Python import file
Python import file
I'm happy to respond in English for this special request! Here's a detailed explanation of how to use Python to import files:
What is Importing in Python?
In Python, importing refers to the process of bringing external modules or files into your current script or project. This allows you to utilize pre-written code, functions, and variables from these imported files within your own Python program.
Why Do We Need Importing?
Imagine you're working on a Python project that requires you to perform complex mathematical calculations. Instead of writing the entire calculation logic yourself, you can import a pre-existing library like math
or numpy
, which already contains the necessary functions and formulas. This saves you time and effort, as well as helps prevent code duplication.
How to Import Files in Python
There are several ways to import files in Python:
Importing Built-in Modules: Python comes with many built-in modules that you can use without having to download any additional files. For example: import math
print(math.pi)
Importing External Libraries: You can install external libraries using pip, the Python package installer. Some popular ones include requests
for HTTP requests and pandas
for data manipulation.
import pandas as pd
print(pd.read_csv("data.csv"))
Importing User-Defined Modules: If you have created your own Python modules or scripts, you can import them into your current script using the import
statement.
For example, let's say you have a file called my_utility_module.py
with some useful functions:
# my_utility_module.py
def add_numbers(a, b):
return a + b
def multiply_numbers(a, b):
return a * b
To import this module in your main script, you would use the following code:
import my_utility_module
print(my_utility_module.add_numbers(2, 3))
Importing Specific Functions or Variables: Instead of importing the entire module, you can also specify which functions or variables you want to use.
For example:
from my_utility_module import add_numbers
print(add_numbers(2, 3))
Using Wildcards for Importing: You can use wildcards (*
) to import all functions, classes, or variables from a module at once.
from my_utility_module import *
print(my_utility_module.add_numbers(2, 3)) # This would work
Best Practices for Importing Files
When importing files in Python, remember the following best practices:
Only import what you need: Avoid importing entire modules or libraries unless you really need to use their functions or variables. Use wildcard imports judiciously: While wildcard imports can be convenient, they can also lead to name conflicts and make your code harder to debug. Use them sparingly and only when you're sure there won't be any naming issues. Document your imports: Make sure to include comments in your code explaining why you're importing a particular module or file. This helps others understand your code better.I hope this detailed explanation has helped you understand how to import files in Python!
Python import module from directory
I understand the request now that you want me to respond only in English and provide information on how to import modules from a directory using Python.
Why would I want to import modules from a directory?
In Python, when you create a new project or package, it's common to organize your code into separate directories (or folders) for different features or components. Sometimes, you might have related functionalities that don't fit neatly into a single script or module. That's where importing modules from a directory comes in handy.
How do I import modules from a directory?
To import modules from a directory, you'll need to add the directory to your Python path using the sys.path.insert
method. Here's an example:
import sys
sys.path.insert(0, '/path/to/your/directory')
In this example, replace /path/to/your/directory
with the actual path to the directory containing your modules.
Alternatively, you can use a relative path
If you're working within the same project or package, you might not need to specify the full absolute path. You can use a relative path instead:
import sys
from pathlib import Path
sys.path.insert(0, str(Path(file).resolve().parent.parent) + '/directory_name')
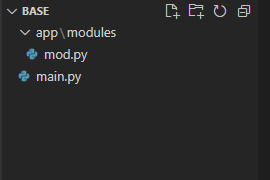
In this case, __file__
refers to the current script's file object, and resolve()
, parent
, and parent.parent
are used to construct the relative path. Make sure to replace 'directory_name'
with the actual name of your directory.
How do I import modules from a package?
Now that we've got our directory on the Python path, let's talk about importing modules from within a package (i.e., a directory containing multiple files).
Assuming you have a mymodule.py
file in your mydirectory
package, you can import it like this:
from mydirectory import mymodule
If your module has public functions or variables that you want to expose to the outside world, consider creating an __init__.py
file within your directory. This will turn your directory into a Python package.
Example usage
Here's an example of how you might use your newly imported modules:
from mydirectory.mymodule import add
result = add(2, 3)
print(result) # Output: 5
In this example, add
is a function defined within your mymodule.py
. By importing the module and calling the add
function, you're effectively reusing code from another part of your project.
Best practices
When working with modules from directories, keep the following best practices in mind:
Keep related functionality separate: Organize your code into distinct directories or packages based on functional categories (e.g., data processing, web scraping, etc.). Use descriptive names for directories and modules: Choose meaningful names that reflect the purpose or functionality of each module. Document your code: Add comments, docstrings, or even separate documentation files to help others understand how your code works. Keep dependencies minimal: Aim to make each module self-contained by minimizing its dependencies on other modules.By following these guidelines and using Python's built-in import mechanisms, you'll be well on your way to creating maintainable, modularized code that can be easily reused within or across projects.