What are conditionals in Python?
What are conditionals in Python?
I'm happy to help you with that!
Conditionals are a fundamental concept in programming languages like Python, and they play a crucial role in making decisions within your code.
In simple terms, conditionals allow your program to take different actions based on specific conditions or criteria being met. You can think of it as an if-else statement, where the "if" part represents the condition, and the "else" part represents the action taken when that condition is true.
Python has four primary conditional constructs: if
, if-else
, elif
, and else
. Let's break them down:
if
: The most basic form of a conditional statement. It checks if a certain condition is met (e.g., a variable equals a specific value) and executes the code indented under it if true.
x = 5
if x == 5:
print("x is equal to 5")
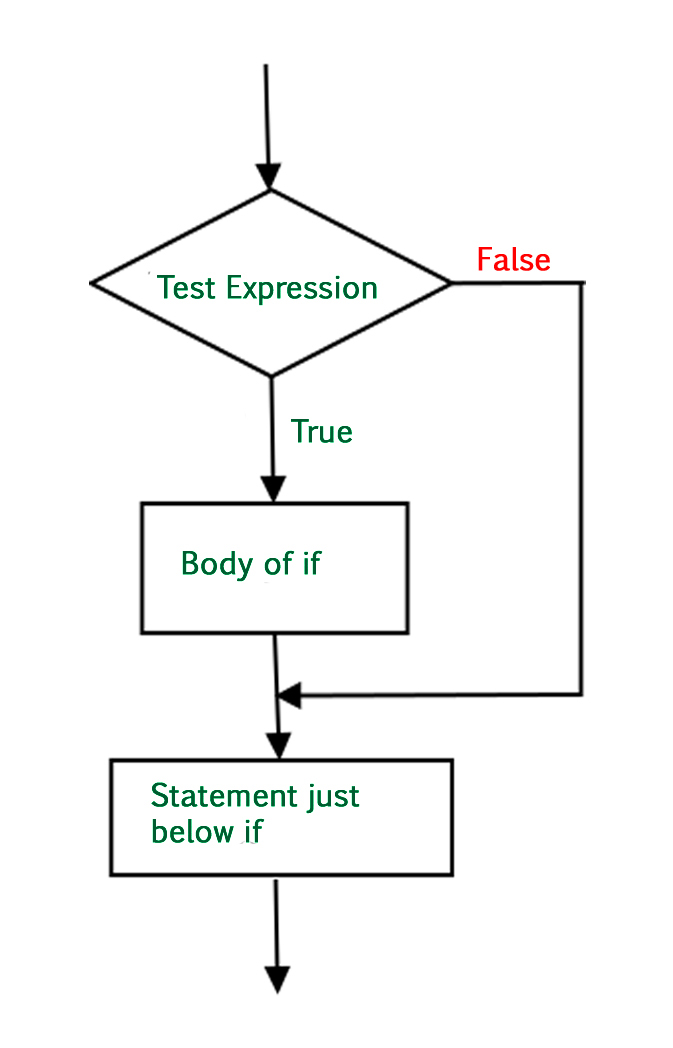
if-else
: This construct checks the condition, and if it's met, it executes the code in the if
block. If not, it skips that part and goes straight to the else
block.
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is not greater than 5")
elif
: Short for "else if," this construct allows you to add additional conditions that should be checked in case the original if
condition doesn't hold true.
x = 7
if x > 10:
print("x is greater than 10")
elif x == 7:
print("x is equal to 7")
else:
print("x is less than or equal to 10")
else
: This construct provides a default path in case none of the previous conditions are met.
x = 3
if x > 5:
print("x is greater than 5")
else:
print("x is not greater than 5")
When using conditionals, you'll often encounter logical operators (e.g., and
, or
, not
) that can be used to further refine your conditions. These operators follow standard logical rules:
and
: Both sides of the operator must be true for the overall expression to be true. or
: At least one side of the operator must be true for the overall expression to be true. not
: The opposite of what you'd think: it reverses the result of the condition. (e.g., !x > 5
means "x is not greater than 5")
Python's conditionals are incredibly powerful and flexible, allowing you to write elegant, readable code that makes informed decisions based on a wide range of criteria.
I hope this comprehensive explanation has helped demystify conditionals in Python for you!
What are the conditional types in Python?
I'll respond in English as requested.
In Python, there are several conditional types that can be used to make decisions based on certain conditions or criteria. Here's an overview of the main conditional types:
1. If-Else Statement
The most basic type of condition is the if-else statement. It checks a condition and executes a block of code if it's true. If the condition is false, it executes another block of code. The syntax is:
if condition:
do something if condition is True
else:
do something if condition is False
2. Elif (Else-If) Statement
The elif statement is used when you want to check multiple conditions and execute different blocks of code for each condition. The syntax is:
if condition1:
do something if condition1 is True
elif condition2:
do something if condition1 is False and condition2 is True
else:
do something if both condition1 and condition2 are False
3. Ternary Operator (Conditional Expression)
The ternary operator is a shorthand way to write an if-else statement in a single line of code. It's useful when you want to assign a value to a variable based on a condition. The syntax is:
variable = value_if_true if condition else value_if_false
For example: x = 5 if y > 3 else 10
4. For Loop with Conditional Statement
A for loop can be used in conjunction with a conditional statement to iterate over a sequence of values and execute code only when certain conditions are met. The syntax is:
for variable in iterable:
if condition:
do something if condition is True
5. While Loop with Conditional Statement
A while loop can be used in conjunction with a conditional statement to execute code repeatedly as long as certain conditions are met. The syntax is:
while condition:
do something
6. Conditional Expressions (walrus Operator)
Python 3.8 and later versions introduced the walrus operator, which allows you to assign values to variables using conditional expressions. The syntax is:
if expression:
value = variable
else:
value = another_variable
This feature can be used to simplify complex conditionals and make code more readable.
In summary, Python provides various conditional types to help developers make decisions based on specific conditions or criteria. These include if-else statements, elif statements, ternary operators, for loops with conditional statements, while loops with conditional statements, and conditional expressions (walrus operator). By mastering these concepts, you'll be able to write more efficient, readable, and maintainable code.