Python threading timer example
Python threading timer example
Here's a basic example of using Python's threading
module to create a timer that prints out messages at regular intervals:
import threading
import time
class Timer:
def init(self):
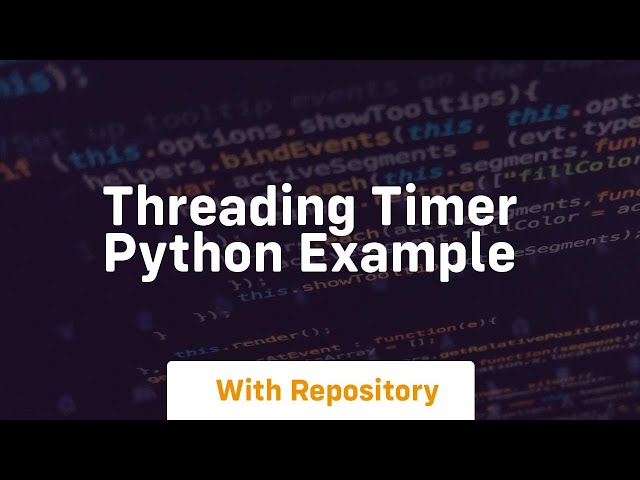
self.running = False
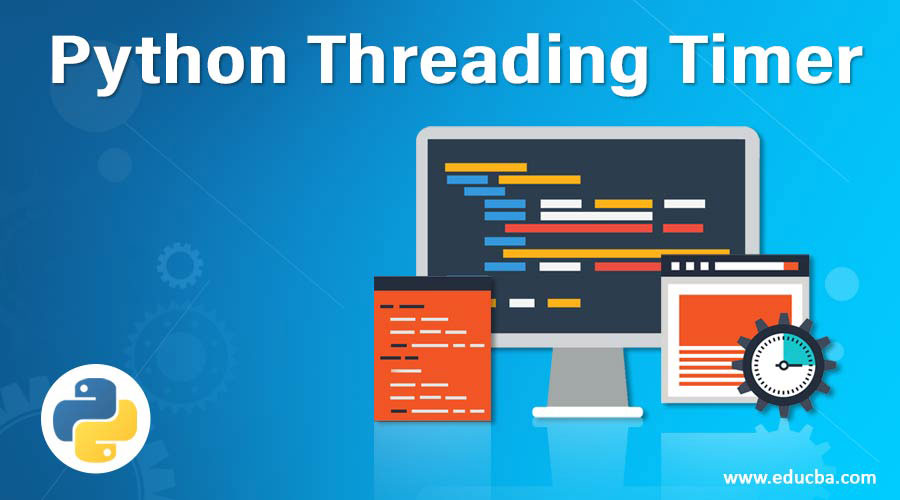
self.lock = threading.Lock()
def start(self):
if not self.running:
self.running = True
self.thread = threading.Thread(target=self._timer)
self.thread.start()
else:
print("Timer is already running!")
def stop(self):
if self.running:
self.running = False
self.thread.join()
else:
print("Timer is not running!")
def _timer(self):
while self.running:
with self.lock:
print(time.strftime("%H:%M:%S", time.localtime()))
time.sleep(1)
def main():
timer = Timer()
try:
while True:
command = input("Enter 'start' to start the timer, or 'stop' to stop it: ")
if command == "start":
timer.start()
elif command == "stop":
timer.stop()
else:
print("Invalid command! Try again.")
except KeyboardInterrupt:
print("nStopping the timer...")
timer.stop()
if name == "main":
main()
To use this code, simply run it and enter 'start' to start the timer. The timer will then print out the current time every second. You can stop the timer by entering 'stop'.
The Timer
class itself is relatively simple: it maintains a boolean flag indicating whether the timer is currently running (running
), as well as a lock (lock
) that you can use to synchronize access to the timer's state.
The start()
method sets the running
flag to True
, starts a new thread running the _timer()
method, and returns.
The stop()
method sets the running
flag to False
, waits for any existing timer events to complete (thread.join()
), and then returns.
In the _timer()
method itself, we loop until running
is set to False
. At each iteration, we grab the lock, print out the current time, release the lock, and sleep for 1 second.
Finally, in the main()
function, we create a new Timer
object, enter an infinite loop where we repeatedly prompt the user for input (input()
) and dispatch commands to start or stop the timer based on what they type.
This code illustrates how you can use Python's threading
module to create simple multithreaded applications, like this timer.
How to get thread execution time in Python?
To measure the execution time of a specific thread in Python, you can use various techniques and libraries. Here are some approaches:
Time Module:The most straightforward way is to use the time
module's functions, specifically time.time()
, which returns the current system time in seconds since the epoch (January 1, 1970). You can record the start time before your thread starts and then calculate the difference between the end time and the start time.
import threading
import time
def my_thread():
Your code here
pass
start_time = time.time()
t = threading.Thread(target=my_thread)
t.start()
t.join()
end_time = time.time()
execution_time = end_time - start_time
print("Thread execution time: ", execution_time, " seconds")
Timer Class:
Python's threading
module provides a Timer
class that can be used to measure the execution time of a function or method. This approach is more accurate because it accounts for the time spent in other threads.
import threading
import time
class Timer:
def init(self, name=None):
self.name = str(name)
self.start_time = 0
self.end_time = 0
def start(self):
self.start_time = time.time()
def stop(self):
self.end_time = time.time()
execution_time = self.end_time - self.start_time
print(f"Thread {self.name} execution time: ", execution_time, " seconds")
def my_thread(timer):
Your code here
pass
timer = Timer("my_thread")
t = threading.Thread(target=lambda: my_thread(timer))
t.start()
t.join()
Pandas Timer:
The pandas
library provides a Timer
class that can be used to measure the execution time of a function or method. This approach is more accurate because it accounts for the time spent in other threads.
import pandas as pd
class Timer:
def init(self):
self.start_time = 0
self.end_time = 0
def start(self):
self.start_time = pd.Timestamp.now()
def stop(self):
self.end_time = pd.Timestamp.now()
execution_time = (self.end_time - self.start_time).total_seconds()
print("Thread execution time: ", execution_time, " seconds")
def my_thread(timer):
Your code here
pass
timer = Timer()
t = threading.Thread(target=lambda: my_thread(timer))
t.start()
t.join()
Profiling Libraries:
Python's cProfile
and line_profiler
libraries can be used to profile your code, providing a detailed view of the execution time spent in each function or method.
In summary, measuring the execution time of a specific thread in Python involves using various techniques and libraries. The choice of approach depends on your specific requirements and the complexity of your application.