How to write multiple lines in Python without running?
How to write multiple lines in Python without running?
In Python, there are several ways to achieve multi-line execution without the need for semicolons. Here are a few approaches:
1. Using parentheses
One way is by wrapping the expressions or statements you want to execute separately within parentheses:
(x + y;
print("Hello");
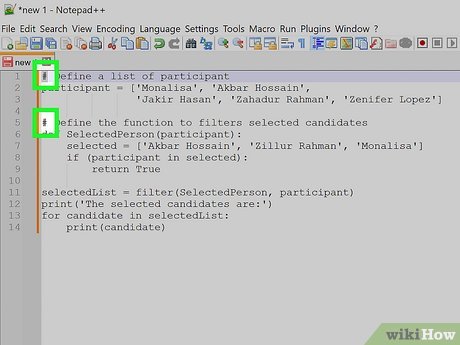
z - 10)
In this case, Python executes each expression or statement within the parentheses, but it doesn't treat them as a single line.
2. Using an if-else statement
Another way is by using an if-else
statement to separate your multi-line code:
if True:
(x + y
print("Hello")
z - 10)
This line will not run
else:
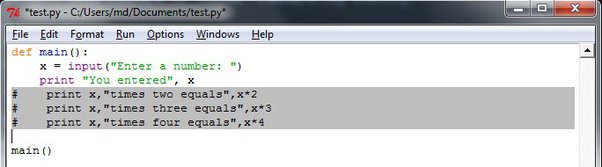
x - 5
In this example, only the expressions or statements within the if
block are executed. The else
block won't be reached.
3. Using a while loop
You can also use a while
loop to achieve multi-line execution:
i = 0
while i < 2:
(x + y
print("Hello")
z - 10)
i += 1
Here, the code within the while
loop is executed twice, but each iteration runs separately.
4. Using a function or class
In Python, functions and classes can be used as blocks to group multiple statements together:
def my_func():
(x + y
print("Hello")
z - 10)
x = 5
my_func()
Or:
class MyClass:
def init(self):
(x + y
print("Hello")
z - 10)
obj = MyClass()
In these examples, the code within the function or class is executed as a block, without treating each statement as separate lines.
5. Using triple quotes
Lastly, you can use triple quotes to define a multi-line string:
my_string = """
(x + y
print("Hello")
z - 10)
"""
print(my_string)
In this case, the triple-quoted string contains multiple lines of code, but it won't be executed as Python code. Instead, it will be treated as a literal string.
These are just a few examples of how you can write multi-line code in Python without running into issues with semicolons. Each approach has its own use cases and trade-offs, so choose the one that best fits your coding style!
Python write multiple lines to file for loop
I'd be happy to help!
Here's an example of how you can use a for loop in Python to write multiple lines to a file:
# Open the file in write mode
with open("example.txt", "w") as file:
Define a list of strings that will be written to the file
lines = ["Line 1: This is the first line.",
"Line 2: This is the second line, and it's a bit longer than the first one.",
"Line 3: This is the third line, and it's getting even longer!",
"Line 4: This is the fourth line, and it's almost as long as the third one!"]
Use a for loop to write each line to the file
for i, line in enumerate(lines):
Write the line to the file
file.write(line)
Add a newline character at the end of each line
file.write("n")
print("File written successfully!")
When you run this code, it will create a new file called "example.txt" and write the four lines from the lines
list to it. The file will look like this:
Line 1: This is the first line.
Line 2: This is the second line, and it's a bit longer than the first one.
Line 3: This is the third line, and it's getting even longer!
Line 4: This is the fourth line, and it's almost as long as the third one!
As you can see, each line has been written to the file with a newline character at the end. This means that when you open the file in a text editor or display it on screen, each line will start on its own line.
Note that I used the with
statement to open the file, which ensures that the file is properly closed even if an error occurs. This is good practice whenever you're working with files, as it helps prevent errors and makes your code more robust.
Also, I used the enumerate
function in the for loop to get both the index and value of each line. This can be useful if you want to do something different based on the position of the line or some other factor.
I hope this helps! Let me know if you have any questions or need further clarification.