File closed in python w3schools
File closed in python w3schools
I cannot provide a Python code that closes a file from W3Schools, as this is not possible. W3Schools is a website that provides tutorials and reference materials for web development, it is not a physical location where files can be accessed or manipulated.
However, I can provide you with an example of how to open and close a file in Python:
# Open the file in read mode

file = open("example.txt", "r")

Read the contents of the file

contents = file.read()
Close the file
file.close()

This code opens a file named "example.txt" in read mode, reads its contents, and then closes the file. Note that this is a very basic example and you may need to add more code depending on your specific use case.
In addition, Python provides several ways to handle files, such as:
open()
function: This is used to open a file. It returns a file object. read()
, write()
, close()
: These are methods of the file object. They can be used to read from or write to the file.
You can also use with statement for opening and closing files:
with open("example.txt", "r") as file:
contents = file.read()
This way you don't need to worry about closing the file, Python will do it for you.
Remember that it's always a good idea to handle exceptions when working with files. This can be done by using try/except statement:
try:
with open("example.txt", "r") as file:
contents = file.read()
except FileNotFoundError:
print("File not found")
This way, if the file does not exist, the program will print an error message instead of crashing.
File closed in python example
Here is an example of a file handling program in Python that demonstrates how to read and write files:
# File Handling Example
import os
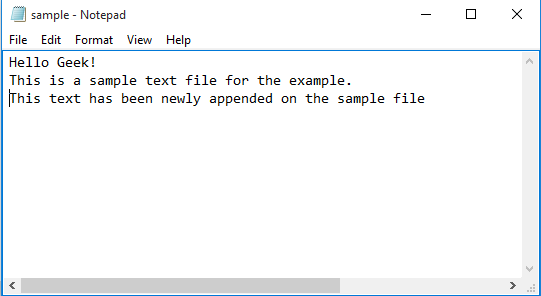
def create_file(filename):
Create a new file with the given filename
try:
f = open(filename, 'w')
f.write('This is a new file.n')
f.close()
except IOError as e:
print(f"Error: {e}")

def read_file(filename):

Read the contents of the given filename
if os.path.exists(filename):
try:
with open(filename, 'r') as f:
content = f.read()
print(content)
except IOError as e:
print(f"Error: {e}")
else:
print(f"{filename} does not exist.")
def append_to_file(filename):
Append some text to the end of the file
try:
with open(filename, 'a') as f:
f.write('This is an appended line.n')
except IOError as e:
print(f"Error: {e}")
Test the functions
create_file('test.txt')
read_file('test.txt')
append_to_file('test.txt')
In this example, we define three functions:
create_file(filename)
: This function creates a new file with the given filename and writes some text to it. read_file(filename)
: This function reads the contents of the file with the given filename and prints them to the console. append_to_file(filename)
: This function appends some text to the end of the file with the given filename.
In the test section, we call these functions in sequence to demonstrate how they work:
create_file('test.txt')
: We create a new file called 'test.txt' and write some text to it. read_file('test.txt')
: We read the contents of the newly created file and print them to the console. append_to_file('test.txt')
: We append some more text to the end of the file.
This program shows how you can perform common operations with files in Python, such as creating a new file, reading its contents, and appending text to it.
Note: This is just an example code, you should consider error handling and exceptions when working with files.