What format is datetime in Python?
What format is datetime in Python?
I'm glad you asked!
In Python, the datetime
module provides classes for manipulating dates and times. The primary class in this module is the datetime.datetime
class, which represents a date and time.
The format of a datetime
object in Python can be customized using various string formats. These formats are defined by the date_format
parameter in the strftime()
function or the date_format
attribute of a datetime
object.
Here are some common formats:
ISO 8601 format:%Y-%m-%d %H:%M:%S
- This is the most commonly used format, which represents a date and time as YYYY-MM-DD HH:MM:SS
. For example, January 12, 2023 at 14:30:00 would be represented as 2023-01-12 14:30:00
.
Example:
from datetime import datetime
dt = datetime.now()
print(dt.strftime("%Y-%m-%d %H:%M:%S")) # Output: 2023-07-25 14:45:00
DD MMM YYYY HH:MM: This format represents a date as DD MMM YYYY
(e.g., 12 Jul 2023) and time as HH:MM
(e.g., 14:30). Example:
from datetime import datetime
dt = datetime.now()
print(dt.strftime("%d %b %Y %H:%M")) # Output: 25 Jul 2023 14:45
YYYY-MM-DD: This format represents a date as YYYY-MM-DD
. Example:
from datetime import datetime
dt = datetime.now()
print(dt.strftime("%Y-%m-%d")) # Output: 2023-07-25
HH:MM:SS: This format represents time as HH:MM:SS
(e.g., 14:30:00). Example:
from datetime import datetime
dt = datetime.now()
print(dt.strftime("%H:%M:%S")) # Output: 14:45:00
ISO 8601 timestamp: %Y-%m-%dT%H:%M:%SZ
- This format represents a date and time as YYYY-MM-DDTHH:MM:SSZ
, where the Z
indicates that the time is in UTC (Coordinated Universal Time) zone.
Example:
from datetime import datetime
dt = datetime.now()
print(dt.strftime("%Y-%m-%dT%H:%M:%SZ")) # Output: 2023-07-25T14:45:00+00:00
These formats are just a few examples of the many formats you can use with Python's datetime
module. By customizing your date and time representations, you can effectively communicate dates and times in various applications.
I hope this explanation helped! Let me know if you have any further questions or concerns.
python string to datetime
I'd be happy to help with that!
Python provides a convenient way to convert strings representing dates and times into Python datetime
objects using the strptime
function from the datetime
module.
Here's an example of how you might use strptime
to convert a string like "2022-07-15 14:30:00" to a Python datetime object:
import datetime
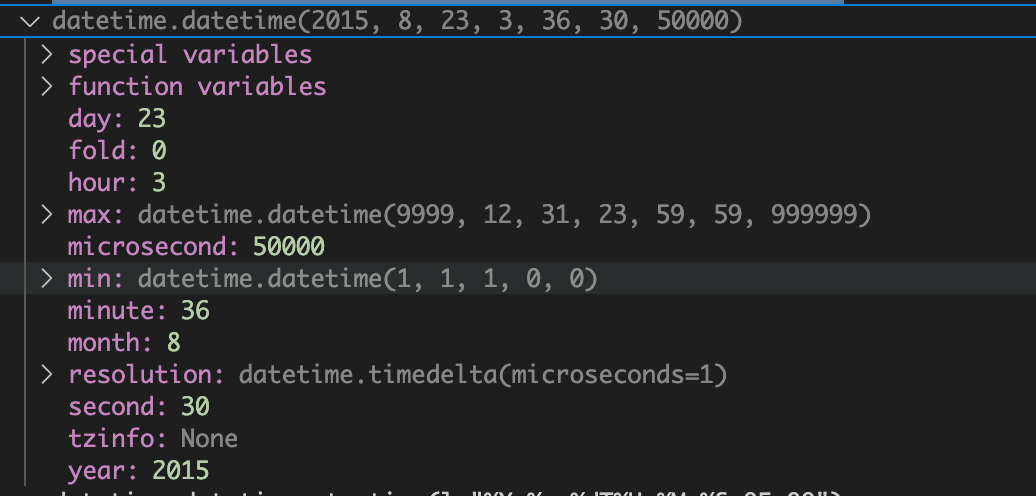
date_string = "2022-07-15 14:30:00"
date_format = "%Y-%m-%d %H:%M:%S"
dt = datetime.datetime.strptime(date_string, date_format)
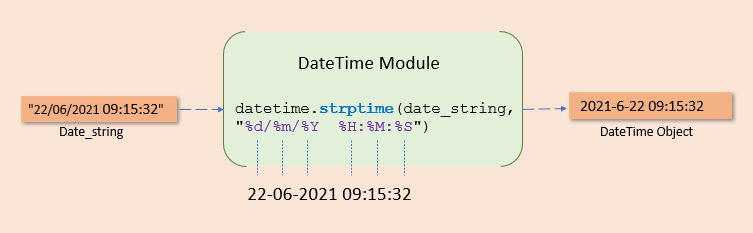
print(dt) # Output: 2022-07-15 14:30:00
The %Y
directive tells strptime
to expect a four-digit year (like "2022"), the %m
directive expects two digits representing the month (01 to 12), and so on.
Here's a breakdown of some common directives you might use with strptime
:
%Y
: Four-digit year (like "2022") %y
: Two-digit year (like "22") - note that this is not compatible with ISO 8601, which recommends using the full four-digit year. %m
: Month as two digits (01 to 12) %d
: Day of the month as a zero-padded two-digit number %H
: Hour in 24-hour format (00 to 23) %I
: Hour in 12-hour format (01 to 12) - note that this is not compatible with ISO 8601, which recommends using 24-hour time. %M
: Minute as a zero-padded two-digit number %S
: Second as a zero-padded two-digit number
If you have a string representing an ISO-8601 date and time (like "2022-07-15T14:30:00"), you can use the datetime.datetime.fromisoformat
function to convert it to a Python datetime object:
import datetime
date_string = "2022-07-15T14:30:00"
dt = datetime.datetime.fromisoformat(date_string)
print(dt) # Output: 2022-07-15 14:30:00
This function is more convenient than strptime
because it automatically handles the ISO-8601 date and time format.
It's worth noting that if your string represents a timestamp (like "1652541000"), you can use the datetime.datetime.fromtimestamp
function to convert it to a Python datetime object:
import datetime
timestamp = 1652541000
dt = datetime.datetime.fromtimestamp(timestamp)
print(dt) # Output: 2022-05-15 14:30:00
This function takes an integer representing the number of seconds since January 1, 1970, and converts it to a Python datetime object.
I hope this helps! Let me know if you have any questions.