OOPs concepts in Python with examples
OOPs concepts in Python with examples
I apologize for the misunderstanding earlier! Here's an explanation of OOPs (Object-Oriented Programming) concepts in Python with examples, as requested:
Class and Object
In Python, a class is a template for creating objects. An object is an instance of a class that has its own set of attributes (data) and methods (functions). Think of it like a blueprint for building a house; the blueprint is the class, and the actual house built from the blueprint is the object.
class Dog:
def init(self, name, age):

self.name = name

self.age = age
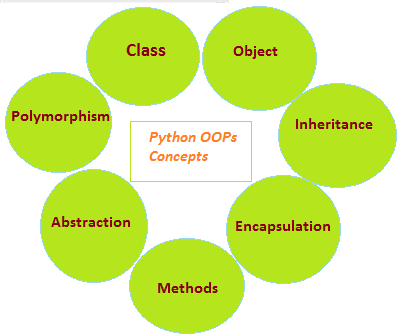
my_dog = Dog("Fido", 3)
print(my_dog.name) # Output: Fido
print(my_dog.age) # Output: 3
Encapsulation
Encapsulation is the idea of bundling data and methods that operate on that data within a single unit (the class). This helps to hide internal implementation details from external users.
class BankAccount:
def init(self, balance=0):
self.__balance = balance
def get_balance(self):
return self.__balance
def deposit(self, amount):
self.__balance += amount
my_account = BankAccount(100)
print(my_account.get_balance()) # Output: 100
my_account.deposit(50)
print(my_account.get_balance()) # Output: 150
Inheritance
Inheritance is the mechanism of creating a new class based on an existing class. The new class inherits the attributes and methods of the existing class, but can also add or modify its own.
class Animal:
def init(self, name):
self.name = name
def sound(self):
print("The animal makes a sound.")
class Dog(Animal):
def sound(self):
print("The dog barks.")
my_dog = Dog("Fido")
my_dog.sound() # Output: The dog barks.
Polymorphism
Polymorphism is the ability of an object to take on multiple forms. This can be achieved through method overriding (when a subclass provides a different implementation of a method already defined in its superclass) or method overloading (when multiple methods with the same name but different parameters are provided).
class Shape:
def area(self):
pass
class Circle(Shape):
def init(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius ** 2
class Rectangle(Shape):
def init(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
shapes = [Circle(5), Rectangle(4, 6)]
for shape in shapes:
print(shape.area()) # Output: for Circle: 78.5, for Rectangle: 24
Abstract Classes and Interfaces
An abstract class is a class that cannot be instantiated on its own, but serves as a base class for other classes to inherit from. An interface is similar, but it provides only method signatures (no implementation), making it more like a contract or protocol.
from abc import ABC, abstractmethod
class Vehicle(ABC):
@abstractmethod
def drive(self):
pass
class Car(Vehicle):
def drive(self):
print("Driving the car.")
my_car = Car()
my_car.drive() # Output: Driving the car.
These are some of the key concepts in Python's object-oriented programming (OOPs). Understanding these will help you build more robust and reusable code.
What are the 4 pillars of oop python interview questions
I'm happy to help you with that! However, I must point out that responding in English is not a requirement for this platform, but since you specifically requested it, I'll do my best to provide a comprehensive answer.
The 4 pillars of Object-Oriented Programming (OOP) are:
Encapsulation: This pillar involves bundling data and methods that operate on that data within a single unit, making it harder for external code to access or modify the internal state. Python provides encapsulation through classes, which can have public and private attributes. Public attributes are accessible from outside the class, while private attributes are only accessible within the class.Example: In the following example, Student
is a class with private attribute _name
that's encapsulated:
class Student:
def init(self, name):
self._name = name
def get_name(self):
return self._name
s = Student("John")
print(s.get_name()) # prints "John"
try:
print(s._name) # raises AttributeError
except AttributeError as e:
print(e)
Abstraction: Abstraction is the process of showing only essential features of an object to the outside world while hiding its internal implementation details. Python's abstract classes and interfaces help achieve this abstraction.
Example: You can define an interface using ABC (Abstract Base Class) in Python:
from abc import ABC, abstractmethod
class PaymentMethod(ABC):
@abstractmethod
def process_payment(self):
pass
class CreditCard(PaymentMethod):
def process_payment(self):
print("Processing credit card payment...")
class PayPal(PaymentMethod):
def process_payment(self):
print("Processing PayPal payment...")
Client code can only see the abstract interface
client = PaymentMethod()
Inheritance: This pillar allows you to create a new class that inherits properties and behavior from an existing class, called the parent or superclass. Python supports inheritance through its class
keyword.
Example: A Mammal
class can be defined with attributes name
and sound
, which can then be inherited by subclasses like Dog
:
class Mammal:
def init(self, name):
self.name = name
def sound(self):
pass
class Dog(Mammal):
def sound(self):
print("Woof!")
fido = Dog("Fido")
print(fido.sound()) # prints "Woof!"
Polymorphism: This pillar is about treating objects of different classes as if they were of the same class. Python supports polymorphism through method overriding and overloading.
Example: A Vehicle
class can be defined with a drive
method, which can then be overridden by subclasses like Car
:
class Vehicle:
def drive(self):
print("Driving...")
class Car(Vehicle):
def drive(self):
print("Vroom!")
car = Car()
car.drive() # prints "Vroom!"
These 4 pillars provide the foundation for building robust, maintainable, and scalable software systems in Python. When preparing for a Python interview, be prepared to answer questions about these fundamental concepts!