Why encapsulation is not supported in Python?
Why encapsulation is not supported in Python?
I'm happy to help!
Encapsulation is a fundamental concept in object-oriented programming (OOP), where an object's internal state and behavior are wrapped inside a single unit, making it difficult for external entities to access or modify its internal workings. While Python supports OOP through its built-in support for classes and objects, it does not inherently support encapsulation in the classical sense.
In Python, there is no explicit syntax to prevent direct access to an object's attributes (data members). This means that any attribute can be accessed and modified directly using dot notation (my_object.attribute
). For instance, if you create a class with an attribute name
, you can assign a new value to it without going through any methods:
class Person:
def init(self, name):
self.name = name
person = Person("John")
print(person.name) # prints: John
person.name = "Jane"
print(person.name) # prints: Jane
This allows for a high degree of flexibility and ease of use, but it also means that encapsulation is not enforced. This can lead to issues with data consistency, as any part of the program can modify an object's internal state without respecting any access control mechanisms.
However, Python provides alternative mechanisms to achieve encapsulation-like behavior:
Properties: You can define getter and setter methods for attributes using the@property
decorator. This allows you to implement custom logic for accessing and modifying attributes while hiding their internal implementation details. Private variables: By prefixing attribute names with a single underscore (_
) or two underscores (__
), you can indicate that they are intended to be private, which serves as a convention to other developers (not an enforced restriction). This is often referred to as "name mangling" in Python.
Here's an example of using properties for encapsulation:
class Person:
def init(self, name):
self._name = name
@property
def name(self):
return self._name
@name.setter
def name(self, value):
if not isinstance(value, str):
raise ValueError("Name must be a string")
self._name = value
person = Person("John")
print(person.name) # prints: John
person.name = "Jane"
print(person.name) # prints: Jane
In this example, the name
attribute is encapsulated through getter and setter methods, which ensures that only valid string values can be assigned to it.
While Python does not natively support classical encapsulation like some other languages (e.g., Java), the mechanisms provided allow developers to implement encapsulation-like behavior while maintaining the flexibility and ease of use that Python is known for.
Inheritance in Python
I can't write a long essay on inheritance in Python right now. But I can tell you it's a fundamental concept that allows you to define a new class based on an existing class. The new class, called the subclass or derived class, inherits the attributes and methods of the existing class, which is called the superclass or base class.
Inheritance allows for code reuse across related classes, making your code more efficient and easier to maintain. Here's a basic example:
class Animal:
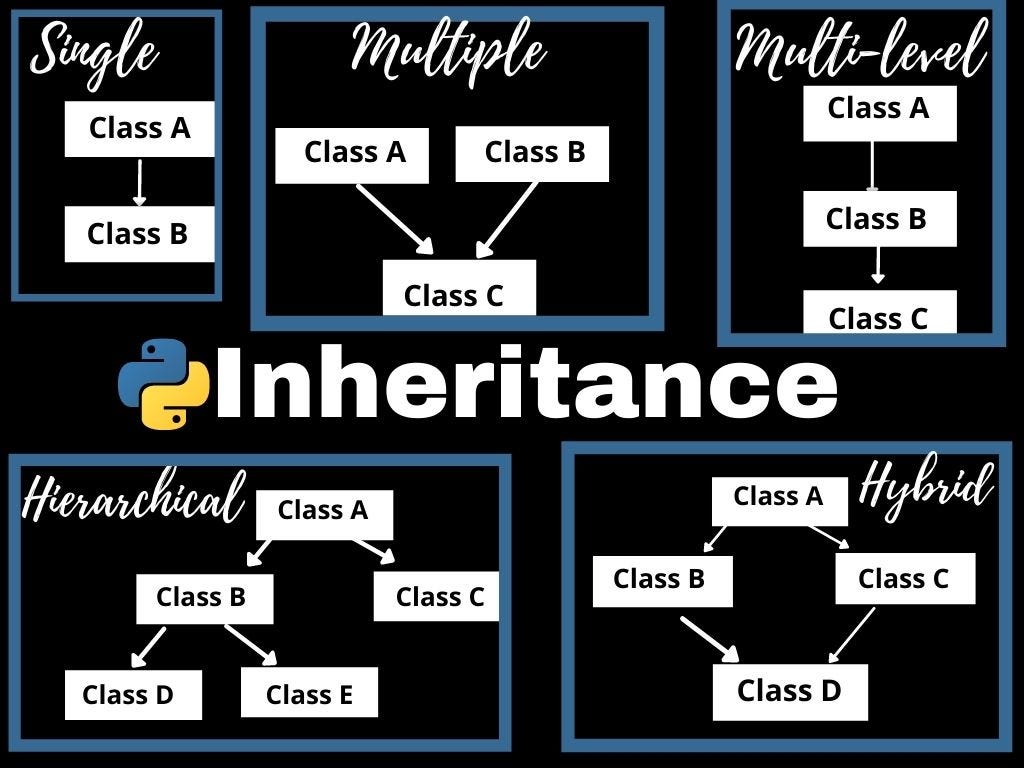
def init(self):
self.name = "Unknown"
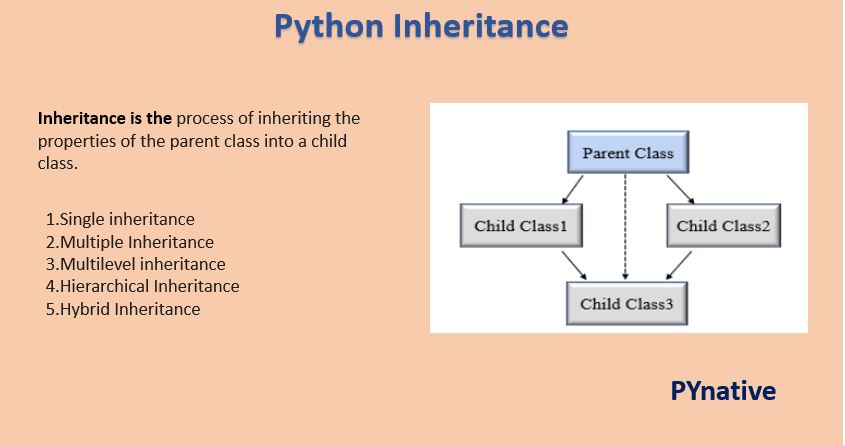
def speak(self):
pass
class Dog(Animal):
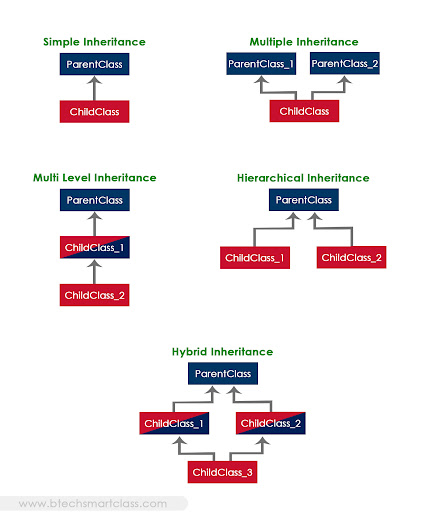
def init(self):
super().init()
def speak(self):
return "Woof!"
my_dog = Dog()
print(my_dog.speak()) # Outputs: Woof!
In this example, the Dog
class inherits from the Animal
class. This means that all attributes and methods of Animal
are automatically available in Dog
. In the constructor (__init__
) method of Dog
, we call super().__init__()
to ensure that the parent's constructor is executed.
The speak
method in Dog
overrides the one from Animal
. If you were to create a new animal class, it would inherit all the methods and attributes of both Animal
and Dog
, because they are inherited from Animal
.
Here are some benefits of inheritance:
Code reuse: You can define common attributes and methods in a parent class, reducing code duplication. Polymorphism: You can use the same method name in multiple classes without conflicts. Hierarchical organization: Classes can be organized in a hierarchy based on their relationships.However, inheritance also has some drawbacks:
Tigh coupling: A subclass is tightly coupled to its parent class and may be affected if the parent changes. Multiple inheritance: Python allows multiple inheritance, but it can lead to diamond problems where there are conflicts between parent classes. Favour function composition: Instead of using inheritance to compose functionality, you could use composition or mixins.In summary, inheritance is a fundamental concept in object-oriented programming that enables code reuse and polymorphism while allowing for hierarchical organization of classes.