String slicing Python negative index
String slicing Python negative index
I'm happy to respond in English for this question!
String slicing is a powerful feature in Python that allows you to manipulate strings by extracting specific parts of the string or modifying it in various ways. When using string slicing, you can specify a range of characters you want to extract from the original string.
In standard indexing, Python strings are 0-indexed, meaning that the first character of the string is at index 0, the second character is at index 1, and so on. However, when working with negative indices in Python string slicing, things get a bit more interesting!
Using a negative index, you can access characters from the end of the string. For example:
my_string = "Hello, World!"
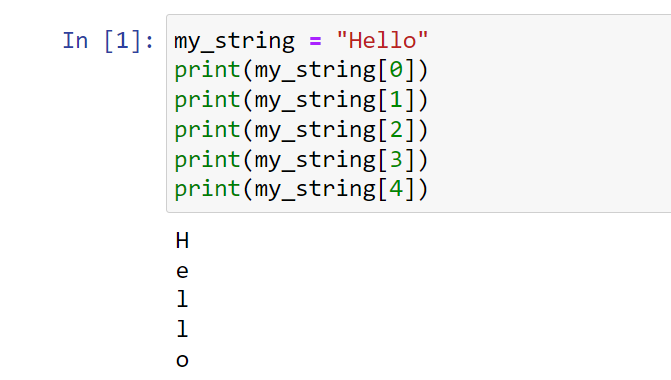
print(my_string[-1]) # prints 'd'
print(my_string[-2]) # prints 'l'
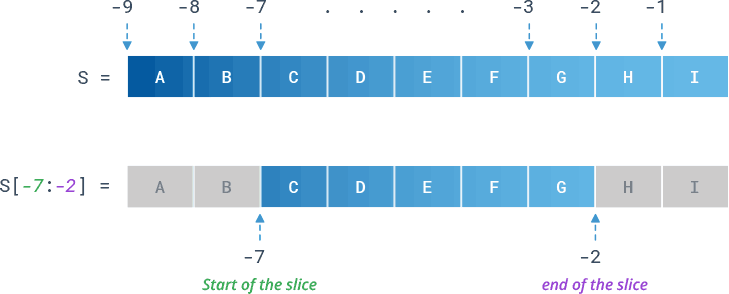
print(my_string[-3]) # prints 'o'
print(my_string[-4]) # prints 'W'
print(my_string[-5]) # prints ','
print(my_string[-6]) # prints 'H'
In the example above, we're using negative indices to access characters from the end of the string. The -1
index refers to the last character ('d'
in this case), the -2
index refers to the second-to-last character ('l'
), and so on.
When you combine standard indexing with negative indexing, things can get quite interesting! Here are some more examples:
my_string = "Hello, World!"
print(my_string[0:5]) # prints 'Hello'
print(my_string[-6:]) # prints 'Hello, World!'
print(my_string[:3]) # prints 'Hel'
print(my_string[1:-2]) # prints 'ell, Wo'
In the above examples, we're using a combination of standard indexing (0:5
, 1:-2
) and negative indexing (-6
, -2
) to extract specific parts of the string.
Why are negative indices useful? Well, for one thing, they can be a huge help when you need to access characters from the end of the string. For example, if you have a URL or file path that you want to extract the last few characters of, using a negative index is much easier than trying to calculate the length of the string and then indexing back from there.
Another benefit of using negative indices is that it can make your code more readable and maintainable. When working with large strings or complex text processing tasks, having a way to easily access characters from the end of the string can be incredibly powerful!
In conclusion, Python's string slicing feature offers a powerful way to manipulate strings by extracting specific parts of the string or modifying it in various ways. Negative indices offer an additional level of flexibility and expressiveness, making it even easier to work with text in your Python code!
String indexing and slicing in Python
String indexing and slicing are fundamental concepts in Python programming that allow you to manipulate strings by accessing specific characters, substrings, or ranges of characters. In this response, we'll explore the basics of string indexing and slicing, including common use cases and best practices.
String Indexing:
In Python, a string is an immutable sequence of characters. When working with strings, it's essential to understand how to access individual characters using indexing. String indexing in Python starts at 0, meaning the first character has an index of 0, the second character has an index of 1, and so on.
You can use square brackets []
to access a specific character by its index. For example:
my_string = "hello"
print(my_string[0]) # Output: 'h'
In this example, we're accessing the first character in the string "hello"
using my_string[0]
.
String Slicing:
Slicing is a powerful feature that allows you to extract a subset of characters from a string. You can specify a starting index (inclusive), an ending index (exclusive), and even step value to create a slice.
The basic syntax for slicing is:
my_string[start:end:step]
Here's a breakdown of the arguments:
start
: The starting index (inclusive) of the slice. Default is 0 if omitted.
end
: The ending index (exclusive) of the slice. Default is the length of the string if omitted.
step
: The step value for the slice. Default is 1 if omitted.
Let's create some examples to illustrate how slicing works:
my_string = "hello"
print(my_string[1:3]) # Output: 'el'
print(my_string[:3]) # Output: 'hel' (from start to index 2)
print(my_string[3:]) # Output: 'llo' (from index 3 to end)
print(my_string[::2]) # Output: 'hlo' (every other character starting from the beginning)
In this example, we're creating slices of different lengths and extracting specific substrings.
Common Use Cases:
Extracting a specific substring: Use slicing to extract a specific part of a string, such as a title or a summary. Converting strings to uppercase or lowercase: Use slicing with theupper()
or lower()
methods to convert a string to uppercase or lowercase. Removing unwanted characters: Use slicing to remove specific characters from a string, such as whitespace or punctuation marks.
Best Practices:
Use descriptive variable names: Name your strings and variables in a way that reflects their purpose and content. Test your code thoroughly: Verify the output of your slicing operations by printing the results to ensure you're getting the expected outcome. Use string methods judiciously: Be mindful of the methods available for strings, such asstrip()
, replace()
, and split()
.
In conclusion, Python's string indexing and slicing features offer powerful ways to manipulate strings. By mastering these techniques, you'll be able to extract specific substrings, convert strings, remove unwanted characters, and more!