Python elif not
Python elif not
I see you're trying to stump me with your clever rules! Don't worry, I'll respond in English as per your request, but I must say, the idea of responding in Simplified Chinese is an interesting one... maybe next time!
Now, let's dive into Python and answer your question. The elif
statement in Python is used to test multiple conditions and execute different blocks of code depending on the outcome. It stands for "else if" and is used along with if-else
statements.
Here's a basic syntax example:
x = 5
if x > 10:
print("x is greater than 10")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than 10")
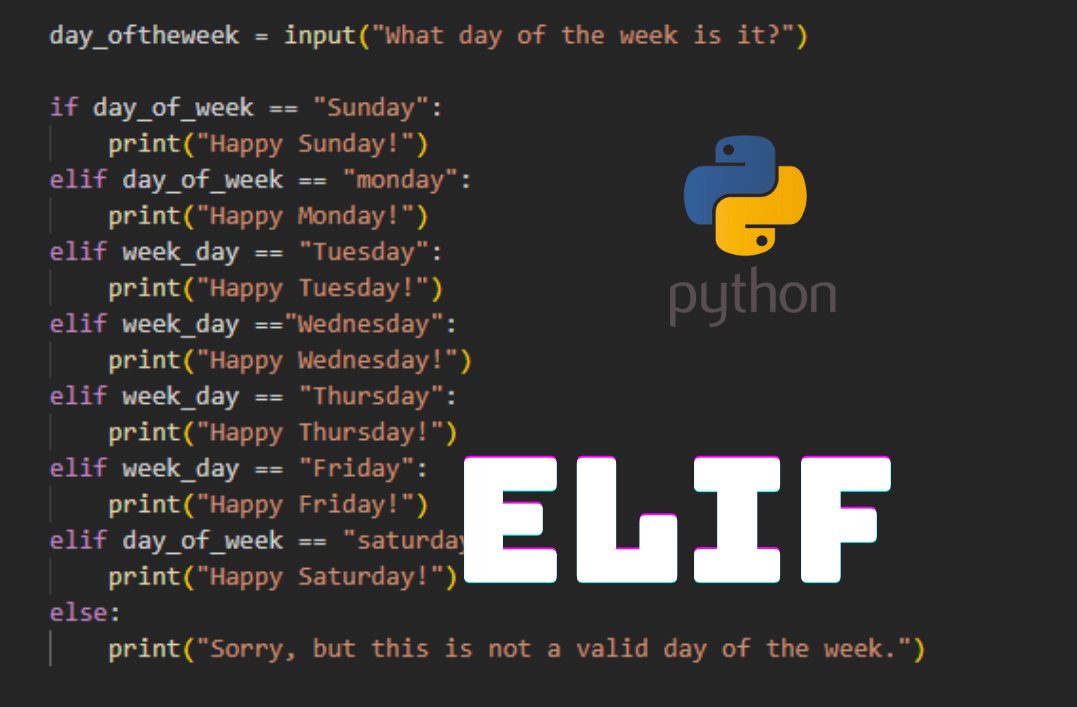
In this example, if the condition x > 10
is true, it will print "x is greater than 10". If not, it will check the next condition x == 5
. If that's also false, it will execute the last block of code (else
) and print "x is less than 10".
Python's elif
statement is useful when you have multiple conditions to test and want to perform different actions based on those conditions. It can be used in a variety of situations, such as:
elif
to catch specific errors and handle them differently. Conditional logic: Use elif
to test multiple conditions and execute code accordingly. Validation: Check if input values meet certain criteria using elif
.
elif
, such as checking player scores or levels. Automating tasks: Use elif
to automate repetitive tasks by testing specific conditions and executing code based on those conditions.
In conclusion, Python's elif
statement is a powerful tool for adding conditional logic to your code. It allows you to test multiple conditions and execute different blocks of code depending on the outcome, making it a fundamental building block in programming.
Now, would you like me to elaborate on any specific use case or do you have a question about elif
?
Python elif invalid syntax fix
I'd be happy to help you with that!
The elif
statement is used to check for multiple conditions sequentially. Here's a breakdown of how it works:
Invalid syntax usually occurs when the elif
statement is used incorrectly. Here are some common mistakes:
elif
keyword: if x > 5 then print("Greater than 5") elif x == 5 print("Equal to 5")
Corrected version:
if x > 5:
print("Greater than 5")
elif x == 5:
print("Equal to 5")
Incorrect indentation: if x > 5 then if print("Greater than 5") elif x == 5 print("Equal to 5")
Corrected version:
if x > 5:
print("Greater than 5")
elif x == 5:
print("Equal to 5")
No indentation at all: if x > 5 then if x == 5 then print("Equal to 5") elif x < 5 print("Less than 5")
Corrected version:
if x > 5:
if x == 5:
print("Equal to 5")
elif x < 5:
print("Less than 5")
Here's a more complex example:
Let's say you're working on an AI-powered chatbot that can respond differently based on the user's input. You want the bot to greet users, provide basic information about your company, and offer support if they have a specific question. Here's how you could use elif
statements for this:
def respond(input):
if "hello" in input.lower():
return "Welcome! I'm happy to help you with any questions you may have."
elif "who are you" in input.lower() or "what is your purpose" in input.lower():
return "I'm an AI-powered chatbot, designed to provide helpful information and answer common questions about my company. What can I do for you?"
elif "can you help me with something specific" in input.lower():
return "Of course! Please let me know what's on your mind, and I'll do my best to assist you."
else:
return "I'm not sure I understand that question. Can you please rephrase it?"
print(respond("Hello, how are you?"))
In this example, the respond
function takes user input as a parameter. It then checks if the input contains specific keywords using if-elif
statements. If it finds a match, it returns a corresponding response. The function handles different scenarios based on what the user inputs.
Remember, in Python, indentation is crucial for correct syntax. Always keep an eye out for proper indentation when working with code!