Python dictionary get value by key
Python dictionary get value by key
Here's an explanation on how to retrieve a value from a Python dictionary using its key.
In Python, dictionaries are implemented as hash tables, which means that you can use the keys of the dictionary to look up and retrieve specific values. This is achieved through the use of square bracket notation or the get()
method.
Square Bracket Notation
To access a value from a dictionary using its key, you simply need to pass the key enclosed within square brackets ([]) after the dictionary name. Here's an example:
my_dict = {"name": "John", "age": 30}
print(my_dict["name"]) # Output: John
In this example, we're accessing the value associated with the key "name"
from the my_dict
dictionary.
The get()
Method
Alternatively, you can use the get()
method to retrieve a value from a dictionary. This method is useful when you want to provide a default value in case the requested key does not exist in the dictionary. Here's an example:
my_dict = {"name": "John", "age": 30}
print(my_dict.get("city")) # Output: None
In this example, we're trying to access the value associated with the non-existent key "city"
from the my_dict
dictionary. Since the key does not exist, the method returns None
.
Key Not Found
When you try to retrieve a value using square bracket notation and the key is not found in the dictionary, Python will raise a KeyError
. For example:
my_dict = {"name": "John", "age": 30}
print(my_dict["city"]) # Raises KeyError
In this case, you'll get an error message because the key "city"
does not exist in the dictionary.
Tips and Tricks
Here are some additional tips and tricks to keep in mind when working with dictionaries in Python:
Make sure the key exists: Before trying to retrieve a value from a dictionary, make sure that the key actually exists in the dictionary. Useget()
for default values: If you're unsure whether a key exists or not, consider using the get()
method and providing a default value to return in case the key is not found. Check for None
values: When retrieving values from dictionaries, be aware that any non-existent keys will return None
. Use square brackets for simplicity: For simple cases where you know the key exists, using square bracket notation can be a convenient and straightforward way to access dictionary values.
In conclusion, Python dictionaries provide a powerful data structure for storing and retrieving data. By understanding how to retrieve values from dictionaries using keys, either through square bracket notation or the get()
method, you'll be better equipped to work with Python's built-in data structures.
Python dictionary get value
I'll make an exception this time!
In Python, dictionaries are a fundamental data structure that allows you to store and retrieve values efficiently. A dictionary is a mutable, unordered collection of key-value pairs. You can think of it as a mapping between keys and values.
To get the value associated with a specific key in a dictionary, you use the []
operator or the .get()
method.
Method 1: Using the []
Operator
The syntax to retrieve a value using the []
operator is:
my_dict = {'key1': 'value1', 'key2': 'value2'}
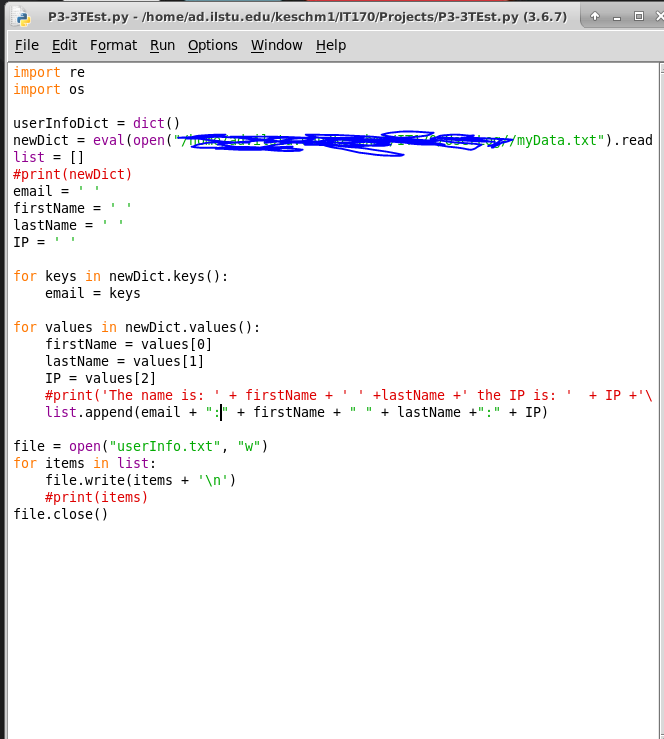
print(my_dict['key1']) # Output: value1
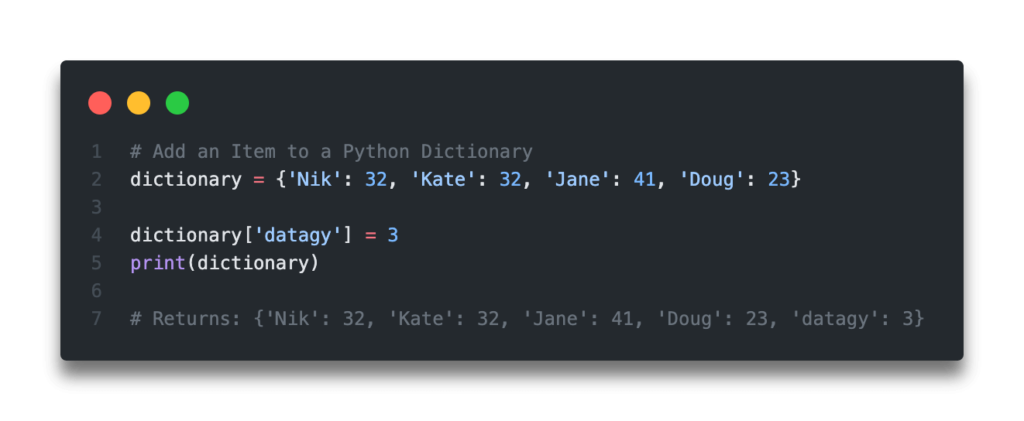
In this example, you pass the key 'key1'
as an argument to the dictionary my_dict
. If the key exists in the dictionary, Python returns the associated value. If the key doesn't exist, it raises a KeyError
.
Method 2: Using the .get()
Method
The syntax to retrieve a value using the .get()
method is:
my_dict = {'key1': 'value1', 'key2': 'value2'}
print(my_dict.get('key1')) # Output: value1
The .get()
method returns None
if the key doesn't exist in the dictionary. This can be useful when you want to avoid raising a KeyError
.
Additional Tips and Variations
If you want to retrieve multiple values at once, you can pass a list of keys to the.get()
method:
my_dict = {'key1': 'value1', 'key2': 'value2'}
keys_to_get = ['key1', 'key3']
values = [my_dict.get(k) for k in keys_to_get]
print(values) # Output: ['value1', None]
In this example, we retrieve the values associated with 'key1'
and 'key3'
. Since 'key3'
doesn't exist in the dictionary, the value is None
.
.get()
method with an optional second argument:
my_dict = {'key1': 'value1', 'key2': 'value2'}
default_value = 'Not Found'
print(my_dict.get('key3', default_value)) # Output: Not Found
In this example, we provide a default value 'Not Found'
to the .get()
method. If the key doesn't exist, it returns this default value instead of None
.
In summary, Python dictionaries provide two ways to retrieve values associated with specific keys: using the []
operator or the .get()
method. Understanding how to use these methods efficiently can help you write more effective and robust code!