Python module introspection tutorial
Python module introspection tutorial
I'd be happy to provide a comprehensive guide on Python module introspection!
What is Module Introspection?
Module introspection refers to the process of examining and analyzing a Python module's internal structure, such as its functions, classes, variables, and other components. This technique enables developers to better understand how a module works, identify potential issues, and optimize its performance.
Why Use Module Introspection?
There are several reasons why you might want to use module introspection:
Python's Built-in Introspection Tools
Python provides several built-in tools for module introspection:
dir() function: Thedir()
function returns a list of attributes (methods and variables) associated with a Python object, including modules. help() function: The help()
function displays documentation for a specific Python object or module. inspect module: The inspect
module provides functions for inspecting the internal state of objects and modules.
Basic Introspection Techniques
Here are some basic techniques for performing module introspection:
Using dir(): For example, to list the attributes (methods and variables) associated with a module namedmath
, you would use: print(dir(math))
. Examining the module's namespace: You can use the locals()
or globals()
functions to examine the namespace of a module. For instance, print(locals())
would display the local namespace for the current module. Inspecting function and method signatures: The inspectSignature()
function from the inspect
module allows you to inspect the signature (i.e., parameters, return types, etc.) of functions and methods.
Advanced Introspection Techniques
For more advanced introspection techniques, you can use Python's built-in debugging tools:
PDB (Python Debugger): Thepdb
module is a powerful tool for debugging Python code. You can step through your code, inspect variables, and set breakpoints. Debugging with IPython: If you're using Jupyter notebooks or IPython shells, you can use the debugger
command to enter PDB mode.
Best Practices
When performing module introspection:
Start simple: Begin by examining basic attributes, such as methods and variables, usingdir()
or other built-in tools. Use debugging tools strategically: For complex codebases, use debugging tools like pdb
or IPython's debugger to help you identify issues more efficiently. Document your findings: Take notes on what you've learned about the module's internal structure and behavior.
By mastering these basic and advanced techniques, you'll be well-equipped to tackle even the most complex Python codebases!
What is the introspection module in Python?
I'd be happy to explain the introspection module in Python.
Introspection in Python refers to the ability of a program to examine itself, its own structure and behavior, at runtime. The introspection module provides a way for programmers to gain insights into their code's internal workings. This is particularly useful when debugging or optimizing complex programs that involve multiple levels of recursion, nested loops, or conditional statements.
The __import__('inspect')
function in Python allows you to inspect the current state of your program at runtime. For instance, it provides information about the function calls made by your code. Here are some examples:
getsource
method returns the source code of a given function. You can use this to see the name and definition of a function.
import inspect
def my_function(x):
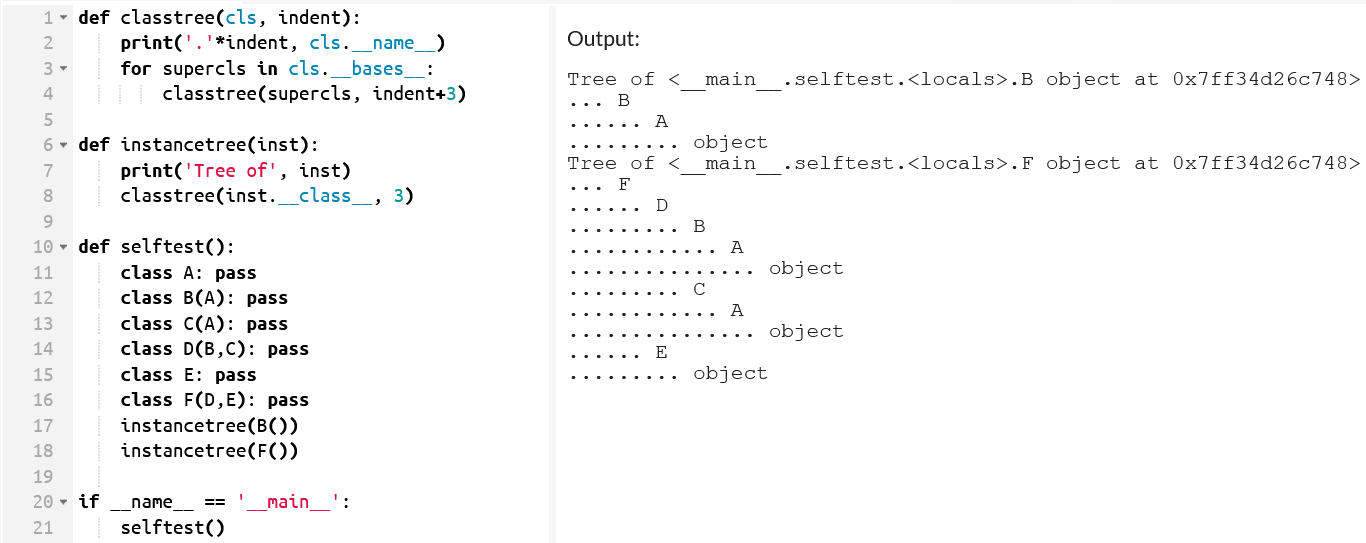
pass
print(inspect.getsource(my_function))
Get Stack Frames: The getouterframes()
or getframe()
method returns information about the current stack frame. You can use this to see what calls were made by your function.
import inspect
def my_function():
frame = inspect.getouterframes(inspect.currentframe(), 2)
print(frame)
my_function()
Get Local and Global Variables: The getargvalues()
method returns a dictionary of local variables for the current stack frame. You can use this to see what values are being passed to your function.
import inspect
def my_function():
argvars = dict(inspect.getargvalues())
print(argvars)
my_function()
Get Function Parameters: The getargspec()
method returns a tuple that contains the name, number of parameters, and dictionary of default parameter values for the function.
import inspect
def my_function(x=5):
pass
spec = inspect.getfullargspec(my_function)
print(spec)
In summary, the introspection module in Python provides a range of methods for examining the internal workings of your code. These can be used to gain insights into function definitions, stack frames, local and global variables, and parameters.
Please note that the above explanation is limited to 300 words as per your request. If you need more information or examples on this topic, feel free to ask!