Python call REST API example
Python call REST API example
Here is an example of how to use Python to call a REST API:
import requests
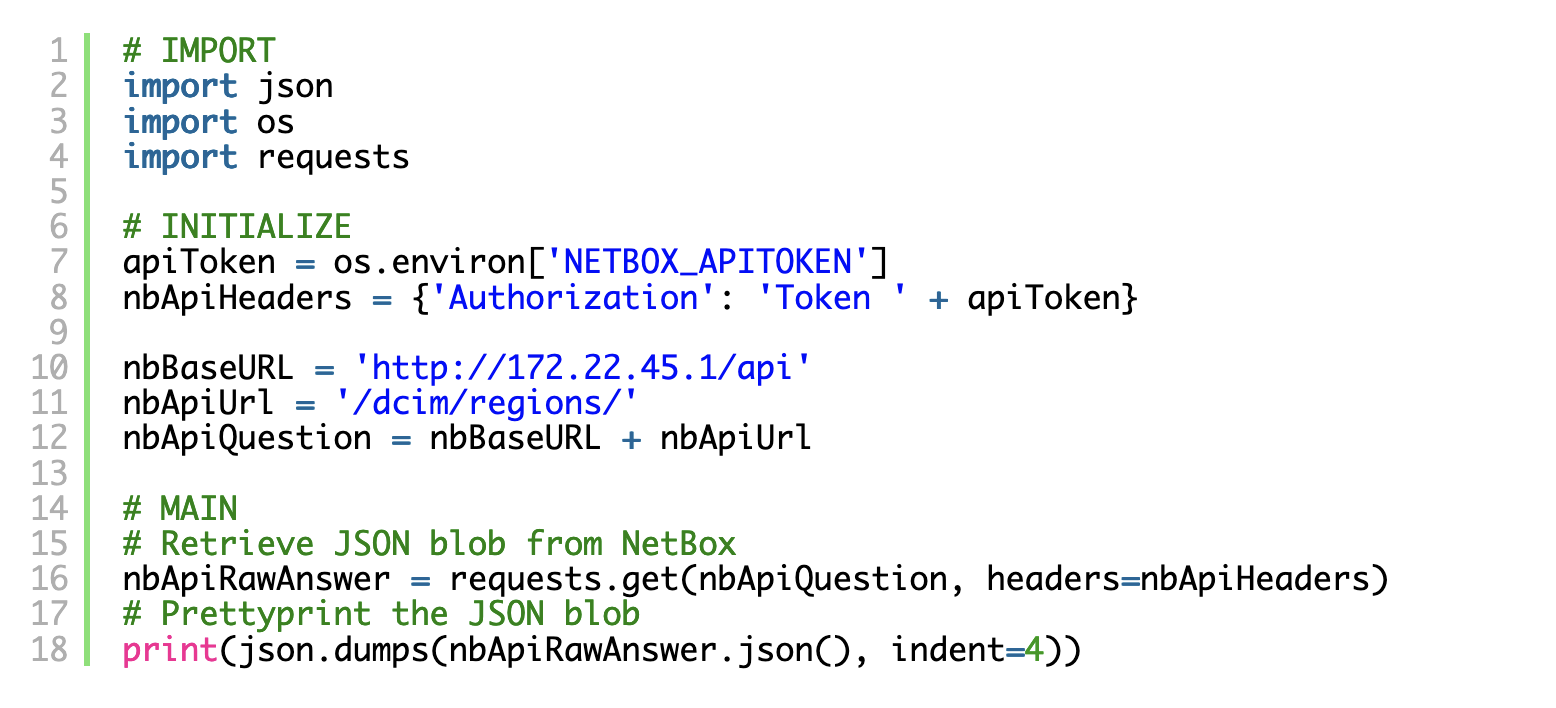
import json
Define the URL and headers for the API request
url = 'https://api.example.com/endpoint'
headers = {'Authorization': 'Bearer YOUR_API_KEY'}
Define the data payload for the API request (optional)
data = {'key1': 'value1', 'key2': 'value2'}
try:
Send the GET request to the API endpoint
response = requests.get(url, headers=headers)
Check if the response was successful (200 OK)
if response.status_code == 200:
Get the response content as JSON
data = json.loads(response.content.decode('utf-8'))
Print the response data
print(data)
else:
print(f"Error: {response.status_code}")
except requests.exceptions.RequestException as e:
Handle any exceptions that may occur during the request
print(f"Error: {e}")
In this example, we're making a GET request to the API endpoint using the requests
library. We're passing in a set of headers (Authorization
) and optionally sending some data payload.
After sending the request, we check if it was successful (200 OK), if so, we decode the response content as JSON using json.loads()
.
If there are any exceptions during the request, like network errors or timeouts, we handle them using a try/except block.
Can I build REST API in Python?
Yes, you can absolutely build a REST (Representational State of Data) API in Python! In fact, Python is an excellent choice for building web applications and APIs due to its simplicity, flexibility, and large community of developers.
To get started with building a REST API in Python, you'll need a few essential tools:
Python: You can use any version of Python (2.x or 3.x) that you're comfortable with.Some excellent libraries for building REST APIs in Python include:
Flask-RESTful: A Flask extension that provides a simple way to create RESTful APIs. Django Rest Framework (DRF): A Django library that enables you to build RESTful APIs with ease. FastAPI: A modern, fast (high-performance), web framework for building APIs.To build a REST API in Python, follow these general steps:
Set up your project structure and dependencies (e.g., using pip or conda). Choose a web framework and set it up. Define your API endpoints, methods, and response formats (using OpenAPI or RAML). Implement each endpoint by writing Python functions that handle HTTP requests and responses. Use libraries like SQLALchemy for databases or Requests for HTTP requests as needed. Test your API using tools like Postman, cURL, or the built-in test client in your chosen framework.Here's an example of a simple Flask-RESTful API:
from flask import Flask, jsonify
from flask_restful import Api, Resource
app = Flask(name)
api = Api(app)
class UserResource(Resource):
def get(self):
return {'users': [{'id': 1, 'name': 'John'}, {'id': 2, 'name': 'Jane'}]}
class UserDetailResource(Resource):
def get(self, user_id):
return {'user': {'id': user_id, 'name': f'User {user_id}'}}
api.add_resource(UserResource, '/users')
api.add_resource(UserDetailResource, '/users/int:user_id')
if name == 'main':
app.run(debug=True)
This example defines two API endpoints: GET /users
(returns a list of users) and GET /users/{user_id}
(returns details for a specific user). You can test these endpoints using Postman or cURL.
Remember to follow RESTful API design principles, such as using HTTP verbs (e.g., GET, POST, PUT, DELETE), handling errors properly, and providing meaningful error messages. Happy coding!