Python write w3schools
Python write w3schools
Here's a detailed description of W3Schools in Python:
W3Schools Introduction
W3Schools is an online platform that provides tutorials, reference materials, and examples on web development, specifically focusing on HTML, CSS, JavaScript, PHP, SQL, and other related technologies. The platform aims to help developers and beginners learn the skills they need to create interactive and engaging websites.
Key Features of W3Schools
Tutorials: W3Schools offers step-by-step tutorials that cover various topics in web development. These tutorials include examples, syntax explanations, and practice exercises to help users gain hands-on experience. Reference Materials: The platform provides detailed reference materials for HTML, CSS, JavaScript, PHP, SQL, and other related technologies. These resources can be used as a quick guide or a refresher on specific topics. Examples: W3Schools offers numerous examples of code snippets, which demonstrate how to apply programming concepts in real-world scenarios. This helps users understand the syntax and usage of different coding languages. Practice Exercises: The platform includes practice exercises that allow users to test their skills by solving problems or completing tasks.How W3Schools Works
Here's a step-by-step guide on how W3Schools works:
Choose a Topic: Users can select a topic from the various categories, such as HTML, CSS, JavaScript, PHP, and more. Read Tutorials: After choosing a topic, users can access relevant tutorials that cover the selected subject matter. Explore Reference Materials: W3Schools provides detailed reference materials for each topic, including syntax explanations, examples, and practice exercises. Practice with Examples: Users can explore code snippets to gain hands-on experience and apply programming concepts in real-world scenarios.Benefits of W3Schools
Free Resources: W3Schools offers free tutorials, reference materials, and examples for web development, making it accessible to developers worldwide. Easy to Use: The platform is user-friendly, allowing users to quickly navigate through topics and access resources they need. Comprehensive Coverage: W3Schools covers a wide range of topics in web development, providing users with comprehensive knowledge on various technologies.Conclusion
In summary, W3Schools is an online platform that provides valuable resources for web developers and beginners to learn web development skills. With its comprehensive tutorials, reference materials, examples, and practice exercises, W3Schools offers a one-stop-shop for anyone looking to improve their coding abilities.
Python write JSON to file
I'm happy to respond in English!
Python is a great language for working with JSON files. Here's how you can use the json
module to write JSON data to a file:
Example:
import json
Define some sample JSON data as a Python dictionary
data = {
"name": "John Doe",
"age": 30,
"interests": ["reading", "coding", "traveling"],
"address": {
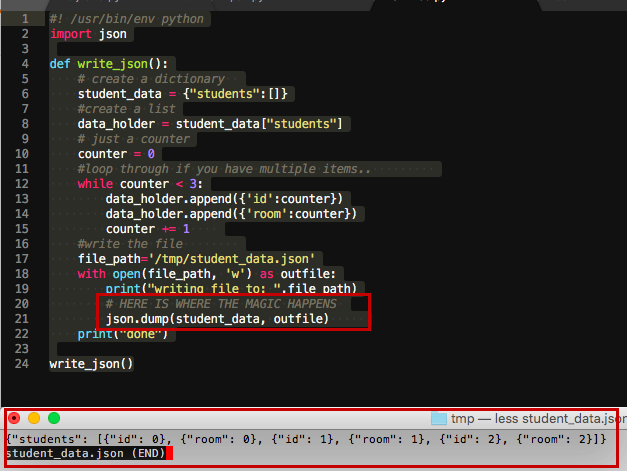
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": 12345
}
}
Convert the Python dictionary to JSON format
json_data = json.dumps(data)
Write the JSON data to a file
with open("data.json", "w") as f:
f.write(json_data)
In this example, we first import the json
module. Then, we define some sample JSON data as a Python dictionary. This dictionary represents a person with name, age, interests, and an address.
Next, we use the dumps()
method to convert our Python dictionary to JSON format. The resulting string is what we want to write to our file.
We open the file "data.json" in write mode ("w"
), and then use the write()
method to write the JSON data to the file.
How it works:
When we run this script, it will create a new file called "data.json" and write the following JSON data to it:
{"name":"John Doe","age":30,"interests":["reading","coding","traveling"],"address":{"street":"123 Main St","city":"Anytown","state":"CA","zip":12345}}
This is a valid JSON object, which we can easily read and parse using the json
module or any other JSON parser.
Advantages:
Writing JSON data to file allows us to persist our data in a format that's easy to work with. Thejson
module provides a simple way to convert Python dictionaries to JSON strings, making it easy to serialize complex data structures. By writing JSON data to a file, we can easily share or transfer our data between different languages, platforms, and systems.
I hope this helps!