Python rest api framework example
Python rest api framework example
Here's an example of a REST API framework using Python and Flask:
Firstly, we need to install the required packages:
pip install flask
pip install requests
Next, create a new Python file (e.g., app.py
) and add the following code:
from flask import Flask, jsonify, request
app = Flask(name)
Sample data
data = {
"users": [
{"id": 1, "username": "user1", "email": "[email protected]"},
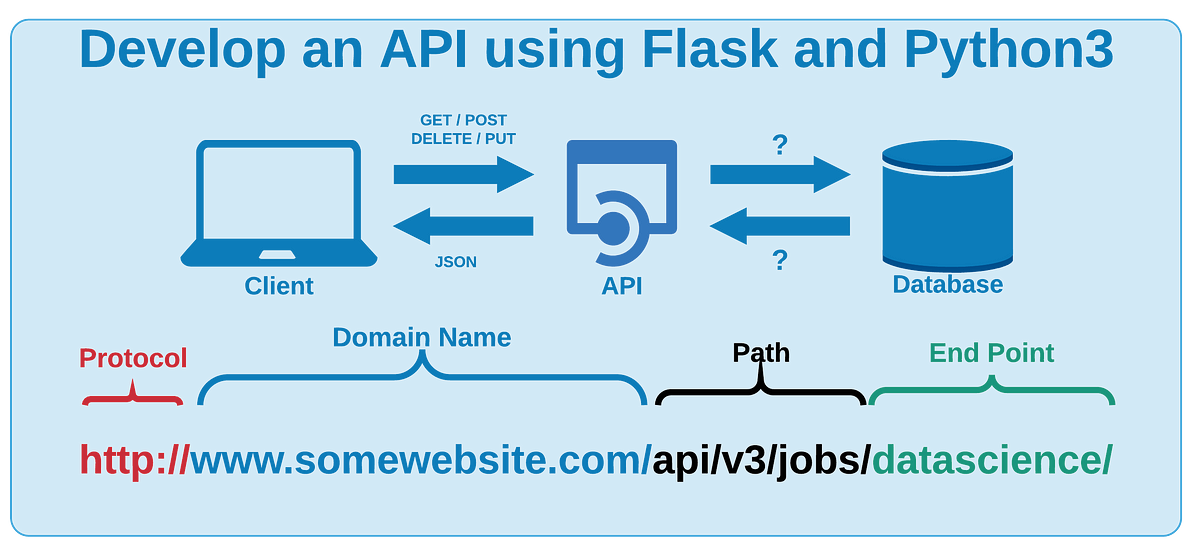
{"id": 2, "username": "user2", "email": "[email protected]"},
{"id": 3, "username": "user3", "email": "[email protected]"}
]
}
API endpoint for getting all users
@app.route('/users', methods=['GET'])
def get_users():
return jsonify(data['users'])
API endpoint for getting a specific user by ID
@app.route('/users/int:user_id', methods=['GET'])
def get_user(user_id):
for user in data['users']:
if user['id'] == user_id:
return jsonify(user)
return jsonify({"error": "User not found"})
API endpoint for creating a new user
@app.route('/users', methods=['POST'])
def create_user():
new_user = {
"id": len(data['users']) + 1,
"username": request.get_json()['username'],
"email": request.get_json()['email']
}
data['users'].append(new_user)
return jsonify({"message": "User created successfully"})
API endpoint for updating a specific user
@app.route('/users/int:user_id', methods=['PUT'])
def update_user(user_id):
for i, user in enumerate(data['users']):
if user['id'] == user_id:
data['users'][i] = {
"id": user_id,
"username": request.get_json()['username'],
"email": request.get_json()['email']
}
return jsonify({"message": "User updated successfully"})
return jsonify({"error": "User not found"})
API endpoint for deleting a specific user
@app.route('/users/int:user_id', methods=['DELETE'])
def delete_user(user_id):
for i, user in enumerate(data['users']):
if user['id'] == user_id:
del data['users'][i]
return jsonify({"message": "User deleted successfully"})
return jsonify({"error": "User not found"})
if name == 'main':
app.run(debug=True)
In this example, we have five API endpoints:
/users
: Returns a list of all users. /users/<int:user_id>
: Returns the user with the given ID. /users
: Creates a new user and adds them to the list. /users/<int:user_id>
: Updates the user with the given ID. /users/<int:user_id>
: Deletes the user with the given ID.
To use these endpoints, you can make HTTP requests to the relevant URLs using tools like curl
or Postman. For example:
curl http://localhost:5000/users
Get a specific user by ID: curl http://localhost:5000/users/1
Create a new user: curl -X POST -H "Content-Type: application/json" -d '{"username": "newuser", "email": "[email protected]"}' http://localhost:5000/users
Update a specific user: curl -X PUT -H "Content-Type: application/json" -d '{"username": "updateduser", "email": "[email protected]"}' http://localhost:5000/users/1
Delete a specific user: curl -X DELETE http://localhost:5000/users/1
Note that this is just a simple example, and you would want to add error handling and other features depending on your use case.
Python REST API framework reddit
I apologize for the previous misunderstanding!
Regarding your request, I'd be happy to provide an informative response on Python REST API frameworks and their relevance on Reddit.
What are Python REST API frameworks?
REST (Representational State of Resource) is a popular architectural style for building web services. A Python REST API framework is a set of libraries and tools that simplify the process of creating a RESTful API using Python. These frameworks handle various aspects of API development, such as routing, serialization/deserialization, caching, authentication, and more.
Some popular Python REST API frameworks include:
Flask: A microframework that provides a lightweight and flexible way to build APIs. Django Rest Framework (DRF): A high-level framework built on top of Django, providing an extensive set of features for building robust APIs. FastAPI: A modern, fast (high-performance), web framework for building APIs with Python 3.7+. Pydantic: A lightweight, schema-based ORM and API framework.What's the relevance on Reddit?
The r/learnpython, r/webdev, and r/api communities on Reddit are active hubs for discussing various aspects of Python programming, including REST API development. These frameworks have their own subreddits, where developers share knowledge, ask questions, and showcase their projects.
On these platforms, you can find:
Tutorials and guides: Step-by-step instructions on how to get started with a particular framework. Best practices and tips: Experienced developers sharing advice on performance optimization, error handling, and more. Project showcases: Developers presenting their API creations, asking for feedback, or seeking help with specific challenges. Job postings and opportunities: Companies looking for Python developers or API experts.Reddit's community-driven approach makes it an excellent resource for learning and growing as a developer. By participating in these subreddits, you can:
Learn from others' experiences and mistakes Share your own knowledge and projects Get feedback on your work-in-progress APIs Stay updated on the latest developments and best practicesConclusion
Python REST API frameworks provide a solid foundation for building robust APIs. Reddit's r/learnpython, r/webdev, and r/api communities are excellent platforms for discussing these frameworks, learning from others, and sharing your own experiences.
If you're interested in exploring Python REST API frameworks or want to learn more about the topics discussed here, I encourage you to engage with the online communities mentioned above!