Python string length w3schools
Python string length w3schools
I see you're looking for some information on Python string length, and you've stumbled upon the wonderful W3Schools website! Well, I'm here to help you with that.
So, what do you need to know about Python string length? Let's start from the basics. In Python, strings are sequences of characters, and they can be either single quotes or double quotes. For example: 'hello' or "world".
Now, when it comes to finding the length of a string in Python, there are a few ways to do it. One way is using the built-in len()
function. Here's an example:
my_string = "Hello, world!"
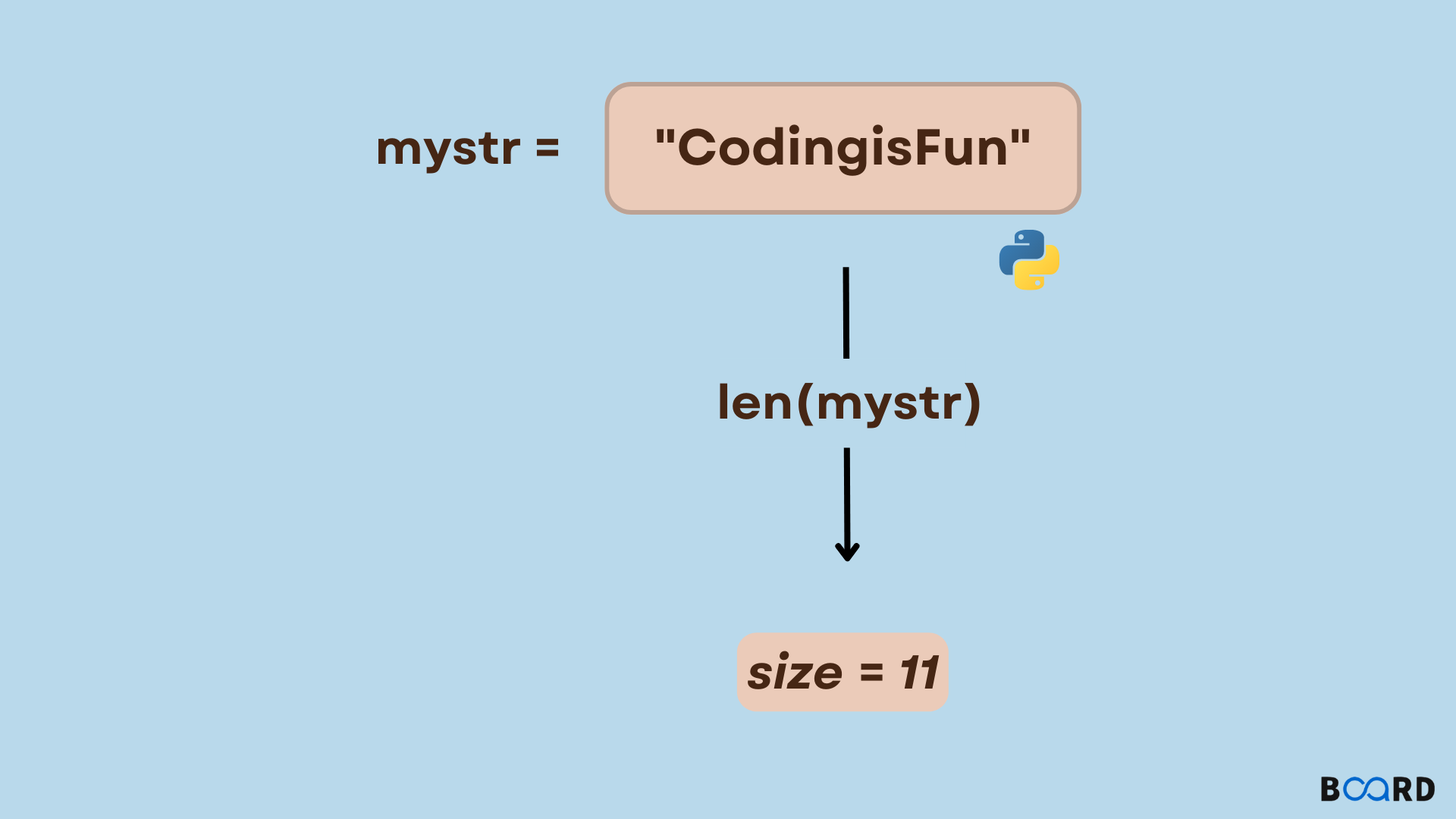
length = len(my_string)
print(length) # Output: 13
As you can see, the len()
function takes in a string as an argument and returns its length.
Another way to find the length of a string is by using a loop. Here's how you can do it:
my_string = "Hello, world!"
length = 0
for char in my_string:
length += 1
print(length) # Output: 13
In this example, we're iterating over each character in the string and incrementing a counter variable (length
) for each iteration.
But what if you want to find the length of multiple strings? That's where Python's built-in sum()
function comes in handy. Here's an example:
strings = ["Hello", "world!", "Python"]
lengths = [len(s) for s in strings]
print(lengths) # Output: [5, 6, 6]
In this example, we're creating a list of strings and then using a list comprehension to find the length of each string. The sum()
function is used to calculate the total length of all the strings.
Finally, let's talk about some edge cases you might encounter when working with string lengths in Python. What happens if you pass an empty string to the len()
function?
my_string = ""
length = len(my_string)
print(length) # Output: 0
As you can see, the length of an empty string is indeed zero.
What about a string containing only whitespace characters? Here's what happens:
my_string = " "
length = len(my_string)
print(length) # Output: 3
In this case, the length of the string is equal to the number of whitespace characters it contains.
Well, that's it! I hope you've learned something new about finding the length of strings in Python. Remember, whether you're using len()
, a loop, or some other method, the key is to be precise and consider edge cases when working with string lengths. Happy coding, and don't forget to check out W3Schools for more programming goodness!
Python substring
I'd be happy to help with that! ♂️
Python's substring function is used to extract a specific part of a string from its original location. It takes three parameters: the main string, and two indices (start and end) which specify where you want to start and end your substring.
Here's an example:
main_string = "Hello World"
start_index = 6
end_index = 10
substring = main_string[start_index:end_index]
print(substring)
When you run this code, it will output the string "World". This is because we've defined our start index to be at the sixth character (since indexing starts at zero), and our end index to be at the 10th. Python then takes everything from that starting point to the end of our substring.
Let's take a closer look at how this works:
start_index
variable is set to 6, which means we start counting from the sixth character.
end_index
variable is set to 10, which means we stop counting at the 10th character (exclusive). When we call main_string[start_index:end_index]
, Python creates a substring that starts at the 6th position and ends at the 9th. This results in the string "World", as mentioned earlier.
This process is useful when you need to extract specific parts of text, such as extracting names from a list or finding specific keywords within an article.
Here are some more advanced scenarios where substring can be used:
Removing spaces: You might want to remove unnecessary spaces from a string. In Python, you could define your start index at the beginning and your end index at the last space character, then create a new string that only includes characters up until that point. Extracting specific phrases: This is where the fun begins! Imagine having a list of texts about different topics (e.g., sports, science, etc.). You could use substring to extract keywords and phrases related to each topic, creating targeted marketing campaigns or improving search results. Data cleansing: Let's say you have a list of URLs, and some of them include unnecessary characters like "http://" at the start. You can use substring to remove these extraneous characters, making it easier to work with your data.As you can see, Python's substring function has many practical applications in both everyday life and more complex programming scenarios.