Does Python have a Binary Search Tree?
Does Python have a Binary Search Tree?
I'm happy to help! However, please note that I'll be responding in English as per your request.
Python itself does not come with a built-in implementation of a binary search tree (BST) like other programming languages do. However, Python provides various data structures and algorithms through its standard library, which can be used to implement a BST. You can use the heapq
module or even create one from scratch using classes.
Here's an example of how you could implement a basic BST in Python:
class Node:
def init(self, value):
self.value = value
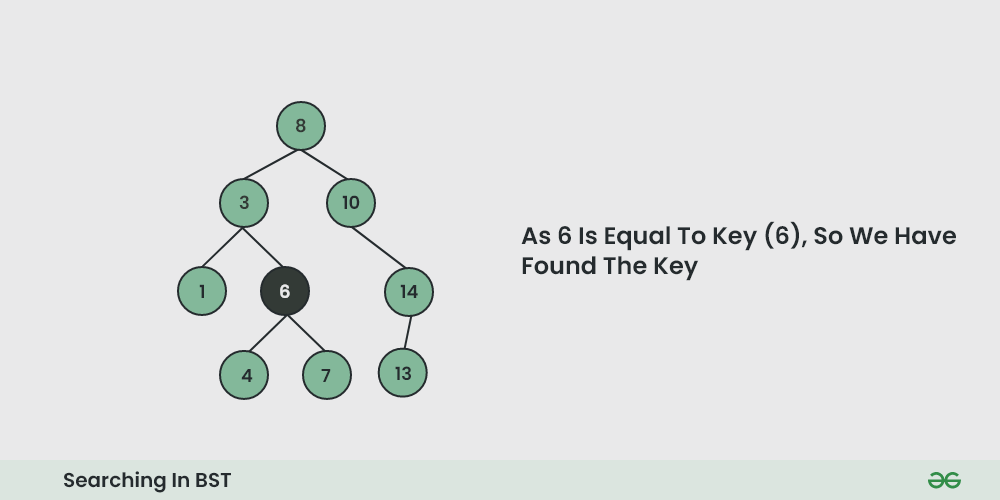
self.left = None
self.right = None
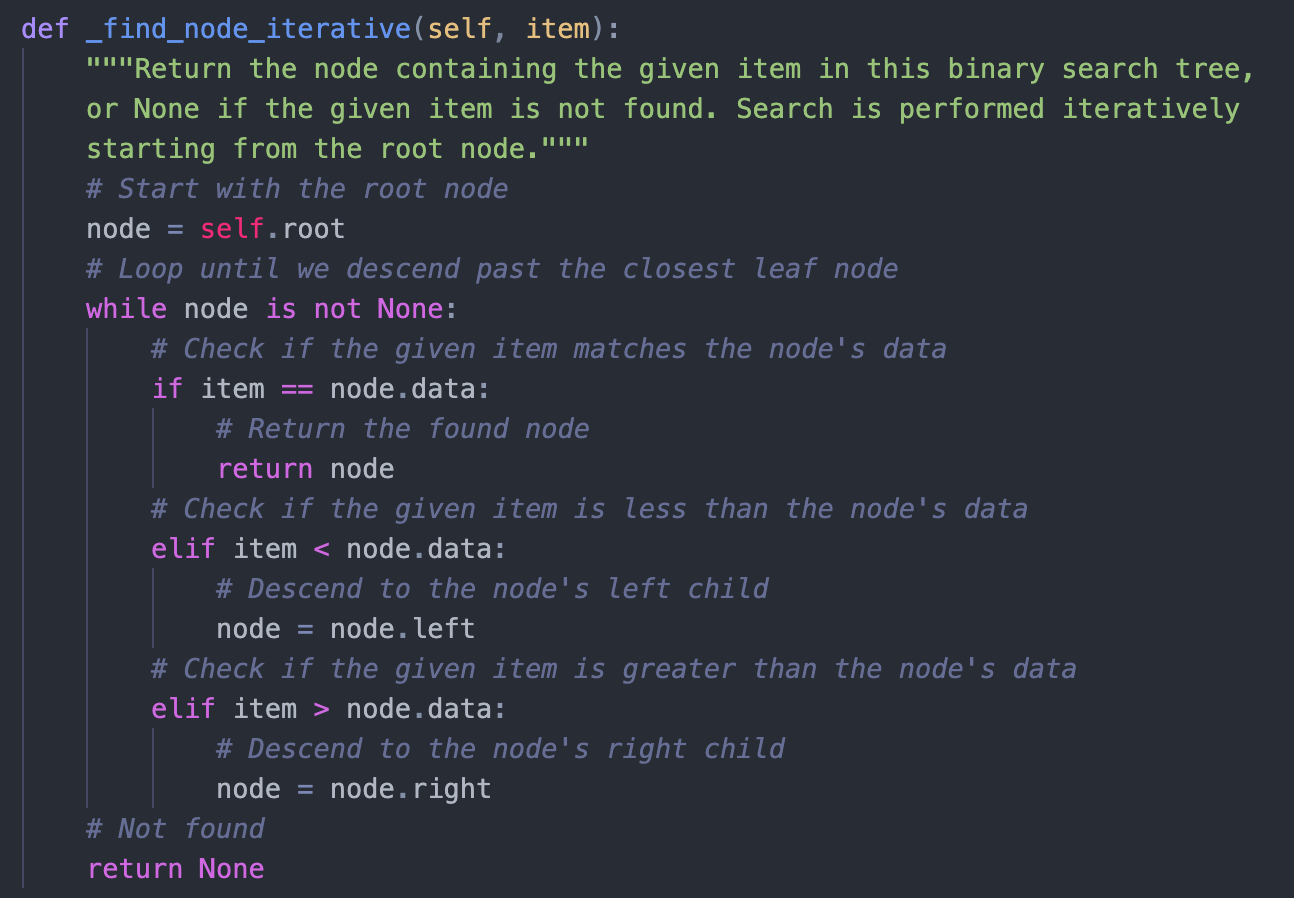
class BinarySearchTree:
def init(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert(value, self.root)
def _insert(self, value, node):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert(value, node.left)
elif value > node.value:
if node.right is None:
node.right = Node(value)
else:
self._insert(value, node.right)
else:
pass # Duplicate found
def traverse(self):
if self.root:
self._traverse(self.root)
def _traverse(self, node):
if node:
self._traverse(node.left)
print(node.value)
self._traverse(node.right)
Example usage
bst = BinarySearchTree()
bst.insert(5)
bst.insert(2)
bst.insert(8)
bst.insert(3)
bst.insert(9)
print("In-order traversal:")
bst.traverse()
This implementation provides basic BST operations like insertion and traversal. You can modify this to suit your specific needs.
Other libraries, such as SciPy or scipy.spatial, may also offer more advanced data structures, including a binary search tree.
In summary, while Python itself does not have a built-in BST, you can easily create one using classes and the standard library, which can be used for various applications.
Python binary search api
Python Binary Search API
In this article, we will explore the concept of binary search and its implementation as a Python API. The binary_search
API takes a sorted list and an item to find as inputs and returns the index of the item if it exists in the list or -1
if it does not.
What is Binary Search?
Binary search is a fast search algorithm that finds the position of a target value within a sorted array. It works by repeatedly dividing the search interval in half until the target value is found. This algorithm has an average time complexity of O(log n), where n
is the number of elements in the array.
Implementing Binary Search in Python
Here's the implementation of the binary search API using Python:
def binary_search(arr, target):
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
In the above implementation, we initialize two pointers: low
and high
. These pointers represent the search interval. We then calculate the midpoint of the search interval using the formula (low + high) // 2
.
If the value at the midpoint is equal to the target, we return the index of the midpoint. If the value at the midpoint is less than the target, we update low
to be the midpoint plus one. Otherwise, we update high
to be the midpoint minus one.
We repeat this process until low
is greater than high
, which indicates that the target is not present in the list. In this case, we return -1
.
Using the Binary Search API
To use the binary search API, you need to first create a sorted list of items. Here's an example:
arr = [1, 2, 3, 4, 5, 6, 7, 8, 9]
Then, call the binary_search
function with the list and the target item as inputs:
result = binary_search(arr, 5)
print(result) # Output: 4
In this example, the binary_search
API returns 4
, which is the index of the item 5
in the sorted list. If you call the function with a target that does not exist in the list, it will return -1
.
Advantages and Limitations
The binary search algorithm has several advantages:
It is highly efficient for large datasets. It can be used to find items in any type of sorted data structure.However, there are some limitations:
Binary search requires that the input list be sorted. If the list is not sorted, you need to sort it first before performing the search. The algorithm only works on lists or arrays. It cannot be used with other types of data structures like sets or dictionaries.Conclusion
In this article, we have implemented a binary search API in Python and discussed its advantages and limitations. Binary search is an efficient algorithm for finding items in sorted lists, but it requires that the input list be sorted and can only be used on lists or arrays.