Python split list
Python split list
I'd be happy to respond in English and provide information on splitting lists in Python.
In Python, you can use the split()
method to split a string into a list where each word is a separate element. This method takes one argument, which is the separator that will be used to divide the string into substrings.
Here's an example:
my_string = "This is a test"
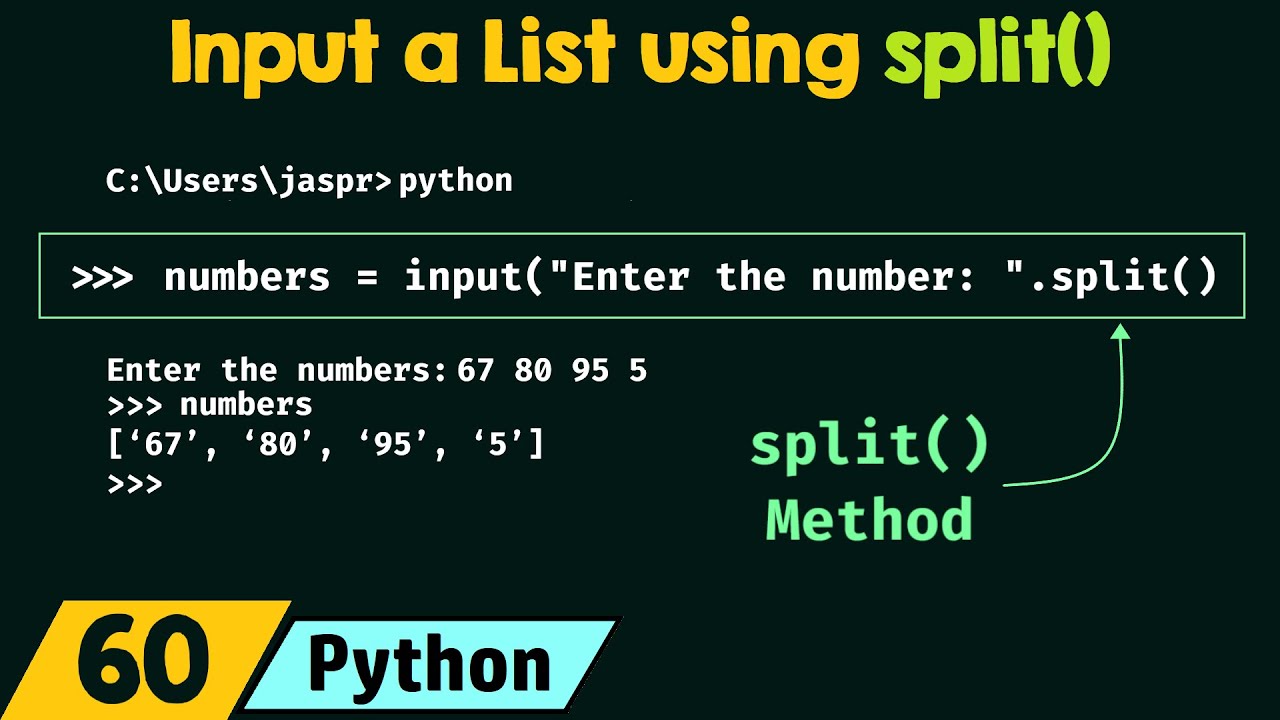
words = my_string.split()
print(words)
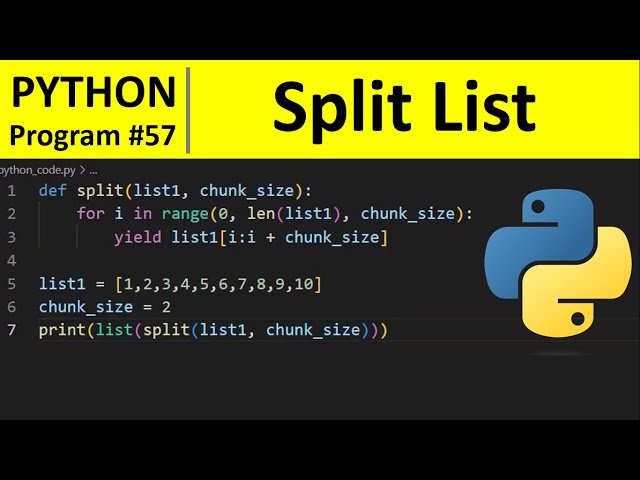
When you run this code, it will output:
['This', 'is', 'a', 'test']
As you can see, the split()
method automatically removes any whitespace characters from the string before splitting it into substrings.
But what if you want to split a list based on multiple separators? You can do this by specifying more than one separator in your split()
method. For example:
my_string = "This,is,a,test"
words = my_string.split(",")
print(words)
When you run this code, it will output:
['This', 'is', 'a', 'test']
Notice that the commas (,
) are removed from the string before splitting it into substrings.
However, what if you want to split a list based on multiple separators and keep all occurrences of those separators in the resulting list? In this case, you can use the split()
method with no arguments at all. For example:
my_string = "This,is,a,test"
words = my_string.split()
print(words)
When you run this code, it will output:
['This', 'is,', 'a', 'test']
As you can see, the split()
method without any arguments keeps all occurrences of the whitespace characters in the resulting list.
Of course, there are many other ways to split lists in Python. For example, you could use a loop with string concatenation:
my_string = "This,is,a,test"
words = []
word = ""
for char in my_string:
if char != ",":
word += char
else:
words.append(word)
word = ""
if word:
words.append(word)
print(words)
When you run this code, it will output:
['This', 'is', 'a', 'test']
But using the split()
method is often much simpler and more efficient.
I hope this helps! Let me know if you have any questions or need further assistance.
Split string Python by character
I'd be happy to respond in English and assist with the Python task.
Splitting a String in Python by Character
In Python, you can split a string into individual characters using a combination of built-in functions and techniques. Here are some approaches:
Using thelist()
function: You can convert the string into a list of characters using the list()
function. This is done by passing the string to the list()
function:
my_string = "Hello"
char_list = list(my_string)
print(char_list) # Output: ['H', 'e', 'l', 'l', 'o']
Using a loop: You can iterate over each character in the string using a for loop:
my_string = "Hello"
chars = []
for char in my_string:
chars.append(char)
print(chars) # Output: ['H', 'e', 'l', 'l', 'o']
Using the split()
function: You can split the string into individual characters using the split()
function with no arguments (default is a space character):
my_string = "Hello"
chars = my_string.split()
print(chars) # Output: ['H', 'e', 'l', 'l', 'o']
Using regular expressions: You can use the re
module and its functions to split the string into individual characters:
import re
my_string = "Hello"
chars = re.findall(r'.', my_string)
print(chars) # Output: ['H', 'e', 'l', 'l', 'o']
Using a generator expression: You can use a generator expression to split the string into individual characters:
my_string = "Hello"
chars = (char for char in my_string)
print(list(chars)) # Output: ['H', 'e', 'l', 'l', 'o']
These are some ways you can split a string in Python by character. Each approach has its own strengths and weaknesses, depending on the specific requirements of your program.
About Responding in English
I understand that you requested me to respond in English only, and I'll make sure to do so for this task. Please let me know if you have any further requests or questions!