How to call a file in Python?
How to call a file in Python?
I'd be happy to help you with that!
In Python, calling a file refers to the process of opening and reading or writing data from a file. There are several ways to call a file in Python, depending on your specific needs and requirements.
Using theopen()
function: The most common way to open a file is by using the built-in open()
function. This function returns a file object, which you can use to read or write from the file. Here's an example:
file = open('example.txt', 'r')
In this example, we are opening a file named example.txt
and reading it ('r'
is for read mode). The file object is stored in the variable file
. You can use methods like read()
, write()
, or close()
on this file object to perform various operations.
with
statement: Python's with
statement provides a way to ensure that the file is properly closed after it is no longer needed. This is achieved by using a context manager, which automatically takes care of closing the file when you are done with it:
with open('example.txt', 'r') as file:
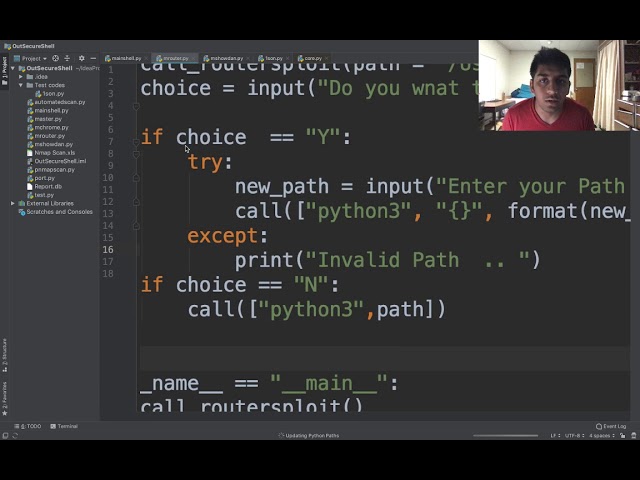
content = file.read()
In this example, we use the with
statement to ensure that the file is properly closed after reading its contents.
os
module: The os
module provides functions for working with operating system-specific details and functionality. One of these functions is os.open()
, which can be used to open a file:
file_descriptor = os.open('example.txt', os.O_RDONLY)
Note that this method returns a file descriptor, rather than a file object.
Using thepathlib
module: The pathlib
module provides classes for working with files and directories in a more Pythonic way:
with open(Path('example.txt').open('r')) as file:
content = file.read()
This method is similar to using the with
statement, but it uses the Path
class from the pathlib
module.
aiofiles
library provides asynchronous file I/O operations:
import aiofiles
async with aiofiles.open('example.txt', 'r') as file:
content = await file.read()
In conclusion, calling a file in Python can be done using various methods and modules. The choice of method depends on the specific requirements and needs of your program.
How to interact with files in python example
I'll respond in English as per your request. Here's a comprehensive overview of how to interact with files in Python, along with examples.
Reading Files
To read the contents of a file in Python, you can use the open
function in read mode ('r'
) or binary read mode ('rb'
). The open
function returns a file object, which has various methods for reading and manipulating the file's content.
Here's an example:
with open('example.txt', 'r') as f:
content = f.read()
print(content)
In this example, we're opening a file named example.txt
in read mode ('r'
) using the open
function. The with
statement ensures that the file is properly closed after we're done reading it.
Writing Files
To write to a file in Python, you can use the open
function in write mode ('w'
) or binary write mode ('wb'
). Be careful when using write mode, as it will overwrite any existing content in the file!
Here's an example:
with open('example.txt', 'w') as f:
f.write('Hello World!')
In this example, we're opening a file named example.txt
in write mode ('w'
) using the open
function. We then write the string 'Hello World!'
to the file.
Appending Files
To append content to a file in Python, you can use the open
function in append mode ('a'
) or binary append mode ('ab'
). This is useful when you want to add new content to an existing file without overwriting its existing content.
Here's an example:
with open('example.txt', 'a') as f:
f.write('This is appended text.')
In this example, we're opening a file named example.txt
in append mode ('a'
) using the open
function. We then write the string 'This is appended text.'
to the end of the file.
Working with Binary Files
Python's open
function can also handle binary files, such as images or audio files. When working with binary files, you typically don't need to worry about character encodings or line endings.
Here's an example:
with open('image.jpg', 'rb') as f:
image_data = f.read()
print(image_data)
In this example, we're opening a binary file named image.jpg
in binary read mode ('rb'
) using the open
function. We then read and store its contents in the image_data
variable.
Working with Text Files (Encoded)
When working with text files encoded with special characters, you might need to specify the encoding when opening the file.
Here's an example:
with open('utf8_file.txt', 'r', encoding='utf-8') as f:
content = f.read()
print(content)
In this example, we're opening a text file named utf8_file.txt
in read mode ('r'
) with UTF-8 encoding using the open
function.
Error Handling
When working with files, it's essential to handle potential errors and exceptions. Python provides various exception types, such as FileNotFoundError
, IOError
, or UnicodeDecodeError
.
Here's an example:
try:
with open('non_existent_file.txt', 'r') as f:
content = f.read()
except FileNotFoundError:
print("The file does not exist.")
In this example, we're trying to open a non-existent file named non_existent_file.txt
in read mode ('r'
) using the open
function. If an error occurs (in this case, a FileNotFoundError
), we catch it and print a custom message.
These examples demonstrate various ways to interact with files in Python. Remember to always handle potential errors and exceptions when working with files!