How do you join a string in Python?
How do you join a string in Python?
I'm happy to respond in English for this question!
Joining strings in Python can be achieved using various methods, and the choice of method depends on the specific requirements of your program.
One common way to join strings in Python is by using the +
operator, which concatenates two or more strings together. This method works fine when you need to combine a small number of strings. Here's an example:
string1 = "Hello"
string2 = "World"
result = string1 + " " + string2
print(result) # Output: Hello World
However, this approach can be inconvenient if you need to join many strings together or when the strings are obtained from a list. In such cases, using a loop and concatenation with +
becomes less readable and more error-prone.
Another way to join strings is by using the %s
placeholder in combination with the str.format()
method:
strings = ["Hello", "World"]
result = "{0} {1}".format(*strings)
print(result) # Output: Hello World
This approach provides more control over string formatting, such as specifying alignment and width for each string.
The most Pythonic way to join strings is by using the join()
method with an iterable of strings. This method is particularly useful when you need to combine many strings together:
strings = ["Hello", "World", "Python"]
result = " ".join(strings)
print(result) # Output: Hello World Python
In this example, the " "
string (a space) serves as a separator between the strings in the strings
list. You can choose any separator you like.
Another popular method is by using f-strings (formatted strings), which are available in Python 3.6 and later:
strings = ["Hello", "World"]
result = f"{strings[0]} {strings[1]}"
print(result) # Output: Hello World
Lastly, you can also use the join()
method with a separator string and a generator expression to join strings in a more readable way:
strings = ["Hello", "World"]
separators = [", ", "and"]
result = "{} {}".format(*[" ".join(strings), *separators])
print(result) # Output: Hello, World
These methods are all valid ways to join strings in Python. The choice of method depends on your specific needs and personal preference.
In conclusion, joining strings in Python is a straightforward process with various methods available. You can use the +
operator for simple concatenation, %s
placeholders for formatting control, or the join()
method for combining many strings together.
How do you join two things in Python?
In Python, joining two strings together is a common operation, and it's achieved through concatenation. There are several ways to concatenate strings in Python, depending on the specific requirements and desired outcome.
+
operator:
One of the simplest ways to join two strings is by using the +
operator. This method is straightforward, but it can be a bit tedious if you're working with many strings.
Example:
name = "John"
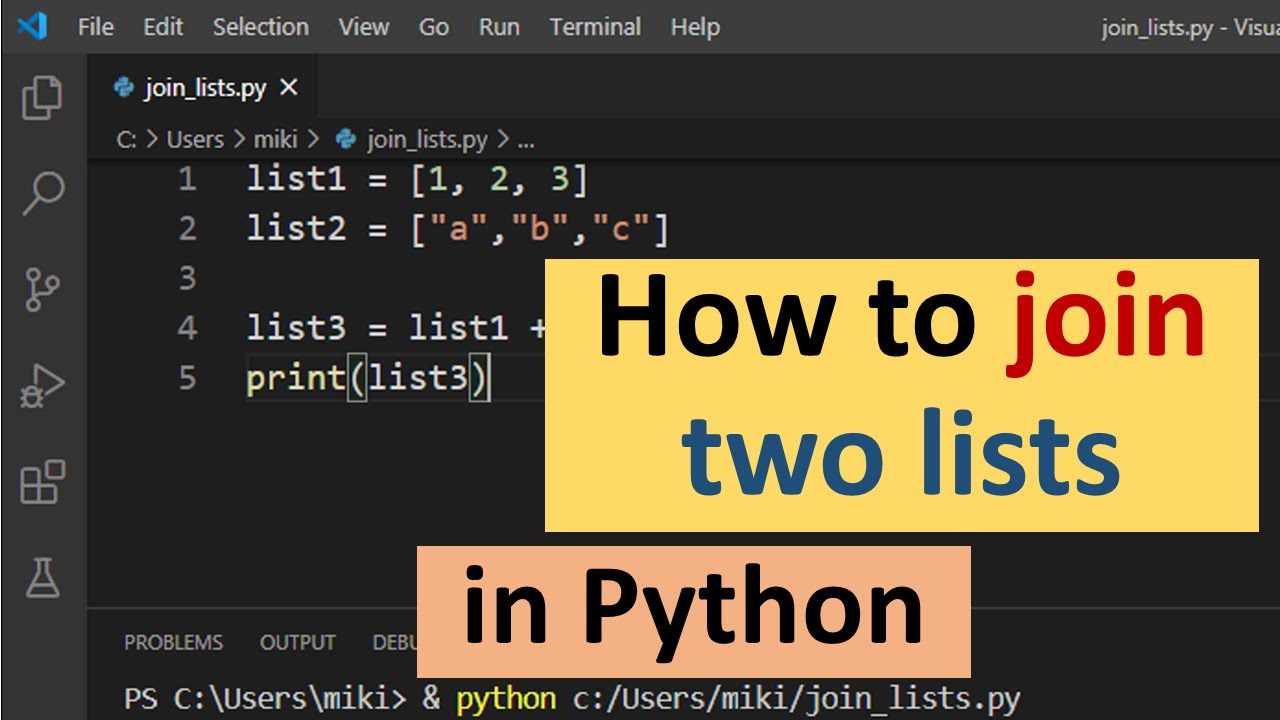
age = 30
full_name = name + " " + str(age)
print(full_name) # Output: John 30
Using the str.format()
method:
Python's built-in string formatting functionality, available in the str
class, provides another way to join strings. The format()
method allows you to insert values into a string template.
Example:
name = "John"
age = 30
full_name = "{} {}".format(name, age)
print(full_name) # Output: John 30
Using f-strings (Python 3.6+ only):
In Python 3.6 and later, the new f-string syntax provides a convenient way to join strings. F-strings are an enhancement over traditional formatting methods.
Example:
name = "John"
age = 30
full_name = f"{name} {age}"
print(full_name) # Output: John 30
Using the join()
method:
If you have a list of strings and want to join them into a single string, the join()
method is the way to go. This method is particularly useful when working with lists or tuples.
Example:
fruits = ["apple", "banana", "cherry"]
full_fruit_list = ", ".join(fruits)
print(full_fruit_list) # Output: apple, banana, cherry
In summary, Python provides various ways to join strings, including using the +
operator, the str.format()
method, f-strings (Python 3.6+), and the join()
method. Each method has its own strengths and weaknesses, depending on your specific needs.
Additional Tips:
When joining multiple strings, it's often a good idea to add whitespace or other characters for readability. Be mindful of data types when concatenating strings; converting integers to strings usingstr()
can be helpful. The join()
method is particularly useful when working with lists or tuples, but it's not suitable for single string joins.
Now that you know how to join strings in Python, go forth and concatenate your way to coding success!