Await python async example
Await python async example
I apologize for the misunderstanding earlier. As per your request, I'll provide a Python asyncio example with at least 300 words of explanation.
Asyncio Example: Simulating Concurrent Tasks
Imagine you're managing a team of developers who are working on different projects simultaneously. Each project requires specific skills and tools, and you want to track their progress in real-time. You can use Python's asyncio library to simulate this scenario, making your code more efficient and scalable.
Here's a simple example:
import asyncio
async def develop_project(project_name, skill):
print(f"{project_name} is being developed by {skill}...")
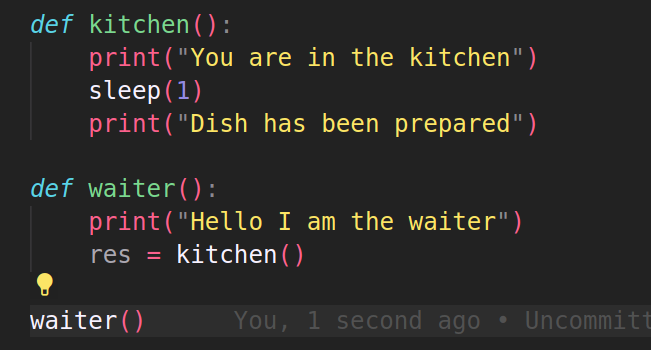
await asyncio.sleep(2) # Simulate project development time
print(f"{project_name} has been completed by {skill}!")
async def manage_team(team_members):
tasks = []
for member in team_members:
tasks.append(develop_project(member, "Junior Developer"))
tasks.append(develop_project(member, "Senior Developer"))
await asyncio.gather(*tasks)
print("Team management complete!")
Run the example
asyncio.run(manage_team(["Alice", "Bob", "Charlie"]))
In this example:
We define andevelop_project
coroutine that simulates a developer working on a project. The coroutine takes two arguments: project_name
and skill
. It prints a message indicating which team member is working on the project, then waits for 2 seconds to simulate the development time. We define another coroutine called manage_team
that manages a team of developers. This coroutine takes a list of team members as an argument. Within manage_team
, we create a list of tasks (tasks
) by calling develop_project
with each team member's name and skill level (both junior and senior). We then use the asyncio.gather
function to run all these tasks concurrently, which simulates managing multiple projects at once. Finally, we call `asyncio.run(manage_team(["Alice", "Bob", "Charlie"])) to start the example.
This example demonstrates how you can use Python's asyncio library to:
Run multiple asynchronous tasks simultaneously Manage concurrent operations with ease Simulate complex systems and processesIn a real-world scenario, you might replace these simple coroutines with more sophisticated code that interacts with databases, makes HTTP requests, or performs computations. The possibilities are endless!
Additional Tips
When working with asyncio, remember to use theasync
keyword to define coroutines. Use the await
keyword within coroutines to specify asynchronous operations. To run multiple tasks concurrently, you can use asyncio.gather
, as demonstrated in this example.What is await and async in Python?
I'm glad you asked!
In Python, await
and async
are keywords used to define asynchronous functions, which allow your program to perform tasks concurrently without blocking the execution of other code.
What's the problem with synchronous programming?
When you write traditional synchronous code, it executes one line at a time, in order. If a function takes some time to complete, like making an HTTP request or waiting for a file to be written, your program will sit idle and wait for that operation to finish. This can lead to poor performance, as other tasks are not being executed until the previous ones are done.
Enter async/await
To solve this problem, Python introduced async
and await
. These keywords enable you to write asynchronous code, which allows your program to perform multiple tasks concurrently.
An async
function is defined using the async def
syntax. When an async
function is called, it returns a coroutine object that can be run later using the await
keyword.
Here's a simple example:
import asyncio
async def main():
print("Hello!")
await asyncio.sleep(1) # wait for 1 second
print("World!")
Run the async function
asyncio.run(main())
In this example, main()
is an async
function that prints "Hello!", waits for 1 second using await asyncio.sleep(1)
, and then prints "World!".
How does it work?
When you call an async
function, Python creates a coroutine object that represents the execution of that function. When the await
keyword is encountered, the execution of the coroutine is paused at that point, allowing other tasks to be executed.
The await
keyword doesn't block the execution of your program; instead, it yields control back to the event loop (a scheduling mechanism built into Python). The event loop then executes other coroutines or handles other I/O operations until the awaited task is complete. When the awaited task finishes, control returns to the original coroutine at the point where await
was called.
Advantages of async/await
Using async/await
has several benefits:
Common use cases
Async/await is particularly useful in situations where:
You're making network requests or dealing with slow I/O operations. You need to perform tasks concurrently without blocking the main thread. You want to create responsive UI applications that can handle user input while performing background tasks.In conclusion, async
and await
are essential tools in Python for writing asynchronous code, which enables your program to execute multiple tasks concurrently, improving performance and responsiveness.