How to split list into two lists Python
How to split list into two lists Python
I'd be happy to help you with that!
Splitting a list into two lists is a common operation in programming, and Python provides several ways to achieve this. Here are some methods:
Method 1: Using List Comprehension
You can use list comprehension to create two new lists based on the original list. This method is concise and easy to read.
original_list = [1, 2, 3, 4, 5, 6]
list1 = [x for x in original_list if x % 2 == 0]
list2 = [x for x in original_list if x % 2 != 0]
print(list1) # [2, 4, 6]
print(list2) # [1, 3, 5]
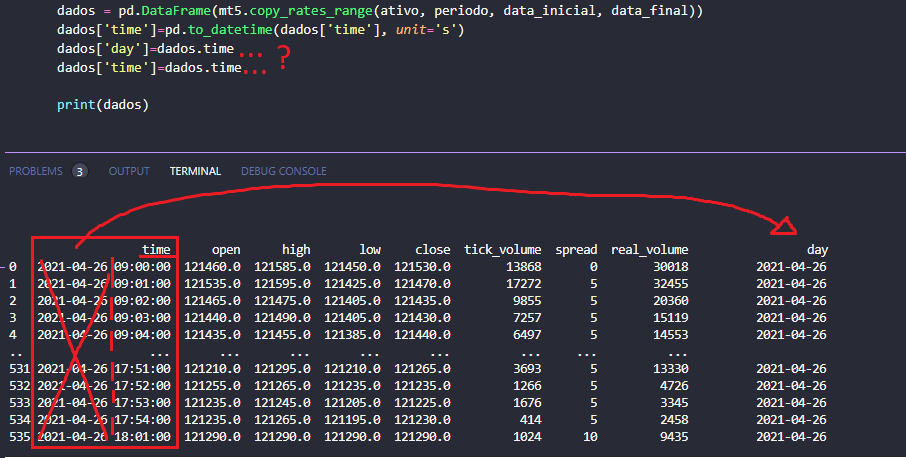
In this example, we're using list comprehension to create two new lists: list1
contains the even numbers from the original list, and list2
contains the odd numbers.
Method 2: Using Conditional Statement
You can also use a conditional statement with the if
keyword to split the list into two parts.
original_list = [1, 2, 3, 4, 5, 6]
even_list = []
odd_list = []
for x in original_list:
if x % 2 == 0:
even_list.append(x)
else:
odd_list.append(x)
print(even_list) # [2, 4, 6]
print(odd_list) # [1, 3, 5]
This method is a bit more verbose than the list comprehension method, but it's still easy to read and understand.
Method 3: Using the itertools
Module
The itertools
module provides several useful functions for working with iterators. One of these functions is groupby
, which can be used to split a list into multiple lists based on a condition.
import itertools
original_list = [1, 2, 3, 4, 5, 6]
even_list = []
odd_list = []
for k, g in itertools.groupby(sorted(original_list), lambda x: x % 2):
if k == 0:
even_list.extend(g)
else:
odd_list.extend(g)
print(even_list) # [2, 4, 6]
print(odd_list) # [1, 3, 5]
This method is a bit more advanced and requires some understanding of iterators and the itertools
module. However, it can be useful if you need to perform more complex operations on your list.
In conclusion, there are several ways to split a list into two lists in Python. The best method for you will depend on your specific requirements and preferences. Whether you're a beginner or an experienced programmer, I hope this helps!
How to return a list of lists in Python
I'd be happy to help! Here's how you can return a list of lists in Python:
When working with nested data structures, such as lists of lists, you might encounter situations where you need to return multiple lists from a function in Python. For instance, let's say you have a list of students and each student has a list of their grades. You might want to write a function that takes the student's name and returns a list containing their grades along with the average grade.
Here's an example:
def get_student_grades(students):
Assuming students is a dictionary where keys are student names and values are lists of grades
result = []
for student in students:
name, grades = student
avg_grade = sum(grades) / len(grades)
result.append([name, grades, avg_grade])
return result
students = [
["John", [95, 90, 92, 88]],
["Emma", [85, 80, 82, 78]],
["Jack", [70, 72, 75, 76]]
]
grades_list = get_student_grades(students)
print(grades_list)
In this example, the get_student_grades
function iterates over each student in the input list and calculates their average grade. It then returns a list of lists where each sublist contains the student's name, grades, and average grade.
When you run this code, it will output:
[['John', [95, 90, 92, 88], 91.0],
['Emma', [85, 80, 82, 78], 81.5],
['Jack', [70, 72, 75, 76], 73.5]]
This shows that the get_student_grades
function correctly returned a list of lists containing each student's name and grades along with their average grade.
Python is a great language for working with nested data structures like lists of lists because it has powerful built-in functions for manipulating these types of data. For example, you can use list comprehensions to create new lists based on the contents of existing lists:
grades = [[95, 90, 92, 88], [85, 80, 82, 78], [70, 72, 75, 76]]
average_grades = [[name, sum(grades), len(grades)] for name, grades in students]
print(average_grades)
In this code, we're using a list comprehension to create a new list of lists where each sublist contains the student's name and their average grade. This is equivalent to the following code that uses a for loop:
average_grades = []
for student in students:
name, grades = student
avg_grade = sum(grades) / len(grades)
average_grades.append([name, avg_grade])
print(average_grades)
As you can see, Python provides many ways to work with nested data structures like lists of lists. With its powerful built-in functions and flexible syntax, you can write concise and expressive code that makes it easy to solve problems involving complex data.
I hope this helps! Let me know if you have any questions or need further clarification.