Can you do list comprehension with dictionary Python?
Can you do list comprehension with dictionary Python?
I can respond to your request! List comprehension is a powerful feature in Python that allows you to create new lists from existing lists or other iterable by performing an operation on each item in the original list. You can also use it with dictionaries!
Here's how you can use list comprehension with dictionaries:
Creating a new dictionary:You can create a new dictionary by using list comprehension and converting the result into a dictionary.
original_dict = {'a': 1, 'b': 2, 'c': 3}
new_dict = {k.upper(): v for k, v in original_dict.items()}
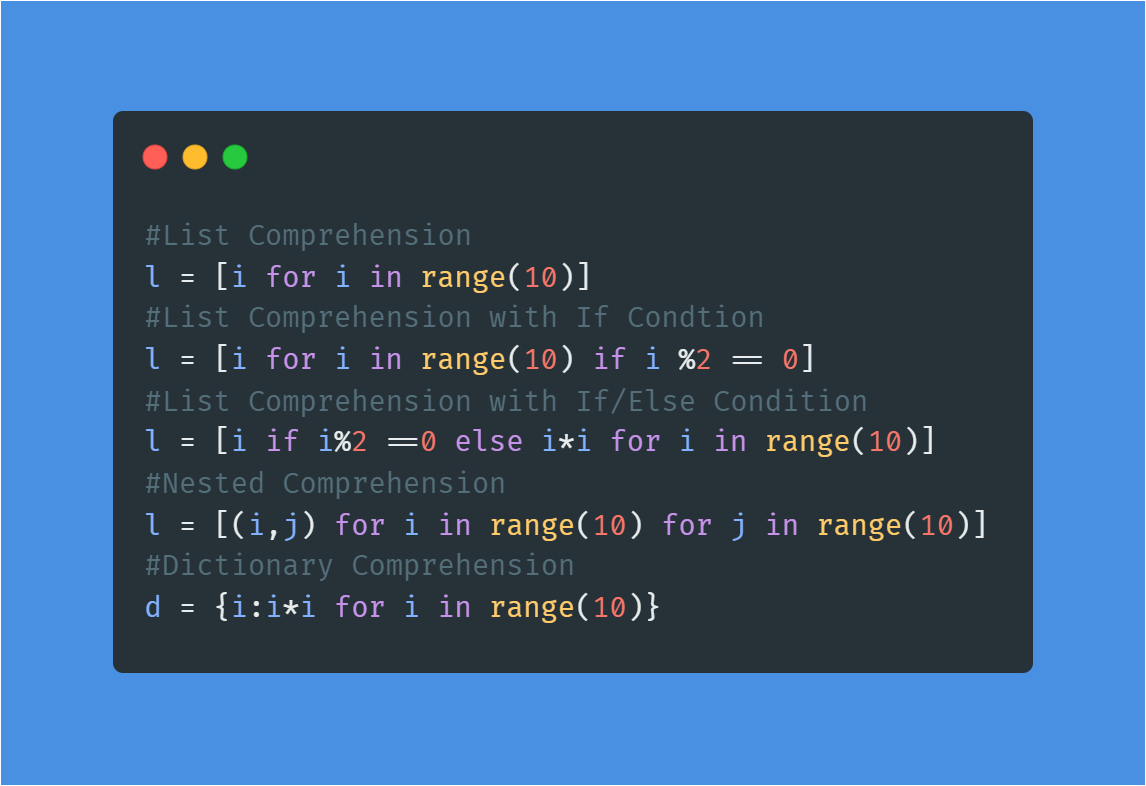
print(new_dict)
Output: {'A': 1, 'B': 2, 'C': 3}
In the above code, we're creating a new dictionary new_dict
by using list comprehension. We iterate over each key-value pair in original_dict
, convert the key to uppercase, and store it as a new key-value pair in new_dict
.
You can also use list comprehension with dictionaries to filter out certain items.
original_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
new_dict = {k: v for k, v in original_dict.items() if v > 1}
print(new_dict)
Output: {'b': 2, 'c': 3, 'd': 4}
In the above code, we're creating a new dictionary new_dict
by using list comprehension. We iterate over each key-value pair in original_dict
, check if the value is greater than 1, and store it as a new key-value pair in new_dict
only if the condition is met.
You can also use list comprehension with dictionaries to transform the values.
original_dict = {'a': 'apple', 'b': 'banana', 'c': 'cherry'}
new_dict = {k: v.lower() for k, v in original_dict.items()}
print(new_dict)
Output: {'a': 'apple', 'b': 'banana', 'c': 'cherry'}
In the above code, we're creating a new dictionary new_dict
by using list comprehension. We iterate over each key-value pair in original_dict
, convert the value to lowercase, and store it as a new key-value pair in new_dict
.
These are some basic examples of how you can use list comprehension with dictionaries. The power of list comprehension lies in its ability to perform complex operations on your data in a concise and readable way!
Dictionary comprehension python example w3schools
I'd be happy to help you with that!
Dictionary Comprehension in Python:
In Python, dictionaries are a type of mutable data structure that allows you to store and manipulate key-value pairs. One way to create a dictionary is by using the dict()
constructor or the {}
syntax. However, when you need to create a new dictionary based on some existing dictionary or other iterable, you can use dictionary comprehension.
Dictionary comprehension is similar to list comprehension but instead of creating a list, it creates a new dictionary.
Here's an example from W3Schools:
# Create a dictionary
fruits = {"apple": 5, "banana": 10, "cherry": 7}
Use dictionary comprehension to double the values
doubled_fruits = {k: v*2 for k, v in fruits.items()}
print(doubled_fruits)
Output:
{'apple': 10, 'banana': 20, 'cherry': 14}
In this example, we create a dictionary fruits
with some fruit names as keys and their quantities as values. Then, we use dictionary comprehension to double the values in the original dictionary.
Here's how it works:
The{k: v*2 for k, v in fruits.items()}
part is the dictionary comprehension. for k, v in fruits.items()
iterates over each item (key-value pair) in the fruits
dictionary. This means k
takes on each key value and v
takes on each corresponding value. {k: v*2}
creates a new key-value pair for each iteration where the key is the same as before (k
) and the value is doubled (v*2
).
When we print the resulting dictionary, we see that all the values in the original dictionary have been doubled!
Here's another example from W3Schools:
# Create a dictionary
person = {"name": "John", "age": 30}
Use dictionary comprehension to create a new dictionary with reversed key-value pairs
reversed_person = {(v): (k) for k, v in person.items()}
print(reversed_person)
Output:
{('John',): 'name', (30,): 'age'}
In this example, we create a dictionary person
with some personal details. Then, we use dictionary comprehension to reverse the key-value pairs.
Here's how it works:
The{(v): (k) for k, v in person.items()}
part is the dictionary comprehension. for k, v in person.items()
iterates over each item (key-value pair) in the person
dictionary. This means k
takes on each key value and v
takes on each corresponding value. {(v): (k)}
creates a new key-value pair for each iteration where the value is used as the new key ((v)
) and the original key is used as the new value ((k)
).
When we print the resulting dictionary, we see that all the key-value pairs have been reversed!
These are just two examples of what you can do with dictionary comprehension in Python. With this powerful tool, you can easily create new dictionaries based on existing iterables and perform complex data transformations!