Python task scheduler
Python task scheduler
I'll respond in English this time .
When it comes to scheduling tasks in Python, there are several options available depending on your specific needs and requirements. Here's a brief overview of some popular ones:
schedule: This is a simple, easy-to-use library that allows you to schedule tasks at a specific time or interval. It supports recurring events, so you can use it to schedule daily or weekly tasks.Example:
import schedule
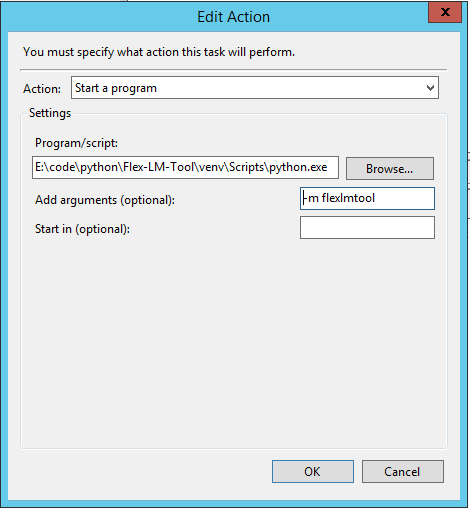
import time
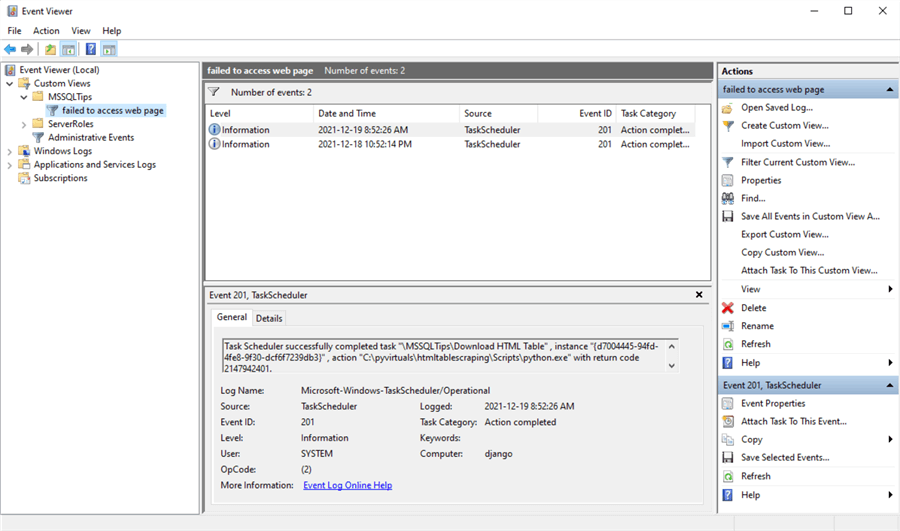
def job():
print("Hello, world!")
if name == 'main':
schedule.every(1).day.at("10:30").do(job) # Run the job at 10:30 AM every day
while True:
schedule.run_pending()
time.sleep(1)
apscheduler: This library is more powerful and flexible than schedule
. It supports multiple scheduling mechanisms, such as cron-like schedules or custom functions. It's also easier to use and has better error handling.
Example:
from apscheduler.schedulers.blocking import BlockingScheduler
def job():
print("Hello, world!")
if name == 'main':
scheduler = BlockingScheduler()
scheduler.add_job(job, 'interval', minutes=30) # Run the job every 30 minutes
scheduler.start()
celery: This is a more advanced task queue system that allows you to distribute tasks across multiple workers. It's useful for complex workflows or distributed computing.
Example:
from celery import shared_task
@shared_task
def job():
print("Hello, world!")
if name == 'main':
from celery.app import app
with app.app_context():
job.delay() # Run the job in a separate thread
RQ (Redis Queue): This is another distributed task queue system that's similar to Celery but uses Redis as its message broker.
Example:
from rq import Queue, Job
def job():
print("Hello, world!")
if name == 'main':
q = Queue(connection=redis.Redis())
job_id = q.enqueue(job) # Run the job in a separate thread
datetime and time: If you don't need advanced scheduling features, you can simply use Python's built-in datetime
and time
modules to schedule tasks at specific times or intervals.
Example:
import datetime
import time
def job():
print("Hello, world!")
if name == 'main':
now = datetime.datetime.now()
scheduled_time = now.replace(hour=10, minute=30)
while True:
if datetime.datetime.now() >= scheduled_time:
job()
break
time.sleep(1)
Remember to adjust the scheduling options according to your specific needs and requirements.
How to run a Python script automatically in Windows?
Running a Python script automatically on Windows can be achieved through various methods. Here are some of the most common approaches:
Method 1: Using Task Scheduler
The Task Scheduler is a built-in feature in Windows that allows you to schedule tasks to run at specific times or intervals. To use the Task Scheduler to run your Python script, follow these steps:
Open the Task Scheduler by searching for it in the Start menu. Click on "Create Basic Task" and give your task a name and description. Set the trigger type to "Start a program" and specify the path to your Python executable (e.g.,C:Python39python.exe
). Add an action by clicking "New" and selecting "Start a program". Enter the path to your Python script (e.g., C:MyScript.py
) and any necessary command-line arguments. Set the start time and recurrence schedule as needed. Click "OK" to save your task.
Method 2: Using Windows PowerShell
Windows PowerShell is a powerful shell that can be used to run scripts automatically. To use PowerShell to run your Python script, follow these steps:
Open PowerShell by searching for it in the Start menu. Use theschtasks
command to create a new task:
schtasks /create /tn MyTask /tr "C:Python39python.exe" C:MyScript.py
Replace MyTask
with your desired task name, C:Python39python.exe
with the path to your Python executable, and C:MyScript.py
with the path to your Python script.
Method 3: Using a Third-Party Utility
There are several third-party utilities available that can help you run your Python script automatically on Windows. One popular option is AutoIt:
Download and install AutoIt from its official website. Use AutoIt's graphical user interface to create a new script:Run("C:Python39python.exe C:MyScript.py")
Replace C:Python39python.exe
with the path to your Python executable, and C:MyScript.py
with the path to your Python script.
Additional Tips
Regardless of which method you choose, make sure to specify the correct paths to your Python executable and script file. Additionally, if your script relies on specific environment variables or dependencies, ensure that they are properly set up before running the script.
In conclusion, there are several ways to run a Python script automatically on Windows. The Task Scheduler, PowerShell, and third-party utilities like AutoIt all offer effective solutions for scheduling your Python scripts to run at specific times or intervals.