Can you do list comprehension with dictionary Python?
Can you do list comprehension with dictionary Python?
I can respond to your request! List comprehension is a powerful feature in Python that allows you to create new lists from existing lists or other iterable by performing an operation on each item in the original list. You can also use it with dictionaries!
Here's how you can use list comprehension with dictionaries:
Creating a new dictionary:You can create a new dictionary by using list comprehension and converting the result into a dictionary.
original_dict = {'a': 1, 'b': 2, 'c': 3}
new_dict = {k.upper(): v for k, v in original_dict.items()}
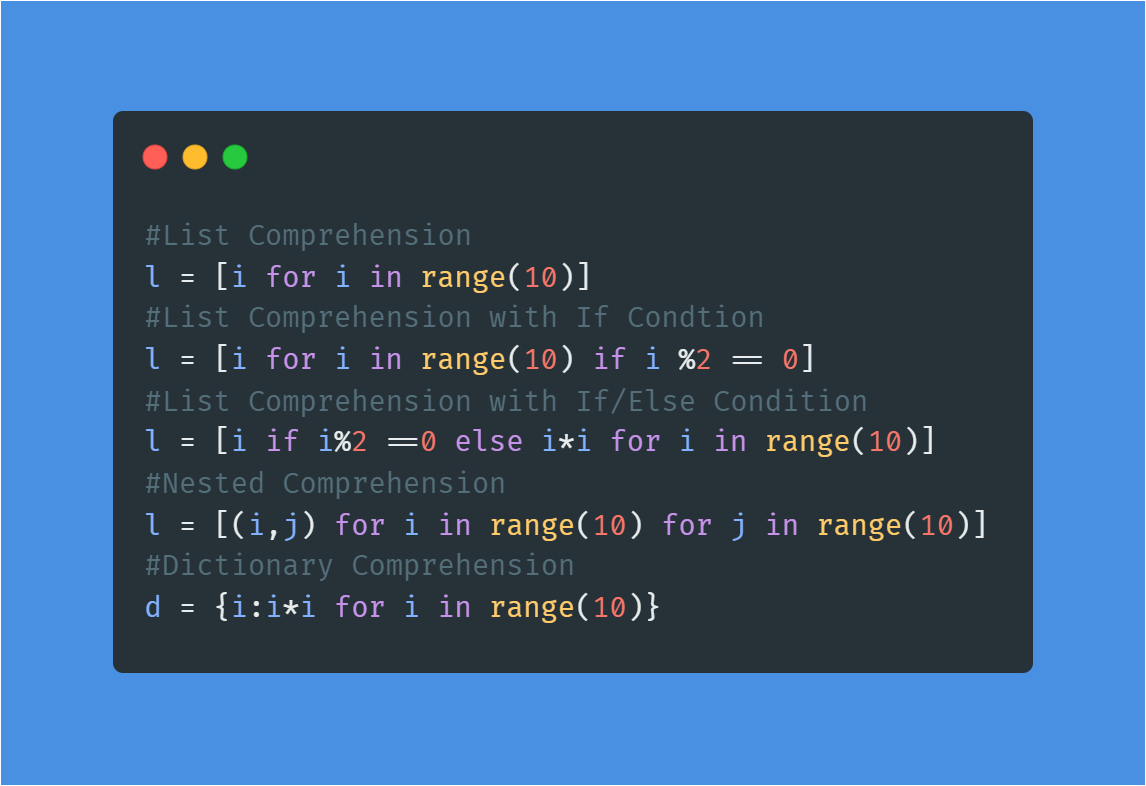
print(new_dict)
Output: {'A': 1, 'B': 2, 'C': 3}
In the above code, we're creating a new dictionary new_dict
by using list comprehension. We iterate over each key-value pair in original_dict
, convert the key to uppercase, and store it as a new key-value pair in new_dict
.
You can also use list comprehension with dictionaries to filter out certain items.
original_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
new_dict = {k: v for k, v in original_dict.items() if v > 1}
print(new_dict)
Output: {'b': 2, 'c': 3, 'd': 4}
In the above code, we're creating a new dictionary new_dict
by using list comprehension. We iterate over each key-value pair in original_dict
, check if the value is greater than 1, and store it as a new key-value pair in new_dict
only if the condition is met.
You can also use list comprehension with dictionaries to transform the values.
original_dict = {'a': 'apple', 'b': 'banana', 'c': 'cherry'}
new_dict = {k: v.lower() for k, v in original_dict.items()}
print(new_dict)
Output: {'a': 'apple', 'b': 'banana', 'c': 'cherry'}
In the above code, we're creating a new dictionary new_dict
by using list comprehension. We iterate over each key-value pair in original_dict
, convert the value to lowercase, and store it as a new key-value pair in new_dict
.
These are some basic examples of how you can use list comprehension with dictionaries. The power of list comprehension lies in its ability to perform complex operations on your data in a concise and readable way!
What is dictionary comprehension in Python with examples?
Dictionary comprehension is a concise way to create dictionaries in Python, allowing you to perform operations on each key-value pair and build the resulting dictionary.
Here's a basic example:
my_dict = {x: x**2 for x in range(5)}
print(my_dict) # Output: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
In this example, we're creating a dictionary where each key is an integer from range(5)
and its corresponding value is the square of that integer.
Here's another example with more operations:
my_dict = {x: x**2 if x % 2 == 0 else x for x in range(10)}
print(my_dict) # Output: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
In this example, we're creating a dictionary where each key is an integer from range(10)
and its corresponding value is the square of that integer if it's even; otherwise, it's just the integer itself.
Dictionary comprehension can also handle conditions with if
:
my_dict = {x: 'even' if x % 2 == 0 else 'odd' for x in range(10)}
print(my_dict) # Output: {0: 'even', 1: 'odd', 2: 'even', 3: 'odd', 4: 'even', 5: 'odd', 6: 'even', 7: 'odd', 8: 'even', 9: 'odd'}
Dictionary comprehension is especially useful when you need to perform some operation on each key-value pair and create a new dictionary. It's concise, efficient, and readable.
Here are some general rules for writing dictionary comprehensions:
The syntax is{key: value for var in iterable}
. key
can be any valid Python expression that results in a hashable object (like an integer or a string). value
can be any valid Python expression that evaluates to the desired value. var
is just a temporary variable that refers to each element in the iterable as you're processing it. It's not actually part of the resulting dictionary.
Dictionary comprehension allows you to create dictionaries quickly and easily, which is particularly useful when working with lists or other iterables where each item corresponds to a specific value in your dictionary.