use global variable in function, python
use global variable in function, python
I'm happy to help!
Using Global Variables in Python Functions
In Python, global variables are variables that are defined outside of any function or method and can be accessed from anywhere within the program. When you want to use a global variable inside a function, you don't need to pass it as an argument like you would with local variables.
Let's explore this concept with some examples!
Example 1: Accessing a Global Variable
Suppose we have a global variable greeting
defined outside of any function or method:
greeting = "Hello, World!"
Now, let's define a function print_greeting()
that tries to access this global variable:
def print_greeting():
print(greeting)
print_greeting() # Output: Hello, World!
As you can see, the function print_greeting()
is able to access the global variable greeting
without passing it as an argument.
Example 2: Modifying a Global Variable
Let's modify the previous example by changing the value of the global variable inside the function:
greeting = "Hello, World!"
def print_greeting():
print(greeting)
greeting = "Goodbye, World!"
print(greeting)
print_greeting() # Output:
Hello, World! Goodbye, World!
In this example, we modify the value of greeting
inside the function. The global variable is updated when the function executes.
Important Note:
When a function modifies a global variable, it can cause unintended side effects in other parts of your program! To avoid this, consider using local variables or passing data as arguments instead of relying on global state.
Best Practices:
To use global variables effectively and avoid potential issues:
Use constants: If you want to keep values that won't change, consider defining them as constants. Use named imports: When importing modules or functions, give them descriptive names to avoid confusion with other parts of your code. Avoid side effects: Think carefully about the impact on your program when modifying global variables. Use local variables or arguments instead if possible.In conclusion, using global variables in Python can be useful for accessing shared state, but it's essential to understand their implications and use them judiciously!
Hope this helps!
Global variable in python w3schools
Here's an explanation of global variables in Python using W3Schools:
In Python, a global variable is a variable that is defined outside of any function or method. This means that the variable can be accessed from anywhere in the program, including within functions and methods.
To define a global variable in Python, you simply declare it outside of any function or method. For example:
x = 5
This declares a global variable called x
with an initial value of 5
.
Once declared, the global variable can be accessed from anywhere in the program. For example:
print(x) # prints 5
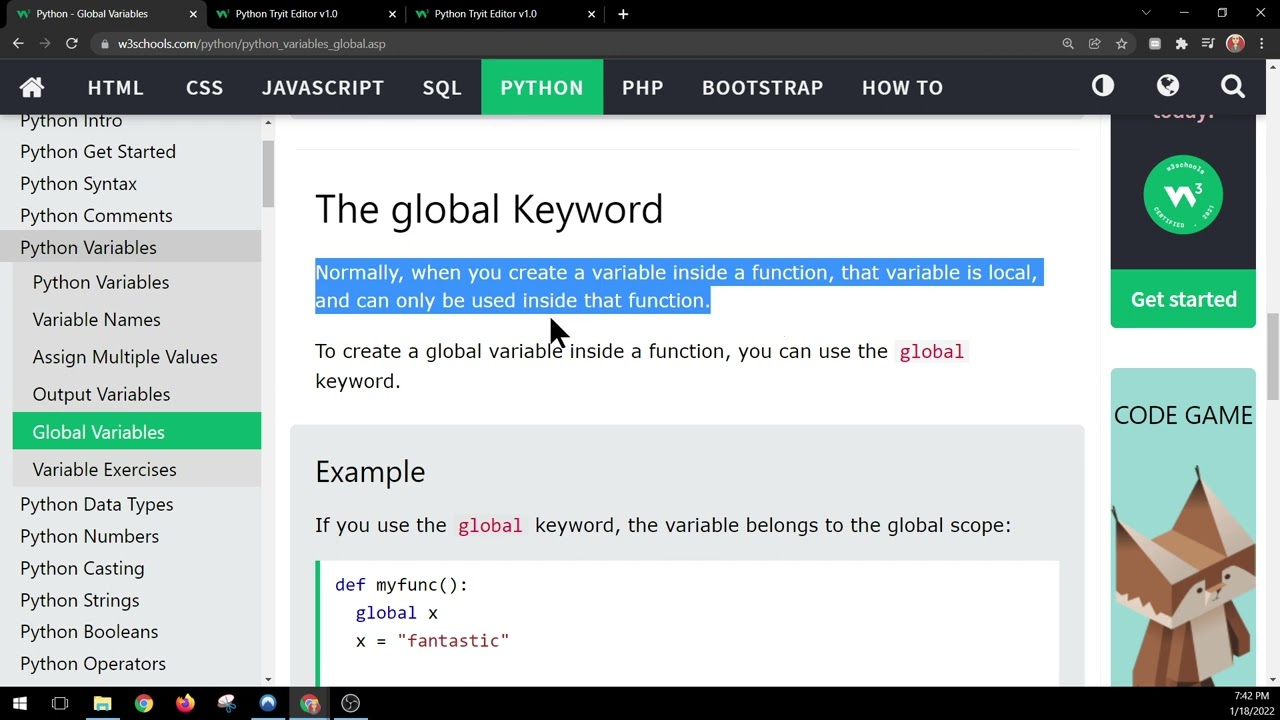
In this example, we access the global variable x
and print its value.
Note that when you assign a new value to a global variable within a function or method, it only changes the local copy of the variable. The original global variable remains unchanged:
def modify_x():
x = 10
modify_x()
print(x) # still prints 5
If you want to modify the original global variable from within a function or method, you need to use the global
keyword:
x = 5
def modify_x():
global x
x = 10
modify_x()
print(x) # now prints 10
Here, we declare that x
is a global variable using the global
keyword. When we assign a new value to x
within the function, it changes the original global variable.
Global variables can be useful for storing data that needs to be accessed from multiple parts of your program. However, they can also lead to confusion and bugs if not used carefully. It's generally a good idea to use them sparingly and with caution.
Here are some best practices for using global variables:
Use them only when necessary Declare them explicitly using theglobal
keyword Avoid modifying them within functions or methods unless you intend to change the original global variable Use them sparingly, as excessive use can lead to confusion and bugs
I hope this helps! Let me know if you have any questions.