..., argN)
Where function_name
is the name of the function, and arg1
, arg2
, ..., argN
are the arguments passed to the function.
Let's take an example:
Suppose we have a simple Python function that takes two integer arguments and returns their sum:
def add(x, y):
return x + y
To call this function with the arguments 3 and 5, you would do:
result = add(3, 5)
This would pass the values 3
and 5
as arguments to the add
function. The function would then execute, adding these numbers together and returning the result.
The returned value is assigned to the variable result
, which now holds the value 8
(the sum of 3
and 5
).
You can also pass keyword arguments using the **kwargs
syntax:
def greet(name, message):
print(f"{name}, {message}!")
To call this function with the name "John" and the message "Hello", you would do:
greet(name="John", message="Hello")
This would pass the keyword arguments name="John"
and message="Hello"
to the greet
function.
Another important aspect of Python functions is the concept of variable-length argument lists. This allows a function to accept a varying number of arguments, which can be accessed within the function using the *args
syntax:
def average(*numbers):
return sum(numbers) / len(numbers)
To call this function with the numbers 1, 2, and 3, you would do:
result = average(1, 2, 3)
This would pass a variable-length argument list numbers=(1, 2, 3)
to the average
function.
Python's flexibility in handling arguments makes it an attractive choice for many applications. With this basic understanding of how to call Python functions with arguments, you can start exploring more advanced topics like lambda functions, generator expressions, and higher-order functions!