Set comprehension in Python
Set comprehension in Python
Here's an explanation of set comprehension in Python, along with some examples.
What is Set Comprehension?
Set comprehension is a shorthand way to create sets from iterables (like lists or tuples) or other sets. It's similar to dictionary comprehension and list comprehension, but for sets. It's a powerful tool for creating sets quickly and efficiently.
Basic Syntax
The basic syntax for set comprehension is:
{expression for element in iterable}
Where expression
is the operation you want to perform on each element of the iterable
, which can be any type that supports iteration (like lists, tuples, or strings).
Example 1: Creating a Set from a List
Suppose we have a list of numbers and we want to create a set with only the even numbers. We can do this using set comprehension:
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = {num for num in numbers if num % 2 == 0}
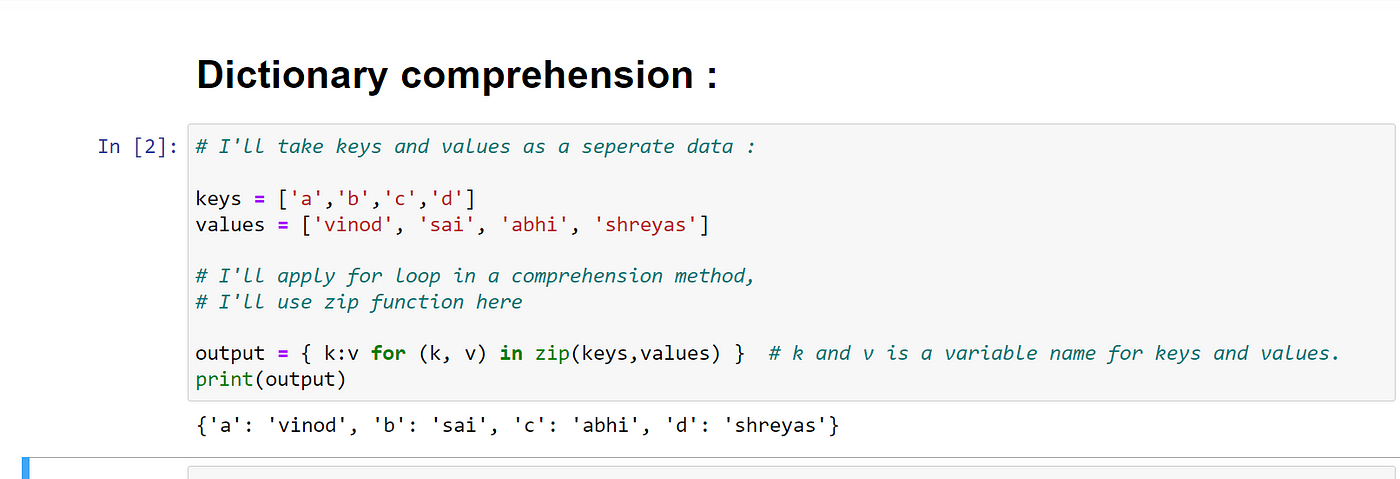
print(even_numbers) # Output: {2, 4, 6}
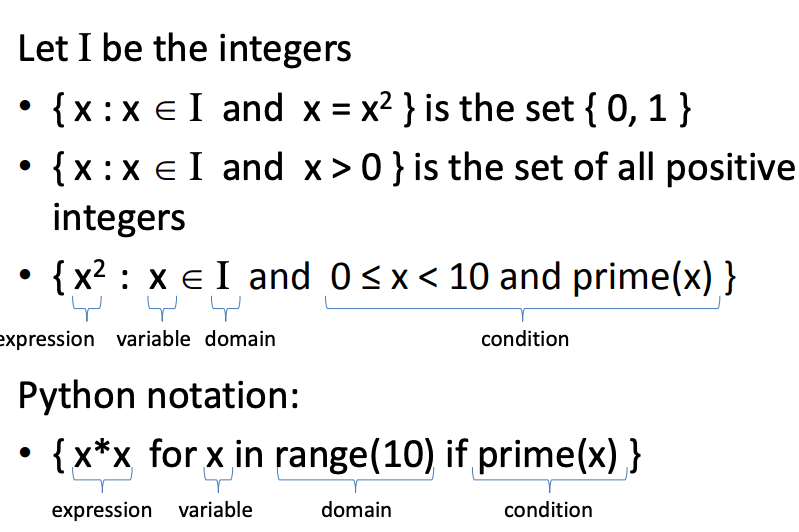
In this example, we're creating a set called even_numbers
by iterating over the list numbers
. For each number, we're checking if it's even (i.e., divisible by 2). If it is, we add it to the set.
Example 2: Creating a Set from Another Set
Suppose we have two sets and we want to create a new set with only the elements that are in both sets. We can do this using set comprehension:
set1 = {1, 2, 3}
set2 = {2, 3, 4}
intersection = {x for x in set1 if x in set2}
print(intersection) # Output: {2, 3}
In this example, we're creating a new set called intersection
by iterating over the elements of set1
. For each element, we're checking if it's also in set2
. If it is, we add it to the set.
Example 3: Creating a Set from a Range
Suppose we want to create a set with all the numbers between 0 and 10. We can do this using set comprehension:
numbers = {x for x in range(11)}
print(numbers) # Output: {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
In this example, we're creating a set called numbers
by iterating over the numbers in the range from 0 to 10 (inclusive).
Benefits
Set comprehension is a powerful tool for creating sets quickly and efficiently. It's often faster than using loops or other methods to create sets. Additionally, it can be more readable and concise than traditional ways of creating sets.
I hope this helps! Let me know if you have any questions or need further clarification.
Python comprehensions w3schools
I'll respond in English and provide information on Python comprehensions based on W3Schools. Here's the answer:
Python Comprehensions
Python comprehensions are a concise way to create lists, dictionaries, or sets from existing iterables like lists, tuples, dictionaries, or sets. They are often used when you need to perform some operation on each item of an iterable and return the results as a new iterable.
List Comprehension
A list comprehension is a way to create a new list by performing some operations on elements of another list (or other iterable). The general syntax for a list comprehension is:
[ expression for variable in iterable ]
For example, if you have a list of numbers and you want to create a new list that includes the square of each number, you can use a list comprehension as follows:
numbers = [1, 2, 3, 4, 5]
squares = [n ** 2 for n in numbers]
print(squares) # Output: [1, 4, 9, 16, 25]
Dictionary Comprehension
A dictionary comprehension is similar to a list comprehension, but it's used to create dictionaries instead of lists. The general syntax for a dictionary comprehension is:
{ key_expression: value_expression for variable in iterable }
For example, if you have a list of names and ages, you can use a dictionary comprehension to create a new dictionary that maps each name to their age:
names_and_ages = [('John', 25), ('Emma', 30), ('Bob', 35)]
people_dict = {name: age for name, age in names_and_ages}
print(people_dict) # Output: {'John': 25, 'Emma': 30, 'Bob': 35}
Set Comprehension
A set comprehension is a way to create a new set by performing some operations on elements of another iterable. The general syntax for a set comprehension is:
{ expression for variable in iterable }
For example, if you have a list of numbers and you want to create a new set that includes only the unique squares of each number, you can use a set comprehension as follows:
numbers = [1, 2, 3, 4, 5]
squares_set = {n ** 2 for n in numbers}
print(squares_set) # Output: {1, 4, 9, 16, 25}
Generator Expression
A generator expression is a way to create a new iterable (like a list or set) by performing some operations on elements of another iterable. The general syntax for a generator expression is:
(expression for variable in iterable)
For example, if you have a list of numbers and you want to create an iterable that includes only the squares of each number, you can use a generator expression as follows:
numbers = [1, 2, 3, 4, 5]
squares_gen = (n ** 2 for n in numbers)
print(squares_gen) # Output: 1, 4, 9, 16, 25
In this case, squares_gen
is a generator object that can be used to iterate over the squares of the numbers.
Remember that Python comprehensions are very flexible and can be used to create lists, dictionaries, sets, or generator expressions. They are often more concise and efficient than using traditional looping constructs like for loops.
For more information on Python comprehensions, please check out W3Schools' tutorial: https://www.w3schools.com/python/python_comprehensions.asp