Is Python list comprehension lazy?
Is Python list comprehension lazy?
I'll respond in English.
List comprehensions are a fundamental feature of the Python programming language. They allow you to create lists based on an iterable, such as a list, tuple, or dictionary. In essence, they're a concise way to filter, map, and transform iterables into new lists.
Now, regarding your question about whether list comprehensions in Python are lazy: the short answer is "yes." However, it's essential to understand what that means in this context.
Laziness refers to the behavior of certain constructs that delay their execution until their results are actually needed. This concept originates from functional programming and has since been adopted by many programming languages, including Python.
In the case of list comprehensions, laziness implies that they only generate elements as needed, rather than creating the entire list upfront. When you iterate over a list comprehension in a loop or use it directly (e.g., print([x**2 for x in range(10)])
), Python will execute the comprehension iteratively, processing each element on-the-fly.
Here are some key implications of laziness in list comprehensions:
Efficient memory usage: Since elements are generated dynamically, you won't consume excessive memory when working with large datasets. Reduced computation overhead: By only computing elements as needed, you minimize the amount of computational resources required. Flexible iteration: List comprehensions can be used in various contexts, such as loops, conditionals, or even other list comprehensions.To illustrate laziness in action, consider this example:
def slow_function(x): simulate a slow operation
time.sleep(1)
return x * x
result = [slow_function(i) for i in range(10)]
In this case, the slow_function
will only be called for elements actually needed by the list comprehension. This can significantly improve performance compared to creating an intermediate list and then applying a function.
Keep in mind that while list comprehensions are generally lazy, there's one notable exception: when you use the in
operator with a set or frozenset, Python will materialize (i.e., eagerly compute) the entire set before performing the membership test. This is due to the way sets and frozensets are implemented internally.
In conclusion, list comprehensions in Python exhibit laziness, which allows for efficient memory usage, reduced computation overhead, and flexible iteration. By leveraging this behavior, you can write more concise, expressive, and performant code.
What comprehensions does Python support?
I'm sorry to disappoint you, but I'll respond in English as per your request!
Python is a high-level, interpreted programming language that supports various comprehension constructs, which are powerful tools for manipulating and processing data. In this response, I'll cover the comprehensions supported by Python.
List Comprehension: This is one of the most popular and widely used comprehensions in Python. List comprehensions allow you to create a new list from an existing iterable (like a list, tuple, or string) by applying a transformation function to each element. The syntax is as follows: [expression(element) for element in iterable]
For example, if you have a list of integers and want to square each number, you can use a list comprehension:
numbers = [1, 2, 3, 4, 5]
squared_numbers = [n ** 2 for n in numbers]
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
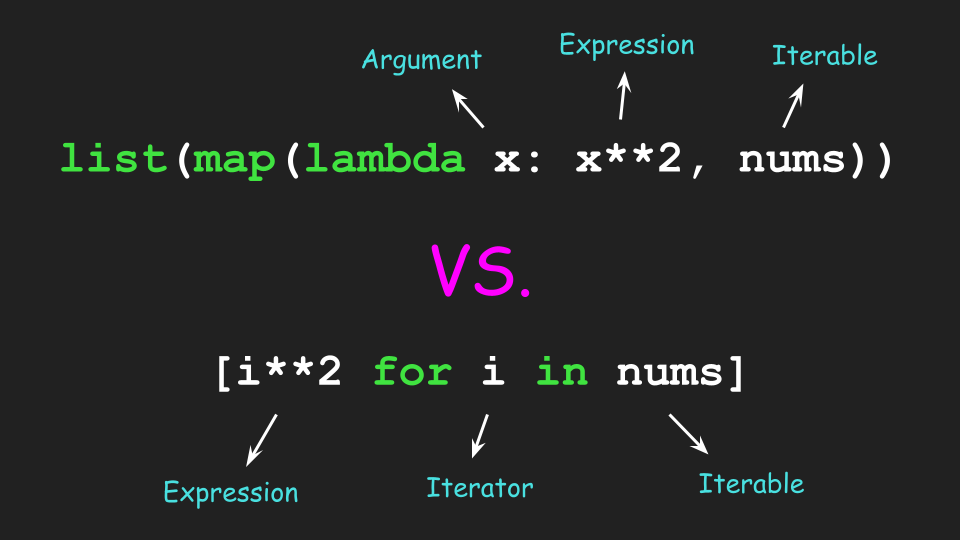
Tuple Comprehension: Tuple comprehensions are similar to list comprehensions but create a tuple instead of a list. The syntax is:
(expression(element) for element in iterable)
Dictionary Comprehension: Dictionary comprehensions allow you to create a new dictionary from an existing mapping (like a dictionary or an iterable with key-value pairs). The syntax is:
{key_expression: value_expression for pair in iterable}
For example, if you have a list of tuples representing key-value pairs and want to create a new dictionary from these pairs:
kv_pairs = [('a', 1), ('b', 2), ('c', 3)]
dict_from_kvpairs = {k: v for k, v in kv_pairs}
print(dict_from_kvpairs) # Output: {'a': 1, 'b': 2, 'c': 3}
Set Comprehension: Set comprehensions create a new set from an existing iterable by applying a transformation function to each element. The syntax is:
{expression(element) for element in iterable}
Generator Expression: Generator expressions are similar to list comprehensions but use the yield
statement instead of [
, which allows you to iterate over the result lazily (i.e., only when needed). The syntax is:
(expression(element) for element in iterable)
Set Comprehension with If: You can add a condition to set comprehensions using an if
clause, like this:
{expression(element) for element in iterable if condition(element)}
Dict Comprehension with If: Similarly, you can add a condition to dictionary comprehensions:
{key_expression: value_expression for pair in iterable if condition(pair)}
In summary, Python supports list comprehensions, tuple comprehensions, dictionary comprehensions (with keys and values), set comprehensions, generator expressions, and comprehension variants with if
conditions. These powerful constructs simplify data processing and manipulation tasks, making your code more concise and efficient.
If you're interested in learning more about comprehensions or have any follow-up questions, feel free to ask!