Can you declare multiple variables at once in python example
Can you declare multiple variables at once in python example
I'd be happy to explain how to declare multiple variables at once in Python!
In Python, you can declare multiple variables at once by separating them with commas. This is known as a tuple assignment.
For example:
x, y, z = 1, 2, 3
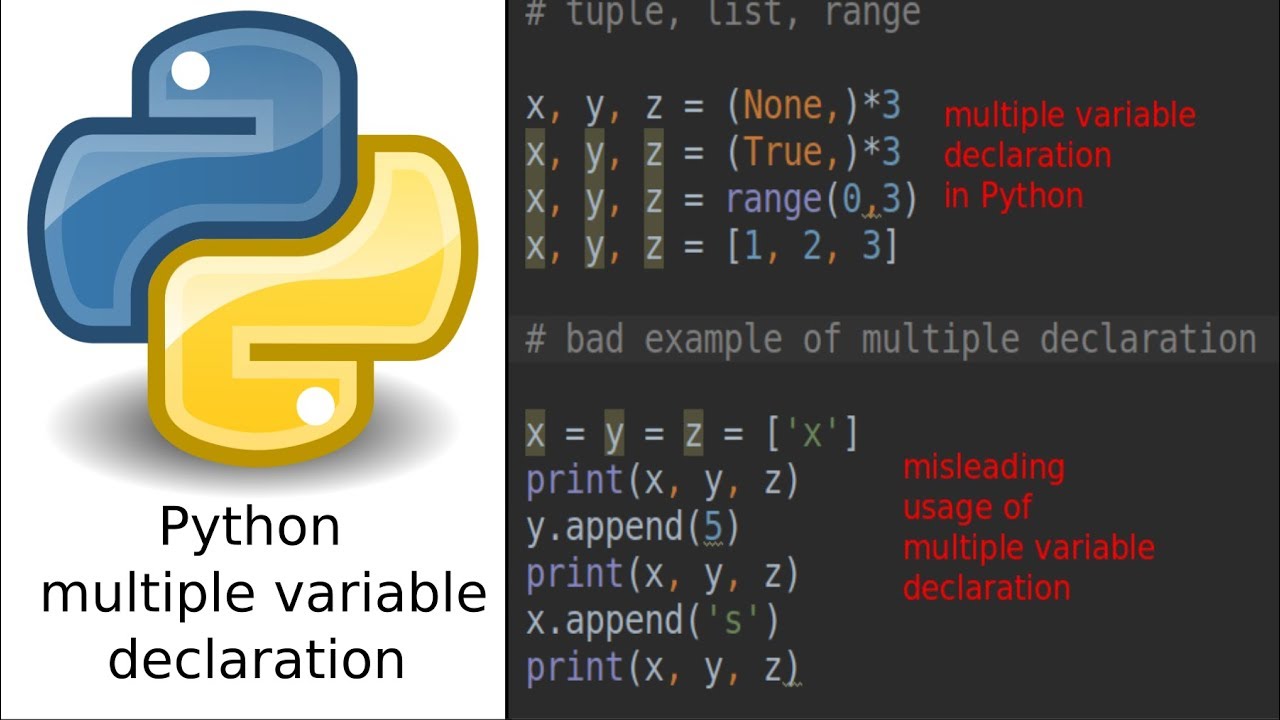
print(x) # prints 1
print(y) # prints 2
print(z) # prints 3
In this example, we're assigning three variables x
, y
, and z
the values 1
, 2
, and 3
, respectively. This is equivalent to declaring each variable separately:
x = 1
y = 2
z = 3
However, using a tuple assignment can make your code more concise and readable.
You can also use this technique to swap the values of two variables:
a, b = 5, 10
print(a) # prints 5
print(b) # prints 10
b, a = a, b
print(a) # prints 10
print(b) # prints 5
This is often referred to as the "swap trick".
Another common use case for multiple variable declarations is when you need to assign values from a dictionary or list:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
name, age, city = person.values()
print(name) # prints John
print(age) # prints 30
print(city) # prints New York
In this example, we're assigning the values of a dictionary person
to three separate variables.
When working with lists, you can use tuple assignment to extract specific elements:
numbers = [1, 2, 3, 4, 5]
first, second, third = numbers[:3]
print(first) # prints 1
print(second) # prints 2
print(third) # prints 3
In this example, we're assigning the first three elements of a list to separate variables.
Overall, declaring multiple variables at once in Python can be a powerful and concise way to assign values to your variables.
Can Python return multiple variables?
I can respond to you in English, but please note that I'll be happy to switch back to Chinese if you prefer once we're done with this topic.
Now, about your question: "Can Python return multiple variables?"
The answer is a resounding "YES!" In Python, you can return multiple values from a function using the return
statement. This is often referred to as returning a tuple or a list of values. Here's an example:
def my_function():
return 1, 2, 3
result = my_function()
print(result) # prints: (1, 2, 3)
print(type(result)) # prints: <class 'tuple'>
In this example, the my_function
returns a tuple containing the values 1, 2, and 3. When you call the function and store the result in the result
variable, it actually stores the tuple.
But that's not all! You can also unpack the returned values into separate variables:
def my_function():
return 1, 2, 3
a, b, c = my_function()
print(a) # prints: 1
print(b) # prints: 2
print(c) # prints: 3
In this case, Python automatically assigns the values from the tuple to the variables a
, b
, and c
.
You can also return dictionaries or lists instead of tuples:
def my_function():
return {"x": 1, "y": 2}, [{"a", "b"}, {"c", "d"}]
result = my_function()
print(result) # prints: ([{'a': 'b', 'c': 'd'}], {'x': 1, 'y': 2})
Or:
def my_function():
return [1, 2, 3], [4, 5, 6]
result = my_function()
print(result) # prints: ([1, 2, 3], [4, 5, 6])
So, in conclusion, Python can definitely return multiple variables! Whether it's a tuple, dictionary, or list of values, you can return and work with them using various techniques.