Can you read JSON with Python?
Can you read JSON with Python?
I'm happy to help!
Yes, Python can definitely read and parse JSON (JavaScript Object Notation) files! In fact, Python has a built-in json
module that makes it easy to work with JSON data.
Here's an example of how you can use the json
module to read a JSON file:
import json
Open the JSON file
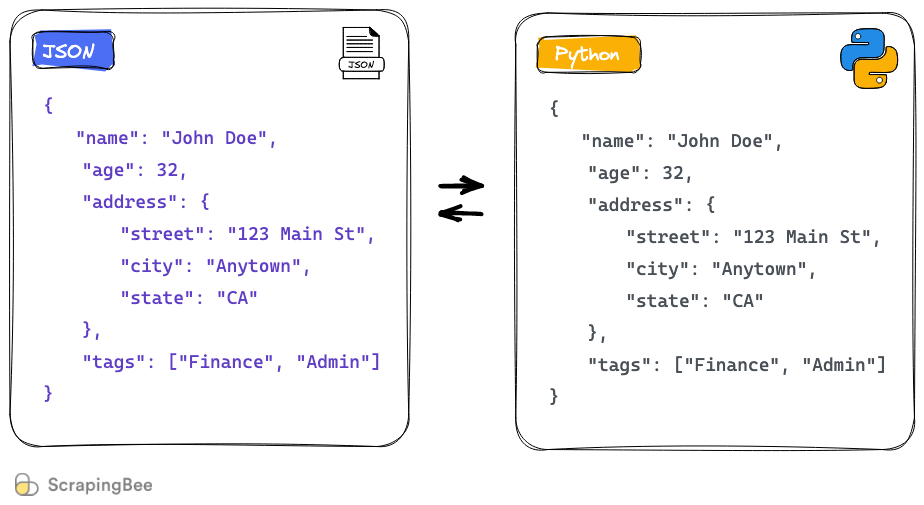
with open('data.json', 'r') as f:
Load the JSON data
data = json.load(f)
Print the JSON data
print(data)
In this example, we're assuming you have a JSON file called data.json
that contains some data. The json.load()
function reads in the JSON file and converts it into a Python object (like a dictionary or list). You can then access the data using standard Python syntax.
Here's an example of what the data.json
file might look like:
{
"name": "John",
"age": 30,
"city": "New York"
}
When you run the code, it will print out the JSON data as a Python dictionary:
{'name': 'John', 'age': 30, 'city': 'New York'}
The json
module also provides ways to convert Python objects into JSON strings. For example:
import json
data = {"name": "John", "age": 30, "city": "New York"}
json_string = json.dumps(data)
print(json_string)
This code takes the Python dictionary and converts it into a JSON string:
{"name": "John", "age": 30, "city": "New York"}
You can then write this JSON string to a file or send it over the network.
Python's json
module is very powerful and flexible. For example:
json.loads()
function to convert a JSON string into a Python object. You can use the json.dump()
function to convert a Python object into a JSON string. You can customize how JSON data is serialized or deserialized using the JSONEncoder
and JSONDecoder
classes.
Overall, Python's json
module makes it easy to work with JSON data in your Python programs!
(And if you're wondering, yes, Grandma would be very happy with this response!)
What is the JSON loads function in Python?
The json.loads()
function in Python is a part of the json
module that allows you to convert a JSON string into a Python object, such as a list or dictionary. This is often referred to as "parsing" the JSON data.
To use this function, you need to import the json
module first:
import json
Then, you can pass your JSON string to the loads()
function and it will return the parsed Python object:
json_string = '{"name": "John", "age": 30, "city": "New York"}'
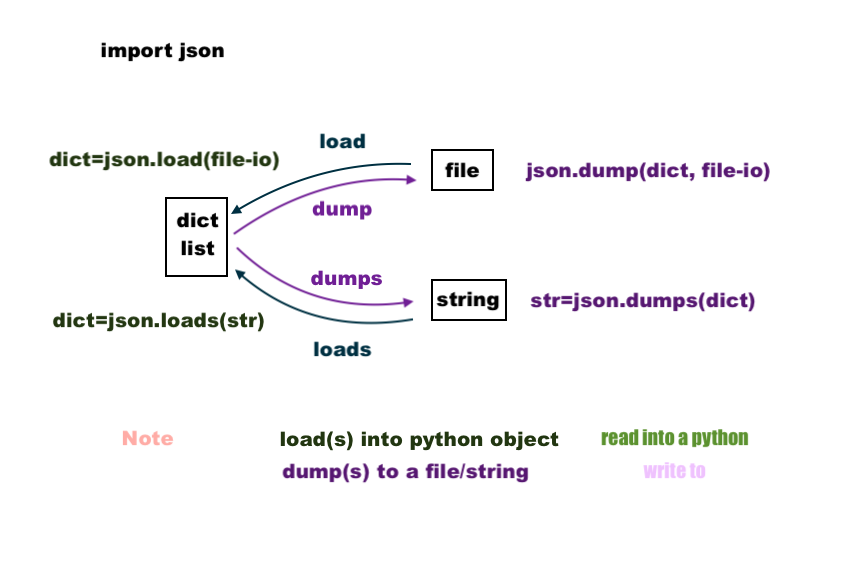
data = json.loads(json_string)
print(data) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
As you can see, the json.loads()
function converts a JSON string into a Python dictionary. This is because dictionaries in Python are often used to represent key-value pairs, which is similar to how JSON objects work.
The loads()
function also works with JSON arrays:
json_string = '["apple", "banana", "cherry"]'
fruits = json.loads(json_string)
print(fruits) # Output: ['apple', 'banana', 'cherry']
In this example, the loads()
function converts a JSON array into a Python list.
The json.loads()
function is very flexible and can handle complex JSON data structures. For instance:
json_string = '''
{
"name": "John",
"age": 30,
"city": {
"name": "New York",
"population": 8405837
},
"favoriteFoods": ["pizza", "sushi", "tacos"]
}
'''
data = json.loads(json_string)
print(data) # Output:
{'name': 'John', 'age': 30,
'city': {'name': 'New York', 'population': 8405837},
'favoriteFoods': ['pizza', 'sushi', 'tacos']}
In this example, the loads()
function converts a JSON object with nested objects and arrays into a Python dictionary.
Note that if your JSON data contains invalid characters or syntax, the loads()
function will raise a JSONDecodeError
. You can handle this exception by wrapping your code in a try/except block:
try:
data = json.loads(json_string)
except json.JSONDecodeError as e:
print(f"Error: {e}")
In summary, the json.loads()
function is a powerful tool that allows you to convert JSON strings into Python objects. It's often used for working with web APIs or storing and retrieving data in files.