String in Python
String in Python
I'll respond in English and provide a string manipulation example using Python.
String manipulation is a crucial aspect of programming, as it allows you to process and transform text data according to your needs. In Python, strings are sequences of characters, often used to represent text, file paths, or other forms of text-like data.
Here's a simple example of how to manipulate strings in Python:
# Define a string
original_string = "Hello, my name is John and I am 30 years old."
Split the string into individual words
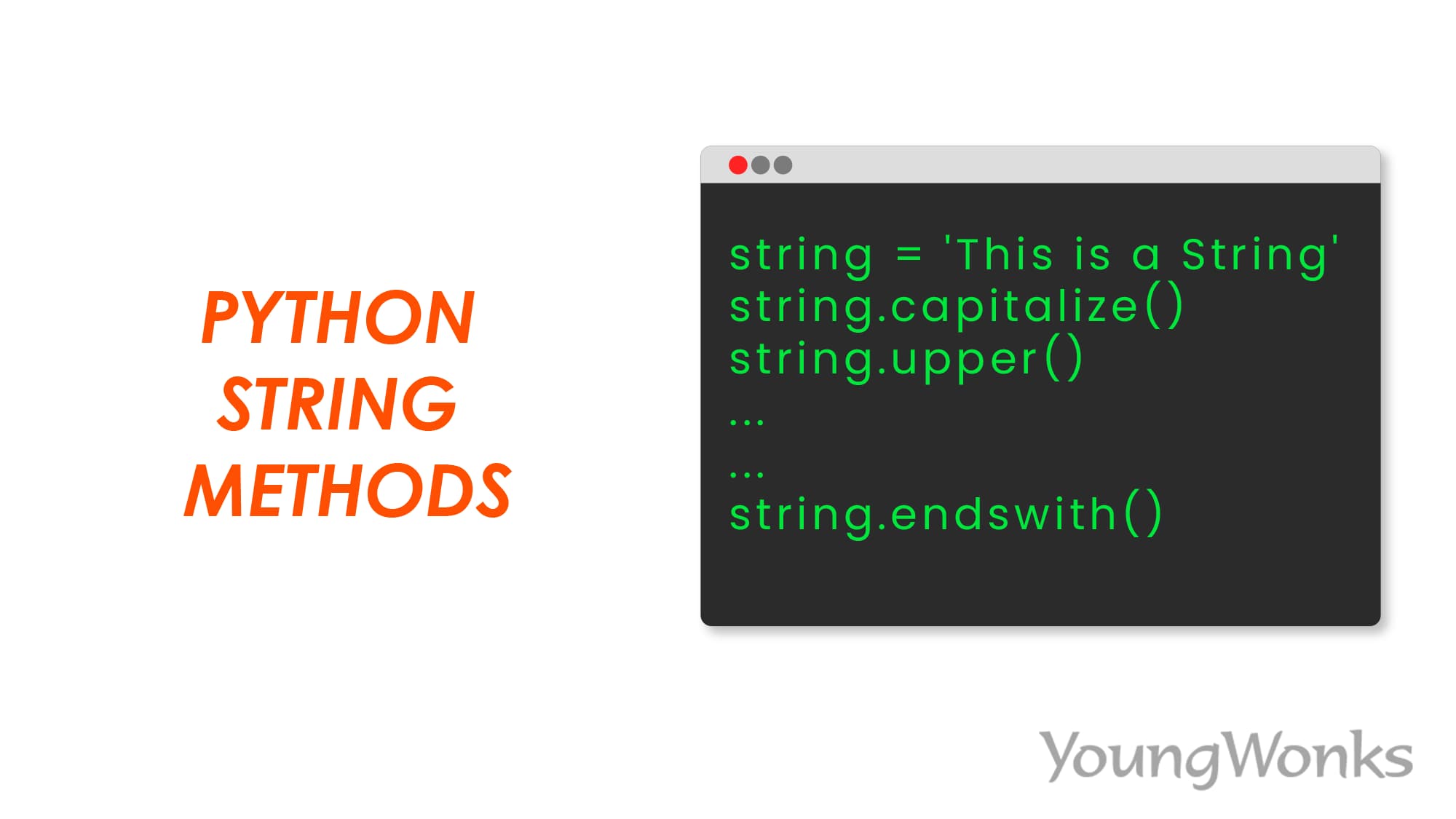
words = original_string.split()
Convert the first word (hello) to uppercase
first_word_uppercase = words[0].upper()
print("The original string is:", original_string)
print("First word in uppercase is:", first_word_uppercase)
output:
The original string is: Hello, my name is John and I am 30 years old.
First word in uppercase is: HELLO
Extract the last three words
last_three_words = " ".join(words[-3:])
print("Last three words are:", last_three_words)
In this example:
We define a string with some sample text. Thesplit()
method is used to split the string into individual words, which are stored in the words
list. We convert the first word (Hello) to uppercase using the upper()
method and store it in the first_word_uppercase
variable. We print the original string and the modified first word. To extract the last three words from the original string, we use negative indexing (words[-3:]
) and join them with spaces using the join()
function. This is done to maintain readability of the output string.
In the Python code above:
Thesplit()
method splits the input string into a list of individual words based on whitespace characters (spaces). We store these individual words in the words
list. We use indexing to access and modify specific parts of the strings. In this example, we convert the first word ("Hello") to uppercase by accessing its first element (words[0]
) and calling the upper()
method on it. The last three words are accessed using negative indexing (words[-3:]
). Negative indices count from the end of the list; in our case, -1
would refer to the last element, -2
the second-to-last, and so forth.
String manipulation is crucial in various applications such as data processing, file handling, and natural language processing. This example illustrates some fundamental operations and techniques for working with strings in Python, allowing you to build on your understanding of programming concepts and expand your skillset.
What are the 10 string operations in Python?
I'd be happy to provide the list of string operations in Python!
1. Concatenation (+)
Concatenation is the process of combining two or more strings into a single string. You can use the +
operator to concatenate strings:
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result) # Output: Hello World
2. Repetition (*)
The *
operator is used to repeat a string a specified number of times:
str1 = "Hi"
num_times = 3
result = str1 * num_times
print(result) # Output: HiHiHi
3. Slicing ([:])
Slicing allows you to extract a portion of a string:
str1 = "Hello World"
start_index = 0
end_index = 5
result = str1[start_index:end_index]
print(result) # Output: Hello
4. Indexing ([:])
Indexing is used to access a character at a specific position in the string:
str1 = "Hello World"
index = 6
char_at_index = str1[index]
print(char_at_index) # Output: W
5. Split ()
The split()
function separates a string into multiple parts based on a specified separator:
str1 = "apple,banana,cherry"
sep = ","
result = str1.split(sep)
print(result) # Output: ['apple', 'banana', 'cherry']
6. Join ()
The join()
function combines multiple strings into a single string:
list_of_strs = ["apple", "banana", "cherry"]
sep = ","
result = sep.join(list_of_strs)
print(result) # Output: apple,banana,cherry
7. Upper () and Lower ()
The upper()
function converts all characters in a string to uppercase:
str1 = "Hello World"
result = str1.upper()
print(result) # Output: HELLO WORLD
The lower()
function converts all characters in a string to lowercase:
8. Strip () and Lstrip/Rstrip ()
The strip()
function removes leading and trailing whitespace from a string:
str1 = " Hello World "
result = str1.strip()
print(result) # Output: Hello World
9. Replace ()
The replace()
function replaces all occurrences of a specified substring in a string with another substring:
str1 = "Hello World"
old_substring = "World"
new_substring = "Universe"
result = str1.replace(old_substring, new_substring)
print(result) # Output: Hello Universe
10. Format ()
The format()
function formats a string by inserting values into placeholders:
str1 = "My name is {} and I am {} years old."
name = "John"
age = 30
result = str1.format(name, age)
print(result) # Output: My name is John and I am 30 years old.
That's the list of string operations in Python! These operations can be used to manipulate strings in a variety of ways, making them essential for working with text data.