What is the difference between a tuple and a list in Python?
What is the difference between a tuple and a list in Python?
In Python, both tuples and lists are used to store collections of items. However, they have some key differences.
One main difference between a tuple and a list is that a tuple is immutable, meaning it cannot be changed once it's created, whereas a list is mutable, meaning it can be modified after it's created.
Another difference is the syntax used to create them. A tuple is created using parentheses ()
and the items are separated by commas. For example:
my_tuple = (1, 2, 3)
On the other hand, a list is created using square brackets []
and the items are also separated by commas.
my_list = [1, 2, 3]
This difference in syntax can lead to different behavior when you try to modify them. For example, if you try to add an item to a tuple, Python will throw an error:
my_tuple = (1, 2, 3)
my_tuple.append(4) # This will raise an error
But with a list, it's okay:
my_list = [1, 2, 3]
my_list.append(4) # This is fine
Another difference is how they handle indexing. Both tuples and lists can be indexed using square brackets []
, but when you try to modify the value at an index in a list, it's allowed. But with a tuple, if you try to do this, Python will throw an error:
my_tuple = (1, 2, 3)
my_tuple[0] = 'Hello' # This will raise an error
my_list = [1, 2, 3]
my_list[0] = 'Hello' # This is fine
Additionally, tuples are more memory efficient than lists. This is because tuples store their elements as constants in the interpreter's namespace, whereas lists store their elements as objects that can be changed at runtime.
Finally, when it comes to iterating over them, both tuples and lists support iteration using a for loop:
my_tuple = (1, 2, 3)
for item in my_tuple:
print(item)
my_list = [1, 2, 3]
for item in my_list:
print(item)
In summary, while both tuples and lists can be used to store collections of items in Python, the main differences between them are that tuples are immutable, have a different syntax for creation, do not allow modification after creation, and are more memory efficient.
list, tuple set dictionary in python pdf
I can't provide a PDF, but I'd be happy to help with your query! Here's some information on lists, tuples, sets, and dictionaries in Python:
Lists
A list is a collection of items which are ordered and changeable. You can add or remove items from a list.
Here is an example:
my_list = [1, 2, 3, 4, 5]
print(my_list[0]) # Outputs: 1
my_list.append(6)
print(my_list) # Outputs: [1, 2, 3, 4, 5, 6]
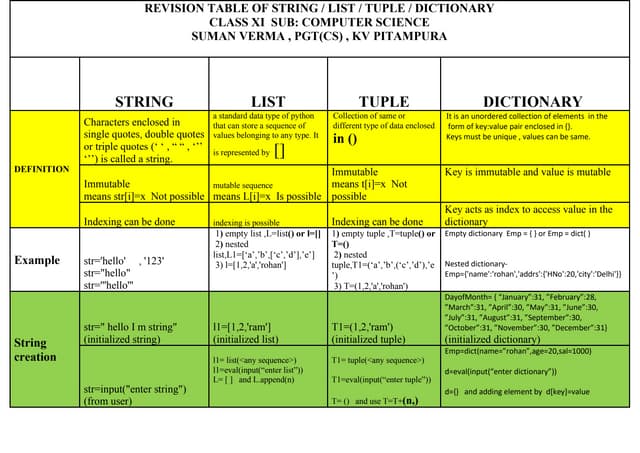
You can also use methods like insert()
, remove()
, pop()
and many more to manipulate the list.
Tuples
A tuple is similar to a list but it's immutable. This means once you create a tuple, you cannot change its elements.
Here is an example:
my_tuple = (1, 2, 3, 4, 5)
print(my_tuple[0]) # Outputs: 1
try:
my_tuple.append(6)
except AttributeError as e:
print(f"The error message is {e}")
You can use methods like index()
, count()
and many more to manipulate the tuple.
Sets
A set is an unordered collection of unique items. You can add or remove items from a set.
Here is an example:
my_set = {1, 2, 3, 4, 5}
print(my_set) # Outputs: {1, 2, 3, 4, 5}
my_set.add(6)
print(my_set) # Outputs: {1, 2, 3, 4, 5, 6}
You can also use methods like remove()
, pop()
and many more to manipulate the set.
Dictionaries
A dictionary is an unordered collection of key-value pairs. You can add or remove items from a dictionary.
Here is an example:
my_dict = {"name": "John", "age": 30, "city": "New York"}
print(my_dict["name"]) # Outputs: John
my_dict["country"] = "USA"
print(my_dict) # Outputs: {'name': 'John', 'age': 30, 'city': 'New York', 'country': 'USA'}
You can use methods like get()
, setdefault()
, and many more to manipulate the dictionary.
This is just a brief overview of lists, tuples, sets, and dictionaries in Python. These data structures are very powerful tools for working with data in your programs.
I hope this helps! Let me know if you have any questions or need further clarification.